What are the benefits of using password_hash for securing passwords in PHP?
Using password_hash
for securing passwords in PHP offers several key benefits that enhance the security and integrity of password storage. Here are the primary advantages:
-
Automatic Salt Generation:
password_hash
automatically generates a unique salt for each password. This prevents pre-computed attacks like rainbow tables, which can significantly weaken password security. By using a unique salt, even if two users have the same password, the stored hashes will be different. - Use of Strong Hashing Algorithms: The function utilizes modern, secure hashing algorithms such as bcrypt, Argon2i, and Argon2id. These algorithms are designed to be computationally intensive, which makes brute-force attacks more difficult and time-consuming.
-
Adaptive Cost Factor:
password_hash
allows you to specify a cost parameter that controls the computational complexity of the hashing process. This can be adjusted based on the server's capabilities, allowing you to increase security over time as hardware improves. - Ease of Use: The function simplifies the process of hashing passwords securely. It requires minimal configuration and automatically selects appropriate defaults, reducing the chance of implementation errors.
-
Portability and Future-Proofing: The resulting hash includes metadata about the hashing algorithm and cost used, which allows
password_verify
to work correctly even if the algorithm or cost parameters change in the future. -
Secure Defaults:
password_hash
uses secure defaults that align with best practices for password hashing, ensuring that even developers without deep cryptographic knowledge can implement secure password storage.
By leveraging these features, password_hash
provides a robust and straightforward way to secure passwords in PHP applications.
How does password_verify ensure the security of stored passwords in PHP?
password_verify
plays a crucial role in maintaining the security of stored passwords in PHP by securely comparing provided passwords against their hashed counterparts. Here's how it ensures security:
-
Time-Constant Comparison:
password_verify
uses a time-constant string comparison function to prevent timing attacks. Timing attacks occur when an attacker can measure how long it takes to compare a correct and incorrect password, potentially revealing information about the password's structure. The time-constant comparison ensures that the verification time is consistent, regardless of how many characters match. -
Handles Algorithm and Cost Information: The function automatically handles the metadata stored with the hash, such as the hashing algorithm and cost factor used. This means that
password_verify
can correctly verify hashes created with different algorithms or cost parameters, ensuring backwards compatibility and forward security. -
Secure Verification Process:
password_verify
internally decodes the stored hash to extract the salt and the hash value, then re-hashes the provided password using the same salt and parameters. It then compares this new hash to the stored hash. This process is designed to be secure and resistant to common attack vectors. -
Simplicity and Reliability: By encapsulating the complex process of hash verification,
password_verify
reduces the likelihood of implementation errors. Developers do not need to manually extract salts, choose hashing algorithms, or implement the comparison process, which minimizes the chance of introducing vulnerabilities.
By handling these aspects, password_verify
ensures that password verification in PHP is both secure and straightforward, significantly enhancing the overall security posture of applications.
Can password_hash and password_verify be used together to enhance password protection in PHP applications?
Yes, password_hash
and password_verify
are designed to be used together to enhance password protection in PHP applications. Here's how their integration can bolster security:
-
Comprehensive Workflow:
password_hash
is used to securely hash and store passwords when a user creates or updates their password.password_verify
is then used during the login process to verify the provided password against the stored hash. This workflow ensures that passwords are securely stored and verified, covering both the storage and authentication phases of password management. -
Enhanced Security through Salted Hashing: By using
password_hash
, each password is salted and hashed using a strong algorithm.password_verify
then securely compares the provided password with this stored hash, ensuring that the password verification process is secure and resistant to various attack vectors. -
Adaptability and Future-Proofing: As new hashing algorithms or higher cost factors become available,
password_hash
can be used to re-hash existing passwords.password_verify
will seamlessly handle the transition, ensuring that the application remains secure even as security practices evolve. - Best Practices Compliance: Using these functions together aligns with best practices for password security, as recommended by security experts and PHP documentation. This helps developers implement secure password management without deep knowledge of cryptographic principles.
- User Experience and Security Balance: The combination allows developers to implement strong password security without adversely affecting the user experience. The underlying complexity is handled by these functions, allowing for seamless integration into applications.
Here is an example of how they can be used together in a PHP application:
// When a user creates or updates their password $password = 'userPassword123'; $hashed_password = password_hash($password, PASSWORD_DEFAULT); // Store $hashed_password in your database // When a user logs in $user_input = 'userPassword123'; $stored_hash = 'fetch the stored hash from the database'; if (password_verify($user_input, $stored_hash)) { // Password is valid, proceed with login } else { // Password is invalid, handle error }
By leveraging password_hash
and password_verify
together, PHP developers can significantly enhance the security of password management in their applications, ensuring robust protection against common security threats.
The above is the detailed content of PHP Password Hashing: password_hash and password_verify.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
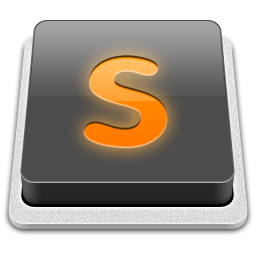
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
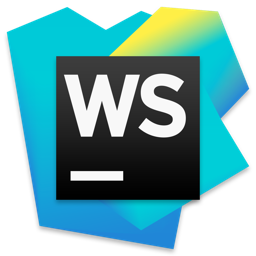
WebStorm Mac version
Useful JavaScript development tools
