Name | Description | Example |
fadeIn( speed, [callback] ) | Achieve the fade-in effect of all matching elements through changes in opacity, and can be used after the animation is completed Optionally trigger a callback function. This animation only adjusts the opacity of the element, which means that the height and width of all matching elements will not change. | Slowly fade the paragraph in for 600 milliseconds:
$("p").fadeIn("slow"); |
##fadeOut( speed, [callback] ) | Achieve the fade-out effect of all matching elements through changes in opacity, and optionally trigger a callback function after the animation is completed. | Slowly fade out the paragraph in 600 milliseconds:
$("p").fadeOut("slow");
|
##fadeTo(speed, opacity, [callback] )Gradually adjusts the opacity of all matching elements to the specified opacity, and optionally triggers a callback function after the animation completes. | Slowly adjust the transparency of the paragraph to 0.66 over 600 milliseconds, about 2/3 visibility:
| $("p").fadeTo("slow", 0.66);$("p").fadeTo("slow", 0.66);
|
Explanation
The fadeIn and fadeOut functions correspond to show and hide, which are used to display and hide objects with a transparency gradient effect:
$("#divPop").fadeIn(200);
$("#divPop").fadeOut("fast");
1. Fade in Fade out
1、jQuery fadeIn() method
jQuery fadeIn() is used to fade in hidden elements.
语法:$(selector).fadeIn(speed,callback);
The optional speed parameter specifies the duration of the effect. It can take the following values: "slow", "fast", or milliseconds. The optional callback parameter is the name of the function to be executed after fading is completed.
The following example demonstrates the fadeIn() method with different parameters:
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeIn();
$("#div2").fadeIn("slow");
$("#div3").fadeIn(3000);
});
});
</script>
</head>
<body>
<p>演示带有不同参数的 fadeIn() 方法。</p>
<button>点击这里,使三个矩形淡入</button>
<br><br>
<div id="div1" style="width:80px;height:80px;display:none;background-color:red;"></div>
<br>
<div id="div2" style="width:80px;height:80px;display:none;background-color:green;"></div>
<br>
<div id="div3" style="width:80px;height:80px;display:none;background-color:blue;"></div>
</body>
</html>
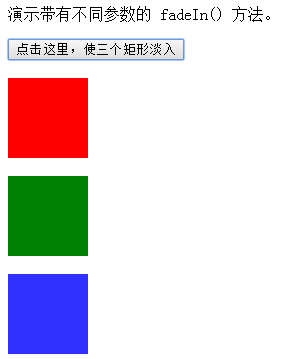
##2. jQuery fadeOut() method
The jQuery fadeOut() method is used to fade out visible elements. 语法:$(selector).fadeOut(speed,callback);
The optional speed parameter specifies the duration of the effect. It can take the following values: "slow", "fast", or milliseconds. The optional callback parameter is the name of the function to be executed after fading is completed. The following example demonstrates the fadeOut() method with different parameters: <!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-3.1.1.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeOut();
$("#div2").fadeOut("slow");
$("#div3").fadeOut(3000);
});
});
</script>
</head>
<body>
<p>演示带有不同参数的 fadeOut() 方法。</p>
<button>点击这里,使三个矩形淡出</button>
<br><br>
<div id="div1" style="width:80px;height:80px;background-color:red;"></div>
<br>
<div id="div2" style="width:80px;height:80px;background-color:green;"></div>
<br>
<div id="div3" style="width:80px;height:80px;background-color:blue;"></div>
</body>
</html>
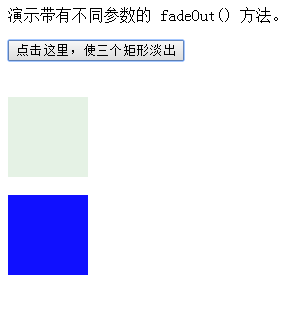
3. jQuery fadeToggle() method
The jQuery fadeToggle() method can switch between the fadeIn() and fadeOut() methods. If the element is already faded out, fadeToggle() adds a fade-in effect to the element. If the element is already faded in, fadeToggle() adds a fade out effect to the element. 语法:$(selector).fadeToggle(speed,callback);
The optional speed parameter specifies the duration of the effect. It can take the following values: "slow", "fast", or milliseconds. The optional callback parameter is the name of the function to be executed after fading is completed. The following example demonstrates the fadeToggle() method with different parameters: <!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeToggle();
$("#div2").fadeToggle("slow");
$("#div3").fadeToggle(3000);
});
});
</script>
</head>
<body>
<p>演示带有不同参数的 fadeToggle() 方法。</p>
<button>点击这里,使三个矩形淡入淡出</button>
<br><br>
<div id="div1" style="width:80px;height:80px;background-color:red;"></div>
<br>
<div id="div2" style="width:80px;height:80px;background-color:green;"></div>
<br>
<div id="div3" style="width:80px;height:80px;background-color:blue;"></div>
</body>
</body>
</html>
4. jQuery fadeTo() method The jQuery fadeTo() method allows gradients to be A certain opacity (value between 0 and 1). 语法:$(selector).fadeTo(speed,opacity,callback);
The required speed parameter specifies the duration of the effect. It can take the following values: "slow", "fast", or milliseconds. The required opacity parameter in the fadeTo() method sets the fade effect to the given opacity (a value between 0 and 1). The optional callback parameter is the name of the function to be executed when the function completes. The following example demonstrates the fadeTo() method with different parameters: <!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeTo("slow",0.15);
$("#div2").fadeTo("slow",0.4);
$("#div3").fadeTo("slow",0.7);
});
});
</script>
</head>
<body>
<p>演示带有不同参数的 fadeTo() 方法。</p>
<button>点击这里,使三个矩形淡出</button>
<br><br>
<div id="div1" style="width:80px;height:80px;background-color:red;"></div>
<br>
<div id="div2" style="width:80px;height:80px;background-color:green;"></div>
<br>
<div id="div3" style="width:80px;height:80px;background-color:blue;"></div>
</body>
</html>
//设置弹出层的透明度
$("#divPop").fadeTo(0, 0.66); //让弹出层透明显示
if ($("#divPop").css("display") == "none")
{
$("#divPop").fadeIn(speed);
} else
{
$("#divPop").fadeOut(speed);
}
After using fadeTo to set the popup layer transparency, using fadeIn will cause the object to display and fade to the fadeTo setting. Transparency.What is introduced here is only the characteristics of the two functions. In actual applications, they do not necessarily need to be used together.
Next Section
<!DOCTYPE html>
<html>
<head>
<script src="http://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").fadeIn();
$("#div2").fadeIn("slow");
$("#div3").fadeIn(3000);
});
});
</script>
</head>
<body>
<p>演示带有不同参数的 fadeIn() 方法。</p>
<button>点击这里,使三个矩形淡入</button>
<br><br>
<div id="div1" style="width:80px;height:80px;display:none;background-color:red;"></div>
<br>
<div id="div2" style="width:80px;height:80px;display:none;background-color:green;"></div>
<br>
<div id="div3" style="width:80px;height:80px;display:none;background-color:blue;"></div>
</body>
</html>