PHP Secure File Uploads: Preventing file-related vulnerabilities
Securing file uploads in PHP is crucial to prevent various vulnerabilities such as code injection, unauthorized access, and data breaches. To ensure the security of your PHP application, it's important to implement robust file upload mechanisms. Let's explore how to enhance security during PHP file uploads by addressing file type validation, secure storage, and proper error handling.
How can I validate file types to enhance security during PHP file uploads?
Validating file types is a critical step in securing file uploads in PHP. By ensuring that only allowed file types are uploaded, you can prevent malicious files from being processed by your application. Here are some methods to validate file types:
-
Check MIME Type:
The MIME type of a file can be checked using the$_FILES['file']['type']
variable. However, this method is not foolproof as MIME types can be easily spoofed. It's a good practice to use it in conjunction with other methods.$allowedMimeTypes = ['image/jpeg', 'image/png', 'application/pdf']; if (in_array($_FILES['file']['type'], $allowedMimeTypes)) { // File type is allowed } else { // File type is not allowed }
-
Check File Extension:
You can check the file extension using thepathinfo()
function. This method is also not entirely secure as file extensions can be manipulated, but it adds an additional layer of security.$allowedExtensions = ['jpg', 'jpeg', 'png', 'pdf']; $fileInfo = pathinfo($_FILES['file']['name']); $extension = strtolower($fileInfo['extension']); if (in_array($extension, $allowedExtensions)) { // File extension is allowed } else { // File extension is not allowed }
-
Use
finfo
to Check File Content:
Thefinfo
function can be used to check the actual content of the file, which is more reliable than checking MIME types or extensions. This method checks the file's magic numbers to determine its type.$finfo = finfo_open(FILEINFO_MIME_TYPE); $mime = finfo_file($finfo, $_FILES['file']['tmp_name']); finfo_close($finfo); $allowedMimeTypes = ['image/jpeg', 'image/png', 'application/pdf']; if (in_array($mime, $allowedMimeTypes)) { // File type is allowed } else { // File type is not allowed }
By combining these methods, you can enhance the security of your file uploads and ensure that only allowed file types are processed by your application.
What are the best practices for securely storing uploaded files in a PHP application?
Storing uploaded files securely is essential to prevent unauthorized access and potential security breaches. Here are some best practices for securely storing uploaded files in a PHP application:
-
Store Files Outside the Web Root:
To prevent direct access to uploaded files, store them outside the web root directory. This ensures that files cannot be accessed via a URL unless explicitly served by your application.$uploadDir = '/path/to/secure/directory/'; $uploadFile = $uploadDir . basename($_FILES['file']['name']); move_uploaded_file($_FILES['file']['tmp_name'], $uploadFile);
-
Use Randomized File Names:
Rename uploaded files to random, unique names to prevent overwriting existing files and to make it harder for attackers to guess file names.$newFileName = uniqid() . '.' . pathinfo($_FILES['file']['name'], PATHINFO_EXTENSION); $uploadFile = $uploadDir . $newFileName; move_uploaded_file($_FILES['file']['tmp_name'], $uploadFile);
-
Implement Access Controls:
Use server-side logic to control access to uploaded files. For example, you can implement user authentication and authorization to ensure that only authorized users can access specific files.if (isUserAuthorized($userId, $fileId)) { // Serve the file } else { // Deny access }
-
Use Secure Protocols for File Transfers:
Ensure that file uploads are performed over HTTPS to encrypt data in transit and prevent man-in-the-middle attacks. -
Regularly Scan for Malicious Files:
Implement a routine to scan uploaded files for malware and other malicious content. Use tools like ClamAV or integrate with a third-party service to perform these scans.
By following these best practices, you can significantly enhance the security of your PHP application's file storage system.
How do I implement proper error handling to prevent information leakage during file uploads in PHP?
Proper error handling is crucial to prevent information leakage and to provide a better user experience. Here are some steps to implement secure error handling during file uploads in PHP:
-
Use Generic Error Messages:
Instead of displaying detailed error messages that could reveal information about your system, use generic error messages that do not disclose sensitive information.if ($_FILES['file']['error'] !== UPLOAD_ERR_OK) { echo "An error occurred while uploading the file. Please try again."; }
-
Log Detailed Errors:
While displaying generic error messages to users, log detailed error information for debugging and monitoring purposes. Ensure that these logs are stored securely and are not accessible to unauthorized users.if ($_FILES['file']['error'] !== UPLOAD_ERR_OK) { error_log("File upload error: " . $_FILES['file']['error']); echo "An error occurred while uploading the file. Please try again."; }
-
Validate and Sanitize Input:
Before processing file uploads, validate and sanitize all input data to prevent injection attacks and other vulnerabilities. Use PHP's built-in functions likefilter_var()
andhtmlspecialchars()
to sanitize input.$fileName = filter_var($_FILES['file']['name'], FILTER_SANITIZE_STRING);
-
Implement Try-Catch Blocks:
Use try-catch blocks to handle exceptions gracefully and prevent the application from crashing. This also helps in logging errors and providing a better user experience.try { // File upload logic if (move_uploaded_file($_FILES['file']['tmp_name'], $uploadFile)) { echo "File uploaded successfully."; } else { throw new Exception("Failed to move uploaded file."); } } catch (Exception $e) { error_log("File upload exception: " . $e->getMessage()); echo "An error occurred while uploading the file. Please try again."; }
By implementing these error handling practices, you can prevent information leakage and ensure that your PHP application remains secure and user-friendly during file uploads.
The above is the detailed content of PHP Secure File Uploads: Preventing file-related vulnerabilities.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
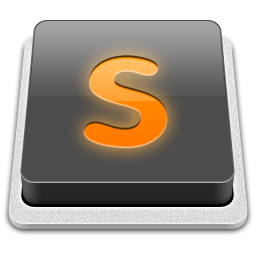
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
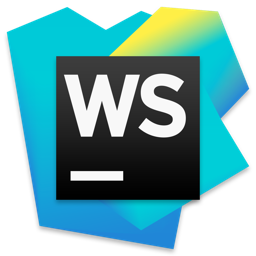
WebStorm Mac version
Useful JavaScript development tools
