PHP XSS Prevention: How to protect against XSS
Cross-Site Scripting (XSS) is a prevalent security vulnerability in web applications where an attacker injects malicious scripts into otherwise benign and trusted websites. To protect against XSS in PHP, it's essential to follow a multi-layered approach:
-
Input Validation and Sanitization: Ensure all user inputs are validated and sanitized to strip out any potential malicious code. PHP provides functions like
htmlspecialchars()
andfilter_var()
to help sanitize input. -
Output Encoding: Always encode data that will be output back to the user. This prevents injected scripts from being executed. PHP functions like
htmlspecialchars()
are useful for this purpose. - Content Security Policy (CSP): Implement a CSP to define which sources of content are allowed to be executed within a web page. This can be set via HTTP headers or meta tags.
-
Use of Security Headers: Implement security headers like
X-XSS-Protection
to enable the browser's built-in XSS filtering. - Regular Security Audits: Conduct regular security audits and penetration testing to identify and fix potential XSS vulnerabilities.
- Education and Awareness: Ensure developers are aware of XSS risks and follow secure coding practices.
By implementing these measures, you can significantly reduce the risk of XSS attacks in your PHP applications.
What are the best practices for sanitizing user input in PHP to prevent XSS attacks?
Sanitizing user input is crucial for preventing XSS attacks. Here are the best practices for sanitizing user input in PHP:
-
Use
htmlspecialchars()
: This function converts special characters to their HTML entities, preventing them from being interpreted as code. For example:$sanitized_input = htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8');
The
ENT_QUOTES
flag ensures that both single and double quotes are encoded, and specifying the charset helps prevent encoding issues. -
Utilize
filter_var()
: PHP'sfilter_var()
function can be used to sanitize and validate input. For example, to sanitize a string:$sanitized_input = filter_var($user_input, FILTER_SANITIZE_STRING);
-
Context-Specific Sanitization: Depending on where the input will be used, apply context-specific sanitization. For example, if the input will be used in a URL, use
urlencode()
:$sanitized_url = urlencode($user_input);
-
Avoid Using
strip_tags()
Alone: Whilestrip_tags()
can remove HTML tags, it's not sufficient for XSS prevention as it doesn't handle attributes or JavaScript events. Use it in conjunction with other sanitization methods. -
Validate Before Sanitizing: Always validate input against expected formats before sanitizing. This helps catch malformed input early. For example:
if (filter_var($user_input, FILTER_VALIDATE_EMAIL)) { $sanitized_email = htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8'); }
By following these practices, you can effectively sanitize user input and reduce the risk of XSS attacks.
How can I implement output encoding in PHP to safeguard against XSS vulnerabilities?
Output encoding is a critical step in preventing XSS vulnerabilities. Here's how you can implement output encoding in PHP:
-
Use
htmlspecialchars()
for HTML Contexts: When outputting data to HTML, usehtmlspecialchars()
to encode special characters. For example:echo htmlspecialchars($data, ENT_QUOTES, 'UTF-8');
This ensures that any HTML special characters in the data are converted to their corresponding HTML entities, preventing them from being interpreted as code.
-
Use
json_encode()
for JSON Contexts: When outputting data as JSON, usejson_encode()
with theJSON_HEX_TAG
option to encode HTML tags:$json_data = json_encode($data, JSON_HEX_TAG | JSON_HEX_APOS | JSON_HEX_QUOT | JSON_HEX_AMP); echo $json_data;
This prevents any HTML tags in the data from being interpreted as code when the JSON is parsed.
-
Use
urlencode()
for URL Contexts: When outputting data as part of a URL, useurlencode()
to encode special characters:$encoded_url = urlencode($data); echo "<a href='example.com?param=" . $encoded_url . "'>Link</a>";
This ensures that any special characters in the data are properly encoded for use in URLs.
-
Context-Specific Encoding: Always consider the context in which the data will be used and apply the appropriate encoding method. For example, if data will be used in a JavaScript context, use
json_encode()
or a similar method to ensure proper encoding.
By consistently applying output encoding based on the context, you can effectively safeguard your PHP applications against XSS vulnerabilities.
What PHP libraries or frameworks can help in automatically preventing XSS attacks?
Several PHP libraries and frameworks can help in automatically preventing XSS attacks by providing built-in security features and functions. Here are some notable ones:
-
HTML Purifier: HTML Purifier is a standards-compliant HTML filter library written in PHP. It can be used to sanitize HTML input and prevent XSS attacks. It's particularly useful for cleaning user-generated content that may contain HTML tags.
require_once 'HTMLPurifier.auto.php'; $config = HTMLPurifier_Config::createDefault(); $purifier = new HTMLPurifier($config); $clean_html = $purifier->purify($dirty_html);
-
OWASP ESAPI for PHP: The OWASP Enterprise Security API (ESAPI) for PHP provides a set of security controls to prevent common security vulnerabilities, including XSS. It includes functions for input validation, output encoding, and more.
use ESAPI\Encoder; $encoder = new Encoder(); $encoded_output = $encoder->encodeForHTML($user_input);
-
Symfony: Symfony is a popular PHP framework that includes built-in security features to help prevent XSS attacks. It provides Twig templating engine, which automatically escapes output by default.
// In a Twig template {{ user_input }} // This will be automatically escaped
-
Laravel: Laravel, another widely-used PHP framework, includes features to help prevent XSS attacks. It uses Blade templating engine, which also automatically escapes output by default.
// In a Blade template {{ $user_input }} // This will be automatically escaped
-
Zend Escaper: Zend Escaper is a component of the Zend Framework that provides context-specific escaping to prevent XSS attacks. It's useful for ensuring that output is properly encoded based on the context.
use Zend\Escaper\Escaper; $escaper = new Escaper('utf-8'); $encoded_output = $escaper->escapeHtml($user_input);
By integrating these libraries and frameworks into your PHP applications, you can leverage their built-in security features to automatically prevent XSS attacks and enhance the overall security of your web applications.
The above is the detailed content of PHP XSS Prevention: How to protect against XSS.. For more information, please follow other related articles on the PHP Chinese website!
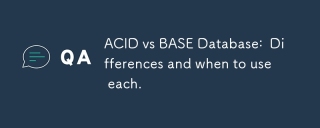
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
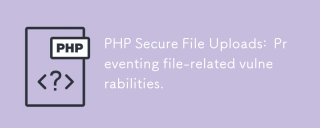
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
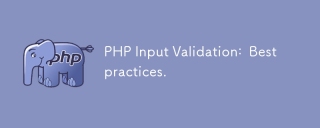
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
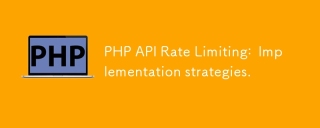
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
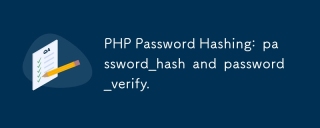
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
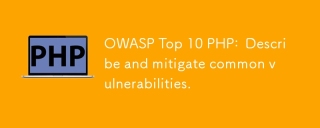
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
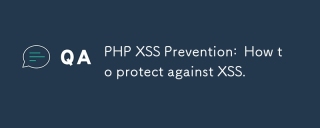
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
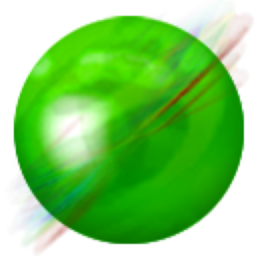
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
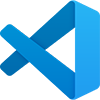
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version