Object-Relational Mapping (ORM) is an essential design pattern in modern software development. The core idea of the ORM pattern is to map objects in the program to tables in the relational database, thereby achieving seamless integration between the program and the database. The implementation of the ORM pattern can utilize existing ORM frameworks, such as Hibernate, Django ORM, and Active Record in Ruby on Rails. However, in PHP, we can use native PHP code to implement the object persistence layer without requiring additional frameworks. This article will introduce how to implement the object persistence layer using native PHP code.
1. Basics of relational database
Before understanding how to use PHP to implement the object persistence layer, we need to first understand some basic concepts in relational databases.
1. Database table
Relational database is composed of many data tables. Each data table consists of one or more columns. Each column has a corresponding data type, such as integer, string, date, etc.
2. Primary key
Each data table needs to have a primary key. The primary key is a field that uniquely identifies each record in the table. The primary key must be unique and cannot be NULL.
3. Foreign keys
Foreign keys are used to establish relationships between two or more tables. Foreign keys define the association between one table and another table, allowing us to obtain information from the two tables and merge them through a joint query.
2. Use PDO to access the database
PDO (PHP Data Objects) is the standard way to access the database in PHP. We can use PDO to access various relational databases (such as MySQL, PostgreSQL, etc.) . Using PDO to operate the database can improve the portability of the program and increase the security of the program.
In PHP, we can use the PDO class to initialize a database connection. Among them, the parameters that the user needs to provide include database type, host name, database name, user name and password, etc. After initialization, we can use the PDO instance to execute SQL queries and process the query results.
The following is an example of using PDO to access the MySQL database:
<?php try { $dbh = new PDO('mysql:host=localhost;dbname=test', $user, $pass); foreach($dbh->query('SELECT * from FOO') as $row) { print_r($row); } $dbh = null; } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br>"; die(); } ?>
Executing the above code will select the FOO table in MySQL and print the query results.
3. Use PHP to implement ORM mode
When implementing the ORM mode, we need to use PHP objects and classes to describe the tables and columns in the database. We need to define a corresponding class for each table and convert the columns in the table into attributes of the class. In order to implement the ORM mode, we also need to introduce a database access layer to operate the database and convert query results into PHP class objects. The following are development steps for using PHP to implement ORM mode:
1. Create a database connection MySQL
In PHP, we can use PDO to connect to the MySQL database. The following is a code example for opening a MySQL database connection:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDBPDO"; try { $conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password); // set the PDO error mode to exception $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connected successfully"; } catch(PDOException $e) { echo "Connection failed: " . $e->getMessage(); } ?>
In the above code, we create a connection to the MySQL database and set the error mode of PDO to exception mode. When using PDO, the exception mode allows us to more conveniently handle errors that may occur in the program.
2. Define ORM classes
In order to implement the ORM mode, we need to define the ORM class corresponding to the table and convert the columns in the table into attributes of the class. The following is a sample code for an ORM class:
<?php class User { private $id; private $name; private $email; private $password; public function __construct($id, $name, $email, $password) { $this->id = $id; $this->name = $name; $this->email = $email; $this->password = $password; } public function getId() { return $this->id; } public function setId($id) { $this->id = $id; } public function getName() { return $this->name; } public function setName($name) { $this->name = $name; } public function getEmail() { return $this->email; } public function setEmail($email) { $this->email = $email; } public function getPassword() { return $this->password; } public function setPassword($password) { $this->password = $password; } } ?>
In the above code, we create a User class and convert the id, name, email, and password columns in the table into attributes of the class. We also define the constructor of the class and a series of getter and setter methods.
