In PHP, sometimes we need to convert a string into an array to facilitate its operation and processing. Here are some simple ways to achieve this goal.
Method 1: Use the explode() function
Theexplode() function can split a string into an array. It takes two parameters: a delimiter and a string. A delimiter is a character or string contained in a string that specifies the split position.
For example, you can use the following code to split a string into an array:
$str = "apple,orange,banana"; $arr = explode(",", $str); print_r($arr);
The above code will output:
Array ( [0] => apple [1] => orange [2] => banana )
In the above example, we Split a string into an array using comma as delimiter. You can also use other characters or strings as delimiters.
Method 2: Use the str_split() function
The str_split() function can split a string into substrings of equal length and store them in an array. It takes two parameters: a string and an integer, which specifies the length of each substring.
For example, you can use the following code to split a string into substrings of length 3:
$str = "abcdef"; $arr = str_split($str, 3); print_r($arr);
The above code will output:
Array ( [0] => abc [1] => def )
above In the example, we split the string into substrings of length 3 and store them in an array.
Method 3: Use the preg_split() function
The preg_split() function can use regular expressions to split a string into an array. It takes two parameters: a regular expression and a string.
For example, you can use the following code to split a string into an array:
$str = "apple orange banana"; $arr = preg_split("/\s+/", $str); print_r($arr);
The above code will output:
Array ( [0] => apple [1] => orange [2] => banana )
In the above example, we Split string into array using regular expression using space as delimiter. You can use other regular expressions to specify delimiters.
Summary
In PHP, there are many ways to convert a string into an array. The above are three common methods: using the explode() function, using the str_split() function and using the preg_split() function. Which method you choose depends on your specific needs and preferences.
The above is the detailed content of How to convert a string into an array in php (three methods). For more information, please follow other related articles on the PHP Chinese website!
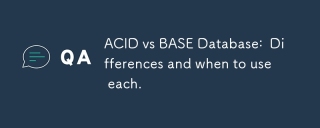
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
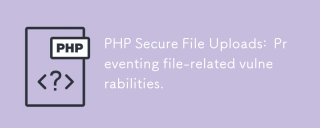
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
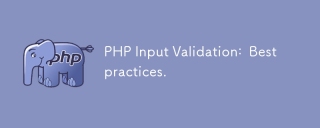
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
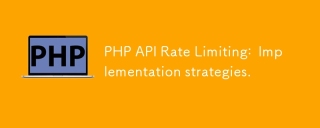
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
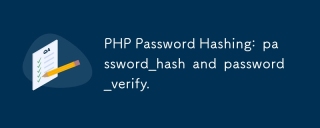
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
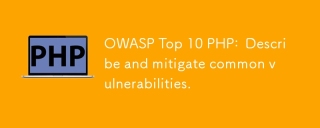
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
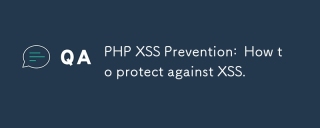
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
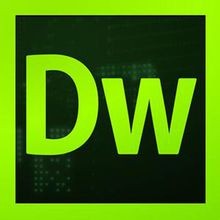
Dreamweaver CS6
Visual web development tools
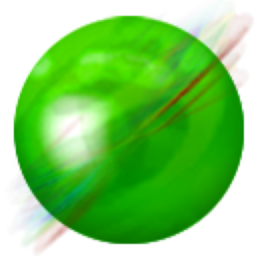
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
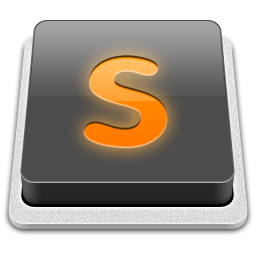
SublimeText3 Mac version
God-level code editing software (SublimeText3)