With the development of the Internet, search engines have become one of the important channels for people to obtain information. Many websites are also equipped with their own search functions to help users quickly find the content they need. PHP, as a commonly used programming language, can also implement search recording functions to provide users with a better user experience. This article will introduce how to implement PHP search records.
1. Database design
The first thing to consider is the design of the database. In this article, MySQL is used as an example.
1.1 Table design
You need to create a table to store the records searched by the user, including the following fields:
- id: the unique identifier of the record, automatically incremented
- keyword: keywords searched by the user
- search_time: time of the user’s search, in the format of datetime
- user_id: the user’s unique identifier, can be empty
The structure of the table is as follows:
CREATE TABLE search_history
(
id
int(11) NOT NULL AUTO_INCREMENT,
keyword
varchar(255) NOT NULL DEFAULT '',
search_time
datetime NOT NULL DEFAULT CURRENT_TIMESTAMP,
user_id
int(11) DEFAULT NULL,
PRIMARY KEY (id
)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
1.2 Field description
In the above table, you need to pay attention to the following points:
- id is the primary key, automatically incremented, and used to uniquely identify a record.
- keyword is the keyword searched by the user and is not allowed to be empty.
- search_time is the time when the search occurs, the format is datetime type, and the default value is set to the current time for easy recording.
- user_id is the user's unique identifier, which can facilitate statistics and analysis of user behavior in some scenarios, but it is not a necessary field, so it can be empty.
2. Record search history
On the page, the user's search function can be implemented through a form. According to the search content, it is saved to the search_history table mentioned above. . The specific code is as follows:
2.1 Connect to the database
First you need to connect to the database in order to store the search results in the database.
$conn = mysqli_connect('localhost', 'root', 'password', 'database_name');
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
mysqli_set_charset ($conn,"utf8mb4");
2.2 Processing search requests
Get the content entered by the user in the search box, for example:
$keyword = $_REQUEST[' keyword'];
If the user does not enter anything, there is no need to save it to the database.
if (empty($keyword)) {
return;
}
2.3 Save the search results to the database
Then, save the search results to the above The search_history table to record the user's search history.
$sql = "INSERT INTO search_history (keyword, user_id) VALUES ('$keyword', 1)";
mysqli_query($conn, $sql);
In the above code , save the searched keyword and user's unique identifier (tentatively 1) to the keyword and user_id fields in the search_history table.
3. Display search history
If you need to display the search history on the website, you can obtain it by querying the search_history table in the database. Below we will introduce how to implement this function through PHP.
3.1 Query the database
$conn = mysqli_connect('localhost', 'root', 'password', 'database_name');
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
mysqli_set_charset($conn,"utf8mb4");
$sql = "SELECT * FROM search_history ORDER BY search_time DESC LIMIT 10";
$result = mysqli_query($conn , $sql);
The above code first connects to the database and queries all records in the search_history table, arranges them in reverse chronological order, and obtains the top 10 most recent records.
3.2 Obtain historical records
Next, loop through the obtained records and output them to the page.
if (mysqli_num_rows($result) > 0) {
while($row = mysqli_fetch_assoc($result)) { echo $row["keyword"]; }
} else {
echo "暂无搜索历史记录";
}
The above code first determines whether the query result is Empty. If it is not empty, the keywords of each record will be output to the page through loop traversal. If it is empty, prompt information will be output.
4. Delete search history
If the user needs to delete some search history, he can clear the records that need to be deleted from the database by adding a "Delete" button on the page.
4.1 Connecting to the database
The operation is the same as above and will not be repeated here.
$conn = mysqli_connect('localhost', 'root', 'password', 'database_name');
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
mysqli_set_charset ($conn,"utf8mb4");
4.2 Processing deletion requests
Get the id of the record that the user needs to delete and delete it from the database.
$id = $_REQUEST['id'];
if (!empty($id)) {
$sql = "DELETE FROM search_history WHERE id='$id'"; mysqli_query($conn, $sql);
}
If id is If empty, no action is required.
5. Summary
Through the above introduction, we can find that the implementation of PHP search records mainly needs to revolve around the design of the database. By setting the corresponding fields in the table, the search results and recording time are stored in the database to facilitate query and exhibit. Of course, you need to pay attention to security issues and avoid SQL injection and other attacks. At the same time, if you need to implement the search record function more flexibly, you can also use other technologies, such as cookies, sessions, etc.
The above is the detailed content of How to implement search records in php. For more information, please follow other related articles on the PHP Chinese website!
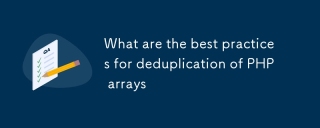
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
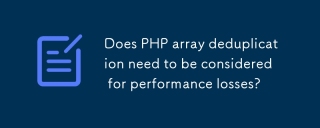
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
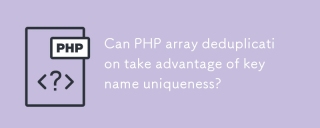
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
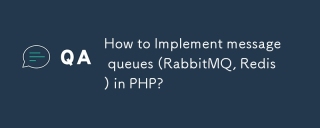
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
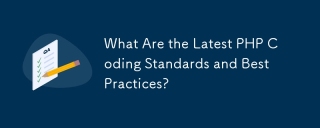
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
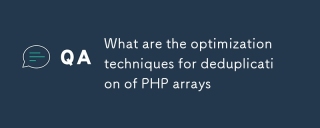
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
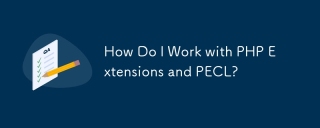
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
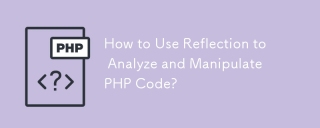
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
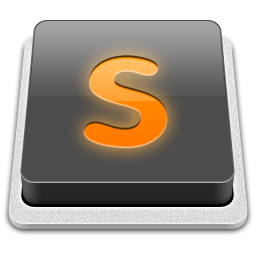
SublimeText3 Mac version
God-level code editing software (SublimeText3)
