php method to obtain random numbers without duplication: 1. Use the [rand(min,max)] function to generate random numbers; 2. Use [array_unique(arr)] to deduplicate the generated array; 3. Use indexes to quickly generate unique random numbers.
php method to get random numbers without repetition:
The first thing that comes to mind isrand(min, The max)
function generates random numbers. In fact, using mt_rand(min,max)
can generate random numbers more quickly.
Secondly use array_unique(arr)
to deduplicate the generated array. In fact, using array_flip(array_flip(arr))
can deduplicate it more quickly.
Understanding the above two points, we can write a slightly optimized function:
/** * 生成指定长度不重复的字符串. * * @param integer $min 最小值. * @param integer $max 最大值. * @param integer $len 生成数组长度. * * @return array */ function uniqueRandom($min, $max, $len) { if ($min < 0 || $max < 0 || $len) { throw new LogicException('无效的参数'); } if ($max <= $min) { throw new LogicException('大小传入错误'); } $counter = 0; $result = array(); while ($counter < $len) { $result[] = mt_rand($min, $max); $result = array_flip(array_flip($result)); $counter = count($result); } shuffle($result); return $result; }
In fact, you can use the index to generate non-repeating random numbers more quickly, and the efficiency is several times better than the above function. street.
/** * 生成指定长度不重复的字符串. * * @param integer $min 最小值. * @param integer $max 最大值. * @param integer $len 生成数组长度. * * @return array */ function uniqueRandom2($min, $max, $len) { if ($min < 0 || $max < 0 || $len < 0) { throw new LogicException('无效的参数'); } if ($max <= $min) { throw new LogicException('大小传入错误'); } if (($max - $min + 2) < $len) { throw new LogicException("传入的范围不足以生成{$len}个不重复的随机数}"); } $index = array(); for ($i = $min; $i < $max + 1; $i++) { $index[$i] = $i; } $startOne = current($index); $endOne = end($index); for ($i = $startOne; $i < $endOne; $i++) { $one = mt_rand($i, $max); if ($index[$i] == $i) { $index[$i] = $index[$one]; $index[$one] = $i; } } return array_slice($index, 0, $len); }
