PHP Design Patterns: The Path to Code Excellence
preface
PHP design patterns are important concepts that programmers apply during the development process to improve code quality and maintainability. PHP editor Xinyi has launched a series of articles called "PHP Design Patterns: The Road to Code Excellence" to provide in-depth analysis of the principles and practical applications of common design patterns to help readers better understand and apply design patterns, improve code levels, and achieve better results. Outstanding programming achievement.
Types of design patterns
There are many different design patterns, each with its own unique purpose and advantages. Here are some of the most commonly used php design patterns:
- Singleton mode: Ensure that a class has only one instance and provide a method to globally access this instance.
- Factory pattern: Creates an object without specifying its exact class. It allows developers to create different types of objects based on conditions.
- Strategy Pattern: Define a family of algorithms and make them interchangeable. It allows developers to change the algorithm at runtime.
- Observer pattern: Define a one-to-many dependency relationship between objects so that when one object changes, all dependent objects will be notified.
- Decorator pattern: Allows you to dynamically add functionality to an existing object without modifying its structure.
- Proxy mode: Provide an alternative or indirect access to another object. It allows developers to control access to objects and add additional functionality.
- Mediator pattern: Define a centralized object to manage communication between a series of objects. It minimizes coupling between objects and simplifies the communication process.
Single case mode demonstration
The following code shows the implementation of the singleton pattern in PHP:
class Singleton { private static $instance; private function __construct() { // 私有构造函数防止实例化多个对象 } public static function getInstance(): Singleton { if (!isset(self::$instance)) { self::$instance = new Singleton(); } return self::$instance; } }
Observer pattern demonstration
The following code shows the implementation of the observer pattern in PHP:
interface Observer { public function update(Subject $subject); } class Subject { private $observers = []; public function attach(Observer $observer) { $this->observers[] = $observer; } public function detach(Observer $observer) { $index = array_search($observer, $this->observers); if ($index !== false) { unset($this->observers[$index]); } } public function notify() { foreach ($this->observers as $observer) { $observer->update($this); } } }
Strategy Mode Demonstration
The following code shows the implementation of the strategy pattern in PHP:
interface Strategy { public function doOperation(); } class ConcreteStrategyA implements Strategy { public function doOperation() { echo "PerfORMing Operation A"; } } class ConcreteStrategyB implements Strategy { public function doOperation() { echo "Performing Operation B"; } } class Context { private $strategy; public function setStrategy(Strategy $strategy) { $this->strategy = $strategy; } public function doOperation() { $this->strategy->doOperation(); } }
Advantage
There are many advantages to using PHP design patterns, including:
- Code maintainability:Following patterns ensures that code conforms to established standards, making the code easier to understand, modify, and maintain.
- Code extensibility: Patterns make code easier to extend and reuse, thereby shortening development time and reducing maintenance costs.
- Code reliability: The design pattern has been verified and tested for a long time to ensure the robustness and reliability of the code.
- Team collaboration: Patterns provide a common language for development teams, promoting code understanding and collaboration.
in conclusion
PHP design patterns are powerful tools for improving code quality and maintainability. By following these patterns, developers can create applications that are scalable, reliable, and easy to maintain. Applying design patterns in practice can significantly improve development efficiency, reduce the risk of errors, and ensure the long-term sustainability of your code.
The above is the detailed content of PHP Design Patterns: The Path to Code Excellence. For more information, please follow other related articles on the PHP Chinese website!
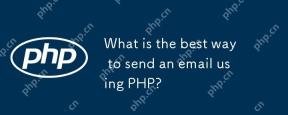
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
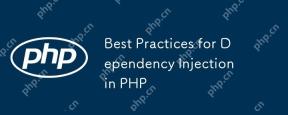
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
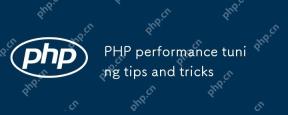
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
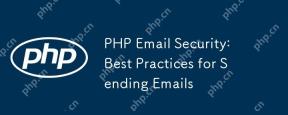
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
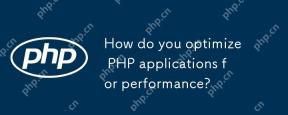
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
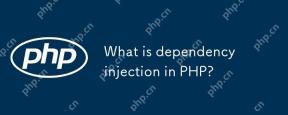
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
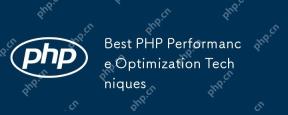
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
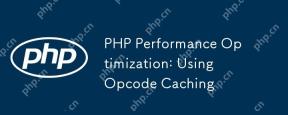
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
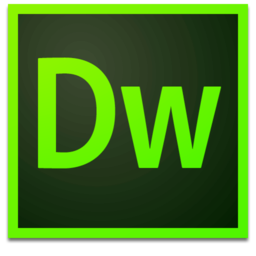
Dreamweaver Mac version
Visual web development tools
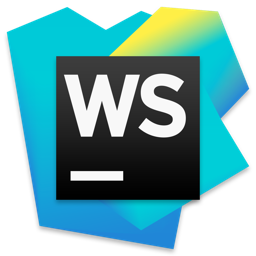
WebStorm Mac version
Useful JavaScript development tools
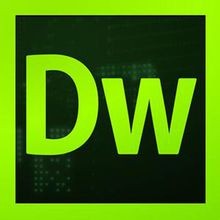
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
