In PHP development, we often need to convert character encodings. ASCII encoding is one of the most common encodings. It is the most basic character encoding and includes common English letters, numbers, punctuation marks and other characters. This article will introduce how to perform ASCII encoding and character conversion in PHP to help developers better understand and apply it.
1. Introduction to ASCII encoding
ASCII encoding (American Standard Code for Information Interchange) is one of the most basic character encodings. It was formulated by the United States and uses 7-bit binary numbers (128 characters in total) ), can represent common characters such as uppercase English letters, lowercase English letters, numbers, punctuation marks, control characters, etc. Each character has a unique binary representation. ASCII encoding is generally used for English character sets and does not include non-English characters, such as Chinese characters.
2. Convert ASCII encoding to characters
In PHP, you can use the chr() function to convert ASCII encoding into corresponding characters. The syntax of the chr() function is as follows:
chr($ascii);
Among them, $ascii is the ASCII encoding value, and the value range is 0-127.
For example, convert the ASCII code 97 into the corresponding characters:
echo chr(97);
The output result is: a
At the same time, you can also use the ord() function to convert the characters into the corresponding ASCII encoded value. The syntax of the ord() function is as follows:
ord($char);
Among them, $char is a character, which can only be one character.
For example, convert character a to the corresponding ASCII code:
echo ord('a');
The output result is: 97
3. Convert string to ASCII code
In In PHP, you can also use the ord() function to convert each character of the string into the corresponding ASCII encoded value and return an ASCII encoded integer array.
$str = "Hello World"; $ascii_arr = array(); for($i=0; $i<strlen print_r><p>Output result: </p> <pre class="brush:php;toolbar:false">Array ( [0] => 72 [1] => 101 [2] => 108 [3] => 108 [4] => 111 [5] => 32 [6] => 87 [7] => 111 [8] => 114 [9] => 108 [10] => 100 )
4. ASCII encoding to string
In PHP, you can use the chr() function to convert the ASCII encoding array into the corresponding string .
$ascii_arr = array(72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100); $str = ""; foreach($ascii_arr as $ascii){ $str .= chr($ascii); } echo $str;
The output result is: Hello World
5. ASCII encoding conversion
In PHP, you can also use functions such as iconv() function and mb_convert_encoding() function to perform ASCII encoding and other common encodings.
$ascii_str = "Hello World"; // ASCII转GB2312 $gbk_str = iconv("ASCII", "GB2312", $ascii_str); echo $gbk_str; // 输出结果为:Hello World // ASCII转UTF-8 $utf8_str = mb_convert_encoding($ascii_str, "UTF-8", "ASCII"); echo $utf8_str; // 输出结果为:Hello World
The above codes convert ASCII encoded strings into GB2312 encoding and UTF-8 encoding respectively.
6. Summary
This article introduces some methods for converting ASCII encoding and characters in PHP, including ASCII encoding to characters, string to ASCII encoding, ASCII encoding to string and ASCII Coding conversion and other related skills. In the actual development process, through these methods, character encoding issues can be better handled to ensure that the application can correctly handle various character sets and encodings.
The above is the detailed content of How to perform ASCII encoding and character conversion in php. For more information, please follow other related articles on the PHP Chinese website!
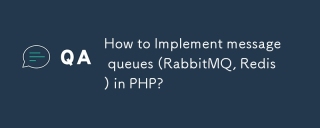
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
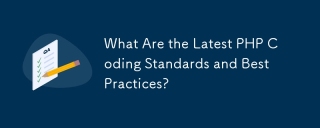
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
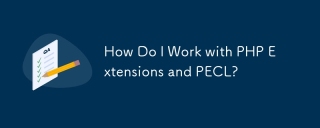
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
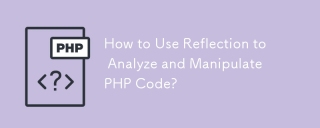
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
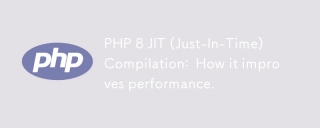
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
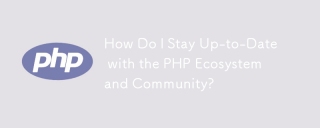
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
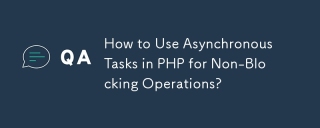
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
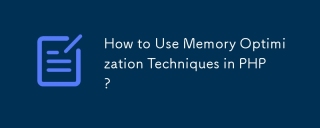
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
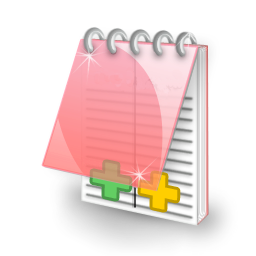
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
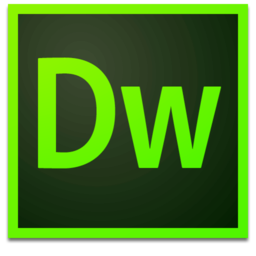
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