3. Implement the ORM data access layer
In order to implement the ORM mode, we also need to implement a data access layer to operate the database. The following is a simple data access layer sample code:
<?php class UserDAO { private $pdo; public function __construct(PDO $pdo) { $this->pdo = $pdo; } public function getUserById($id) { $stmt = $this->pdo->prepare('SELECT * FROM user WHERE id = :id'); $stmt->execute(array('id' => $id)); $data = $stmt->fetch(); if ($data) { return new User($data['id'], $data['name'], $data['email'], $data['password']); }else{ return null; } } public function saveUser(User $user) { if ($user->getId()) { // update $stmt = $this->pdo->prepare('UPDATE user SET name = :name, email = :email, password = :password WHERE id = :id'); $stmt->execute(array('name' => $user->getName(), 'email' => $user->getEmail(), 'password' => $user->getPassword(), 'id' => $user->getId())); } else { // insert $stmt = $this->pdo->prepare('INSERT INTO user (name, email, password) VALUES (:name, :email, :password)'); $stmt->execute(array('name' => $user->getName(), 'email' => $user->getEmail(), 'password' => $user->getPassword())); $user->setId($this->pdo->lastInsertId()); } } public function deleteUser(User $user) { $stmt = $this->pdo->prepare('DELETE FROM user WHERE id = :id'); $stmt->execute(array('id' => $user->getId())); } } ?>
In the above code, we created a UserDAO class, which is used to operate the database and return User objects. The getUserById method in this class obtains user information with a specified id from the database, the saveUser method updates or inserts user information into the database, and the deleteUser method is used to delete user information.
4. Using ORM classes
After implementing the ORM mode, we can use ORM objects like ordinary objects without manually writing any SQL queries. Below is a sample code for an ORM class:
<?php // create a new user $user = new User(null, 'John Doe', 'john@example.com', 'password'); $userDAO = new UserDAO($pdo); $userDAO->saveUser($user); // find a user by id $user = $userDAO->getUserById(1); // update a user's name $user->setName('Jane Doe'); $userDAO->saveUser($user); // delete a user $userDAO->deleteUser($user); ?>
In the above code, we create a new user and insert the user into the database. Then, we use the getUserById method to find the user information with ID 1. We can update that user's name using the setter method and then save the changes to the database using the saveUser method. Finally, we delete the user using the deleteUser method.
Conclusion
In this article, we introduced how to implement the object persistence layer pattern using PHP. First, we learned the basics of relational databases, and then took a deeper look at PHP's use of PDO to connect to the database. By understanding the relational database and PDO connection methods, we can manually implement the ORM mode ourselves. By defining ORM classes and data access layers, we can seamlessly integrate database operations into our PHP code. The use of ORM mode can simplify our code to a great extent and handle database operations more conveniently.
The above is the detailed content of How to implement object persistence layer in php. For more information, please follow other related articles on the PHP Chinese website!
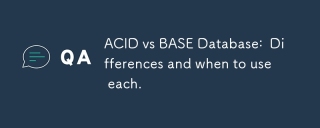
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
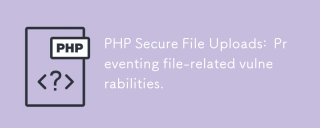
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
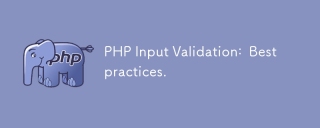
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
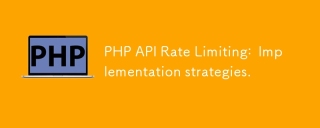
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
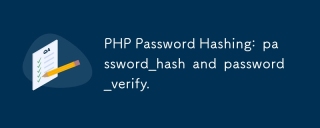
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
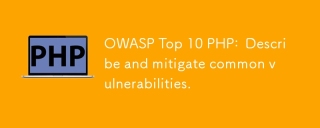
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
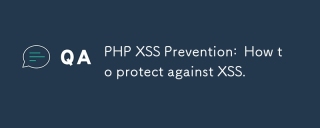
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
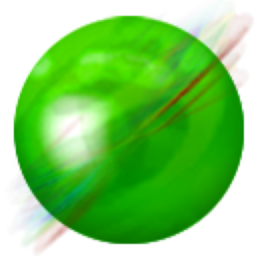
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
