PHP Interface vs Abstract Class: When to use each
In PHP, both interfaces and abstract classes serve as blueprints for classes, but they are used in different scenarios due to their unique characteristics.
Interfaces are used to define a contract that classes must adhere to without providing any implementation. They are useful when you want to specify a set of methods that must be implemented by any class that implements the interface, but you don't care about the details of how those methods are implemented. Interfaces are ideal for defining common behaviors across different types of objects.
On the other hand, abstract classes can contain both abstract methods (methods without a body, like those in interfaces) and concrete methods (methods with a body). They are useful when you want to provide a common base of functionality for related classes, with some parts left to be implemented by the subclasses.
When to use each:
- Use an interface when you want to define a contract for behavior without specifying how it should be implemented. This is useful for creating loosely coupled systems where different classes can implement the same interface in different ways.
- Use an abstract class when you want to provide a common base of functionality for a group of related classes, and you want to enforce that certain methods are implemented by the subclasses.
What specific scenarios call for the use of an interface over an abstract class in PHP?
There are several specific scenarios where using an interface is more appropriate than using an abstract class in PHP:
-
Defining a Contract for Multiple Unrelated Classes: If you have multiple classes that need to implement a certain set of methods but are otherwise unrelated, an interface is the better choice. For example, if you want to ensure that different types of objects (e.g., a
Car
and aPlane
) can both be "driven," you can define aDrivable
interface with adrive()
method. - Multiple Inheritance: PHP does not support multiple inheritance of classes, but it does allow a class to implement multiple interfaces. If a class needs to inherit behavior from multiple sources, interfaces can be used to define these behaviors without the limitations of single class inheritance.
-
Decoupling: Interfaces help in creating loosely coupled systems. If you want to ensure that a class can work with different implementations of a certain behavior without knowing the specifics of those implementations, an interface is ideal. For example, a
Logger
interface can be implemented by different logging classes (e.g.,FileLogger
,DatabaseLogger
), allowing a system to log messages without being tied to a specific logging mechanism. - Testing and Mocking: Interfaces make it easier to create mock objects for testing. Since interfaces define only the method signatures, it's straightforward to create mock implementations for unit testing purposes.
How can using an abstract class in PHP improve code organization and reusability?
Using abstract classes in PHP can significantly improve code organization and reusability in several ways:
-
Common Functionality: Abstract classes allow you to define common functionality that can be shared among related classes. For example, if you have a set of classes representing different types of vehicles, an abstract
Vehicle
class can contain methods likestartEngine()
andstopEngine()
that are common to all vehicles. -
Enforcing Structure: By defining abstract methods, an abstract class can enforce that certain methods must be implemented by its subclasses. This helps maintain a consistent structure across related classes. For instance, an abstract
Animal
class might require subclasses to implement aneat()
method. -
Code Reusability: Since abstract classes can contain concrete methods, you can implement common logic once in the abstract class and reuse it across all subclasses. This reduces code duplication and makes maintenance easier. For example, an abstract
PaymentGateway
class might include avalidateCard()
method that is used by all specific payment gateway implementations. - Hierarchical Organization: Abstract classes help in organizing code into a clear hierarchy. They serve as a middle layer between interfaces (which define contracts) and concrete classes (which provide full implementations). This hierarchical structure makes the codebase more understandable and easier to navigate.
In what ways do interfaces in PHP enhance the flexibility of your code design?
Interfaces in PHP enhance the flexibility of code design in several key ways:
-
Polymorphism: Interfaces allow for polymorphism, which means that objects of different classes can be treated as objects of a common type. This allows you to write more flexible and generic code. For example, if you have an
Iterator
interface, you can write code that works with any object that implements this interface, regardless of its specific class. -
Dependency Injection: Interfaces facilitate dependency injection, a design pattern that promotes loose coupling. By programming to an interface rather than a concrete class, you can easily swap out different implementations without changing the dependent code. For instance, if you have a
PaymentProcessor
interface, you can inject different implementations (e.g.,CreditCardProcessor
,PayPalProcessor
) into your system without modifying the code that uses the processor. -
Extensibility: Interfaces make it easier to extend your system. New classes can be added that implement existing interfaces without affecting existing code. This allows for future enhancements and modifications without breaking the existing system. For example, if you have a
Storage
interface, you can add new storage implementations (e.g.,CloudStorage
,LocalStorage
) as needed. - Testability: Interfaces enhance the testability of your code. By defining interfaces, you can create mock objects that implement these interfaces for unit testing. This allows you to test components in isolation, making your tests more reliable and easier to maintain.
- Clear Contracts: Interfaces provide a clear contract for what a class must do, without specifying how it should do it. This clarity helps in designing systems where different parts can be developed independently, as long as they adhere to the defined interfaces. This separation of concerns leads to more flexible and maintainable code.
The above is the detailed content of PHP Interface vs Abstract Class: When to use each.. For more information, please follow other related articles on the PHP Chinese website!
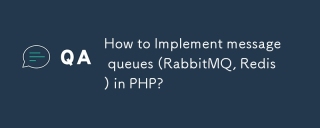
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
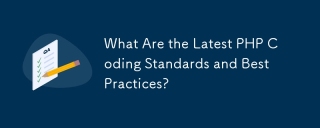
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
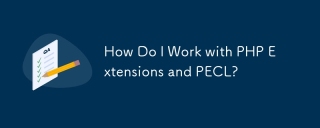
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
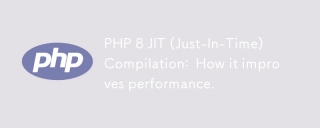
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
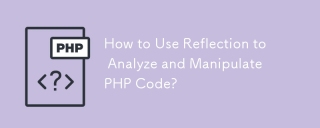
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
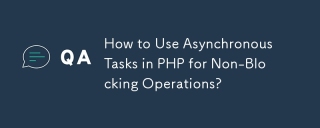
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
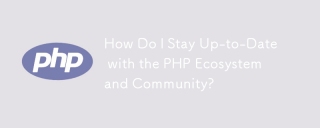
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
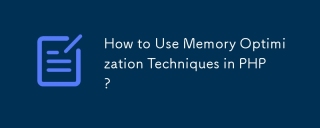
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
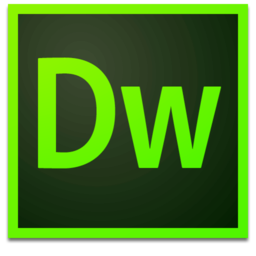
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),