(This article is for reference only. The code may have errors due to changes in time and versions. Readers are asked to adjust by themselves)
In website development, the query function is very common. I will share it with you below. Let’s look at the PHP code implementation of a simple query function.
First, create an input box and a submit button in the HTML file:
Then, write the SQL statement in the query.php file and output the result:
<?php // 建立数据库连接 $conn = mysqli_connect("localhost", "username", "password", "database_name"); // 获取输入框中的名字 $name = $_POST['name']; // 查询语句 $sql = "SELECT * FROM user WHERE name LIKE '%$name%'"; // 执行查询 $result = mysqli_query($conn, $sql); // 输出查询结果 if(mysqli_num_rows($result) > 0) { while($row = mysqli_fetch_assoc($result)) { echo "名字:" . $row['name'] . "<br>"; echo "年龄:" . $row['age'] . "<br>"; echo "性别:" . $row['gender'] . "<br>"; echo "<hr>"; } } else { echo "查询结果为空!"; } // 关闭数据库连接 mysqli_close($conn); ?>
Code explanation:
- To establish a database connection, you need to fill in the corresponding database address, user name, password and database name.
- Get the name in the input box through
$_POST
. -
$sql
is the query statement,LIKE
represents fuzzy query, and%
represents any character. - Execute the query and read the query results row by row through
mysqli_fetch_assoc
. - Output the query results and use
while
loop andmysqli_num_rows
to determine whether the query results are empty. - Close the database connection and release resources.
This article only briefly introduces the PHP code implementation of the query function and does not include other security and optimization content. In actual development, you also need to pay attention to preventing security issues such as SQL injection, and optimizing the performance of query statements.
The above is the detailed content of PHP code implements simple query function. For more information, please follow other related articles on the PHP Chinese website!
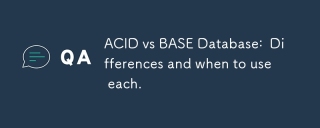
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
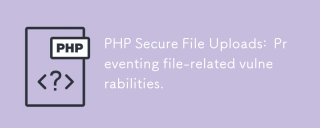
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
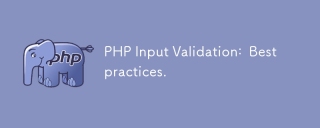
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
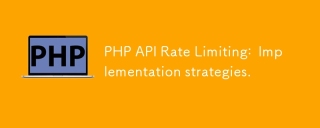
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
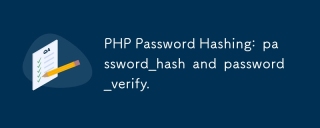
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
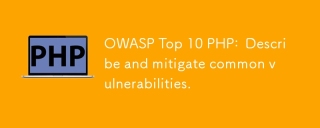
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
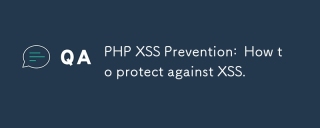
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
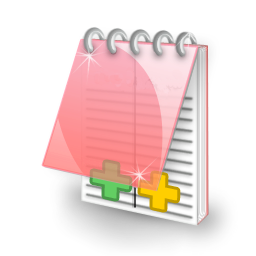
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version
