Dependency injection in PHP is a design pattern that enhances flexibility, testability, and maintainability by providing external dependencies to classes. It allows for loose coupling, easier testing through mocking, and modular design, but requires careful structuring to avoid over-injection and may benefit from using a dependency injection container for complex projects.
Dependency injection in PHP is a design pattern that helps in achieving loose coupling between classes and their dependencies. It's a technique where one object supplies the dependencies of another object. This means instead of a class creating its own dependencies internally, these are provided to it from the outside, usually through constructor arguments, setter methods, or interfaces.
Now, let's dive deeper into what dependency injection in PHP really means and why it's a game-changer in the world of software development.
Dependency injection is all about flexibility and testability. Imagine you're building a spaceship, and every component needs to be easily replaceable and testable. In PHP, this translates to writing classes that can be swapped out or mocked during testing without changing the core logic. It's like having a modular system where you can plug in different engines or navigation systems without rebuilding the entire ship.
When I first started using dependency injection, it felt like a revelation. My code became cleaner, more modular, and easier to maintain. But it's not without its challenges. You need to think about how to structure your code to make the most of this pattern. It's not just about injecting dependencies; it's about understanding the flow of your application and how each component interacts with others.
Let's look at a simple example to illustrate this concept:
class Logger { public function log($message) { echo $message . "\n"; } } class UserService { private $logger; public function __construct(Logger $logger) { $this->logger = $logger; } public function createUser($username) { // Create user logic here $this->logger->log("User created: $username"); } } $logger = new Logger(); $userService = new UserService($logger); $userService->createUser("JohnDoe");
In this example, UserService
depends on Logger
. Instead of creating the Logger
inside UserService
, we inject it through the constructor. This approach allows us to easily swap out different implementations of Logger
without changing UserService
.
One of the key benefits of dependency injection is that it makes your code more testable. You can easily mock the Logger
class in unit tests for UserService
. Here's how you might do that:
class MockLogger { public function log($message) { // Mock logging logic } } $mockLogger = new MockLogger(); $userService = new UserService($mockLogger); $userService->createUser("TestUser");
This flexibility is crucial when you're working on large projects or when you need to ensure that your code can adapt to changing requirements.
However, there are some pitfalls to watch out for. Overuse of dependency injection can lead to what's known as "constructor over-injection," where your constructors have too many parameters, making them hard to read and maintain. To avoid this, you might want to group related dependencies into a single object or use a dependency injection container.
Dependency injection containers are powerful tools that can manage the creation and injection of dependencies across your application. They can help reduce boilerplate code and make it easier to manage complex dependency graphs. Here's a simple example using a container:
class Container { private $instances = []; public function set($key, $value) { $this->instances[$key] = $value; } public function get($key) { return $this->instances[$key] ?? null; } } $container = new Container(); $container->set(Logger::class, new Logger()); $userService = new UserService($container->get(Logger::class)); $userService->createUser("JaneDoe");
Using a container can simplify your code, but it also adds another layer of complexity. You need to decide if the benefits outweigh the added complexity for your project.
In practice, I've found that dependency injection encourages better design patterns and helps in creating more maintainable code. It forces you to think about the structure of your application and how different components interact. It's not just a technical solution; it's a mindset shift towards more modular and flexible software design.
When implementing dependency injection, consider the following tips:
- Start small. Don't try to refactor your entire codebase at once. Begin with new classes or modules.
- Use interfaces to define dependencies. This allows for easier swapping of implementations.
- Be mindful of the number of dependencies. Too many can lead to tight coupling and make your code harder to maintain.
- Consider using a dependency injection container for larger projects, but weigh the added complexity against the benefits.
In conclusion, dependency injection in PHP is a powerful tool for creating more flexible, testable, and maintainable code. It's not just about injecting dependencies; it's about embracing a design philosophy that can transform how you build software. With the right approach, it can make your development process smoother and your applications more robust.
The above is the detailed content of What is dependency injection in PHP?. For more information, please follow other related articles on the PHP Chinese website!
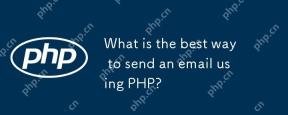
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
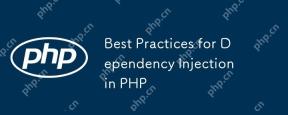
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
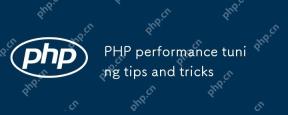
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
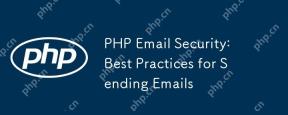
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
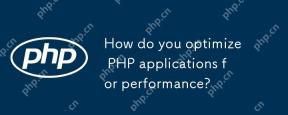
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
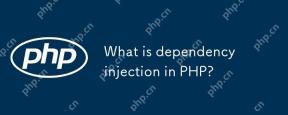
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
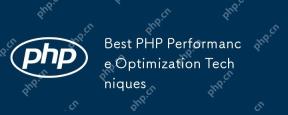
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
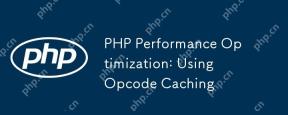
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor
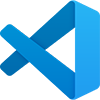
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
