How do you optimize PHP applications for performance?
To optimize PHP applications for performance, use caching, database optimization, opcode caching, and server configuration. 1) Implement caching with APCu to reduce data fetch times. 2) Optimize databases by indexing, balancing read and write operations. 3) Enable OPcache to avoid recompiling PHP code. 4) Configure PHP-FPM for efficient process management, and consider asynchronous programming with ReactPHP for handling multiple tasks concurrently.
Diving into PHP performance optimization is like embarking on a quest to make your code not just run, but soar. Imagine you've built a beautiful PHP application, but it's sluggish, like a snail trying to race in a Formula 1 circuit. What do you do? Let's explore how to turbocharge your PHP apps and share some battle scars from the trenches.
When it comes to optimizing PHP applications for performance, you're looking at a multi-faceted approach. It's not just about tweaking a few lines of code; it's about understanding your application's bottlenecks, leveraging the right tools, and sometimes, it's about knowing when to step back and let the server do the heavy lifting.
Let's dive into the nitty-gritty of PHP performance optimization. We'll cover everything from caching strategies that can make your app feel like it's running on rocket fuel, to database optimizations that can turn your slow queries into lightning-fast retrievals. We'll also touch on opcode caching, which is like giving your PHP interpreter a memory boost, and explore how to fine-tune your server settings for maximum efficiency.
First off, let's talk about caching. Caching is the secret sauce that can make your PHP application feel like it's running at the speed of light. By storing frequently accessed data in memory, you can drastically reduce the time it takes to fetch data from your database or regenerate content. Here's a simple example using PHP's built-in APCu (Alternative PHP Cache User) to cache the results of a computationally expensive function:
<?php if (!apcu_exists('expensive_data')) { $expensive_data = compute_expensive_data(); apcu_store('expensive_data', $expensive_data, 3600); // Cache for 1 hour } $data = apcu_fetch('expensive_data');
This snippet checks if the data is already cached, and if not, it computes it, stores it in the cache, and then fetches it. The beauty of caching is that it can significantly reduce server load and improve response times.
Now, let's talk about database optimization. Your database is often the bottleneck in your application, and optimizing your queries can have a dramatic impact on performance. Indexing is your best friend here. Consider this query:
<?php $query = "SELECT * FROM users WHERE email = 'user@example.com'";
Without an index on the email
column, this query might take ages on a large table. But with an index, it's lightning-fast. Here's how you can add an index in MySQL:
ALTER TABLE users ADD INDEX idx_email (email);
But indexing isn't a silver bullet. Over-indexing can slow down your write operations, so it's a balancing act. You need to analyze your query patterns and index accordingly.
Opcode caching is another game-changer. PHP's opcode cache, like OPcache, compiles your PHP code into machine-readable instructions and stores them in memory. This means your server doesn't have to recompile your code on every request, which can save a significant amount of time. Here's how you can enable OPcache in your php.ini
:
opcache.enable=1 opcache.memory_consumption=128 opcache.interned_strings_buffer=8 opcache.max_accelerated_files=4000
These settings are just a starting point, and you'll need to tweak them based on your specific application's needs.
Server configuration is also crucial. PHP-FPM (FastCGI Process Manager) can help you manage PHP processes more efficiently. Here's a basic configuration for PHP-FPM:
[www] user = www-data group = www-data listen = /run/php/php7.4-fpm.sock listen.owner = www-data listen.group = www-data pm = dynamic pm.max_children = 50 pm.start_servers = 5 pm.min_spare_servers = 5 pm.max_spare_servers = 35
This configuration tells PHP-FPM to start with 5 processes and scale up to 50 if needed. Tuning these settings can help you handle more concurrent requests without overloading your server.
Now, let's talk about some advanced techniques. Have you ever considered using asynchronous programming in PHP? With libraries like ReactPHP, you can write non-blocking code that can handle multiple tasks simultaneously. Here's a simple example of using ReactPHP to fetch data from multiple APIs concurrently:
<?php require 'vendor/autoload.php'; $loop = React\EventLoop\Factory::create(); $dnsResolverFactory = new React\Dns\Resolver\Factory(); $dns = $dnsResolverFactory->create('8.8.8.8', $loop); $connector = new React\Socket\Connector($loop, [ 'dns' => $dns ]); $httpClient = new React\Http\Browser($connector); $promises = []; $urls = ['https://api1.example.com/data', 'https://api2.example.com/data']; foreach ($urls as $url) { $promises[] = $httpClient->get($url)->then(function (Psr\Http\Message\ResponseInterface $response) { return $response->getBody()->getContents(); }); } React\Promise\all($promises)->then(function (array $results) { foreach ($results as $result) { echo $result . "\n"; } }); $loop->run();
This code fetches data from multiple APIs at the same time, which can significantly improve the performance of your application, especially when dealing with external services.
But be warned, asynchronous programming can be a double-edged sword. It can lead to complex code that's hard to debug and maintain. You need to carefully consider whether the performance gains are worth the added complexity.
In conclusion, optimizing PHP applications for performance is a journey, not a destination. It's about understanding your application's unique needs, experimenting with different techniques, and constantly monitoring and tweaking your setup. Whether it's caching, database optimization, opcode caching, or server configuration, each piece plays a crucial role in making your application run smoothly. And remember, sometimes the best optimization is to write clean, efficient code from the start. Happy optimizing!
The above is the detailed content of How do you optimize PHP applications for performance?. For more information, please follow other related articles on the PHP Chinese website!
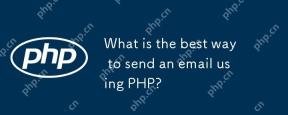
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
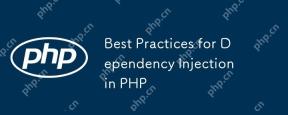
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
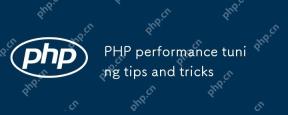
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
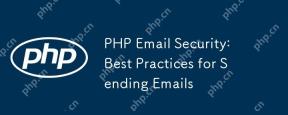
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
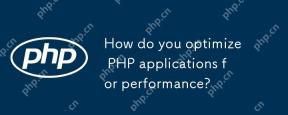
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
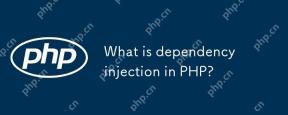
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
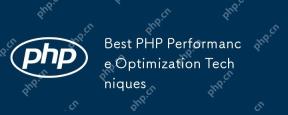
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
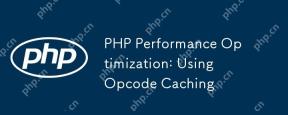
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
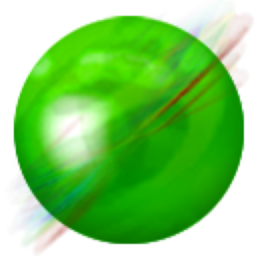
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
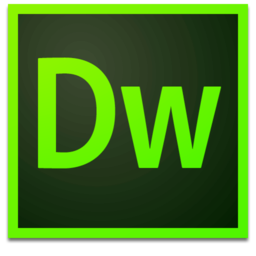
Dreamweaver Mac version
Visual web development tools
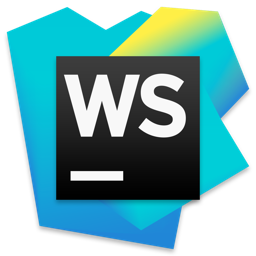
WebStorm Mac version
Useful JavaScript development tools