The ingenuity of this algorithm compared with the above algorithm is:
Randomizes the self-increasing index, there will be no duplication problems, and it avoids deduplication. Overhead
Use the array subscript to replace the array itself for randomization. Every time a random number is obtained, it will be excluded from the value range. Next time, it will only be included in the remaining numbers. Fetching, the selection of random numbers can be completed in one traversal.
Related learning recommendations: PHP programming from entry to proficiency
The above is the detailed content of How to get random numbers without repetition in php?. For more information, please follow other related articles on the PHP Chinese website!
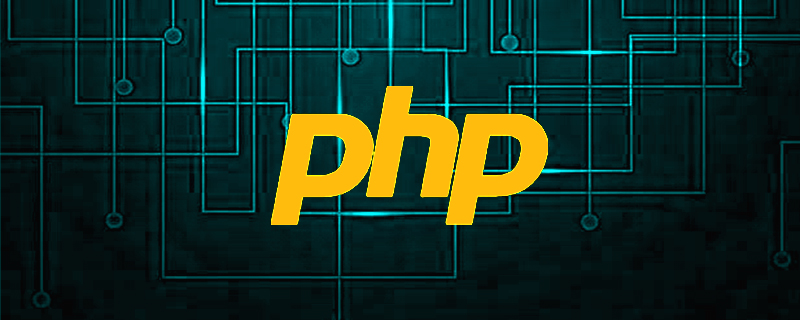
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
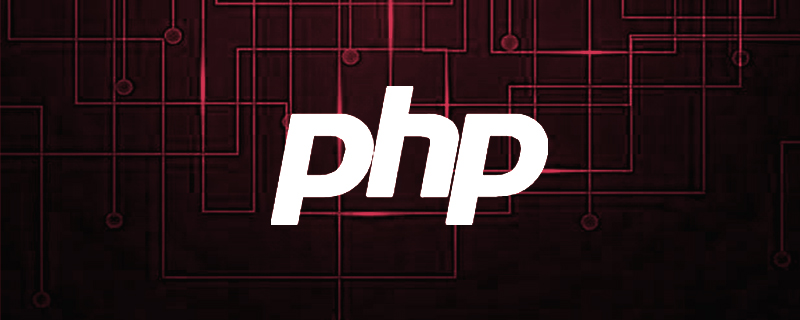
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
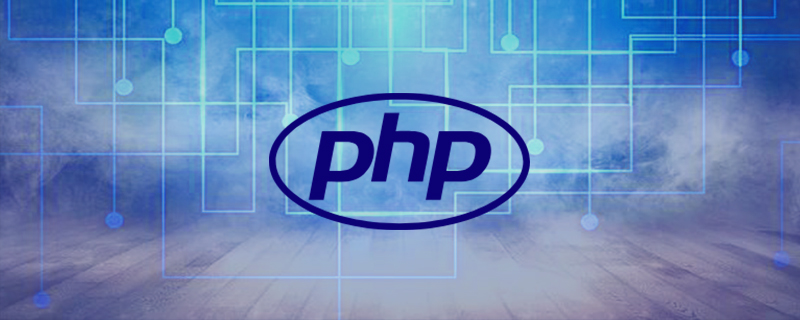
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
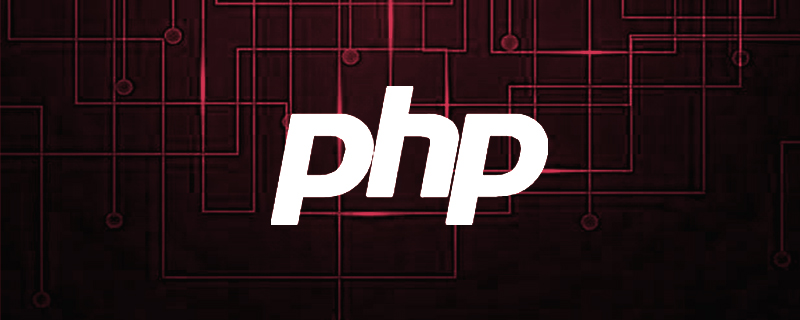
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
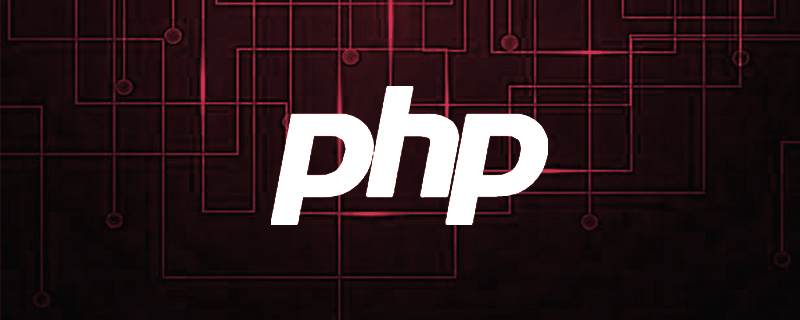
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
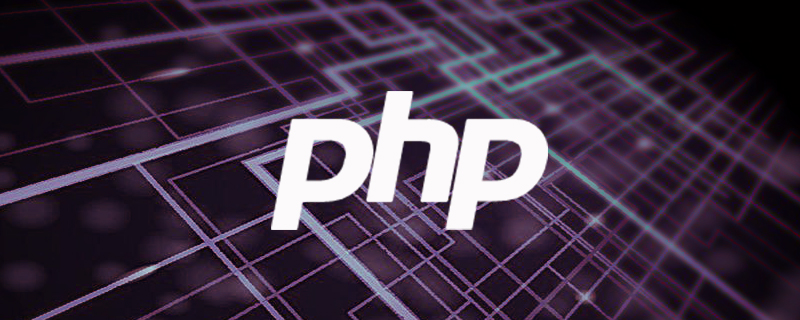
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
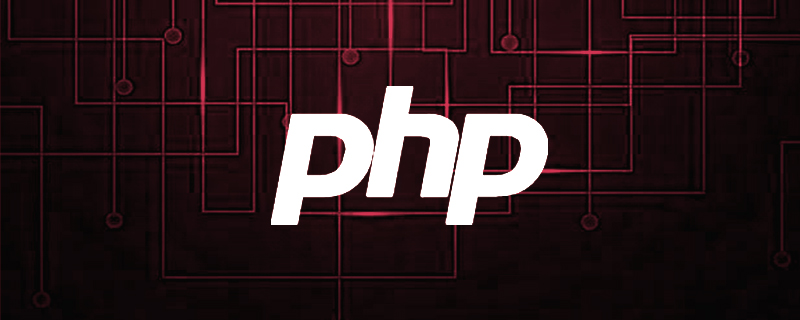
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
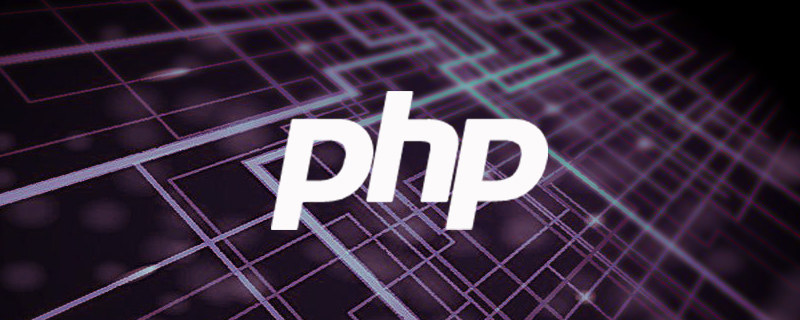
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
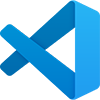
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
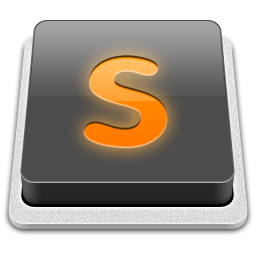
SublimeText3 Mac version
God-level code editing software (SublimeText3)
