PHP performance tuning is crucial because it enhances speed and efficiency, which are vital for web applications. 1) Caching with APCu reduces database load and improves response times. 2) Optimizing database queries by selecting necessary columns and using indexing speeds up data retrieval. 3) Upgrading to PHP 7.x significantly boosts performance due to its improvements over older versions.
When it comes to PHP performance tuning, the journey is as important as the destination. You might ask, why is PHP performance tuning crucial? Well, in a world where speed and efficiency can make or break your web application, optimizing PHP code isn't just a nice-to-have; it's a must-have. It's about making your application not just functional but also fast and resource-efficient. Let's dive deep into the art of PHP performance tuning, where we'll explore some tips and tricks, share personal experiences, and even touch on the pitfalls you might encounter along the way.
Let's start with a personal anecdote. Once upon a time, I was working on a PHP-based e-commerce platform that was struggling under the weight of its own success. The site was slow, and customers were leaving in droves. After some intense performance tuning, we managed to reduce the load time by over 50%, which not only improved user satisfaction but also significantly increased our conversion rates. This experience taught me that performance tuning is not just about code; it's about understanding the entire ecosystem of your application.
Now, let's delve into some PHP performance tuning tips and tricks. I'll share some code examples that reflect my personal style, which might be a bit unconventional but effective.
Caching is Your Friend
Caching is one of the most powerful tools in your performance tuning arsenal. By storing frequently accessed data in memory, you can dramatically reduce the load on your database and improve response times. Here's a simple example using PHP's built-in APCu (Alternative PHP Cache User):
// Using APCu for caching function getExpensiveData($key) { $data = apcu_fetch($key); if ($data === false) { // Simulating expensive operation $data = expensiveOperation(); apcu_store($key, $data, 3600); // Cache for 1 hour } return $data; } <p>function expensiveOperation() { // Your expensive operation here return "Expensive data"; }</p>
This approach can save you from repeatedly performing costly operations. However, be mindful of cache invalidation strategies to ensure you're not serving stale data.
Optimize Database Queries
Database queries are often a bottleneck in PHP applications. One of my favorite tricks is to use EXPLAIN to analyze query performance and then optimize accordingly. Here's an example of how you might optimize a query:
// Original query $query = "SELECT * FROM users WHERE status = 'active'"; <p>// Optimized query $query = "SELECT id, name, email FROM users WHERE status = 'active'";</p>
By selecting only the necessary columns, you reduce the amount of data transferred and processed. Additionally, consider using indexing on frequently queried columns to speed up lookups.
Use PHP 7.x and Beyond
PHP 7.x introduced significant performance improvements over its predecessors. If you're still using an older version, upgrading can be one of the easiest ways to boost performance. Here's a quick benchmark to illustrate:
// Benchmarking PHP 5.6 vs PHP 7.4 $php56 = shell_exec('time php56 -r "for (\$i = 0; \$i echo "PHP 5.6: $php56\n"; echo "PHP 7.4: $php74\n";
The results will show a significant speed difference, highlighting the importance of staying up-to-date with PHP versions.
Embrace Asynchronous Processing
For operations that don't need to be synchronous, consider using asynchronous processing. This can free up your main thread to handle other requests. Here's a simple example using PHP's pcntl_fork
:
// Asynchronous processing example function processInBackground($data) { $pid = pcntl_fork(); if ($pid == -1) { die('Could not fork'); } else if ($pid) { // Parent process return; } else { // Child process longRunningTask($data); exit(0); } } <p>function longRunningTask($data) { // Your long-running task here sleep(10); }</p>
This approach can significantly improve the responsiveness of your application, but be cautious about resource management and potential race conditions.
Code Profiling and Optimization
Profiling your code is essential for identifying performance bottlenecks. Tools like Xdebug and Blackfire can help you pinpoint where your application is spending most of its time. Here's a simple example of how to use Xdebug for profiling:
// Enable Xdebug profiling xdebug_start_profiling(); <p>// Your code here for ($i = 0; $i </p><p>// Stop profiling and save the data xdebug_stop_profiling();</p>
After running this, you can analyze the profiling data to see where optimizations are needed. Remember, though, that profiling can introduce overhead, so use it judiciously.
Avoid Common Pitfalls
While tuning PHP performance, it's easy to fall into traps. One common mistake is over-optimizing, where you spend too much time on minor improvements that don't significantly impact overall performance. Another pitfall is neglecting to test your optimizations thoroughly, which can lead to unexpected bugs or performance regressions.
In my experience, a balanced approach is key. Focus on the areas that will give you the most significant gains, and always measure before and after to ensure your efforts are paying off.
Conclusion
PHP performance tuning is an ongoing journey, not a one-time task. By leveraging caching, optimizing database queries, staying current with PHP versions, embracing asynchronous processing, and using profiling tools, you can significantly enhance your application's performance. Remember, though, that every application is unique, and what works for one might not work for another. Keep experimenting, measuring, and refining your approach, and you'll find the sweet spot that makes your PHP application not just functional but truly exceptional.
The above is the detailed content of PHP performance tuning tips and tricks. For more information, please follow other related articles on the PHP Chinese website!
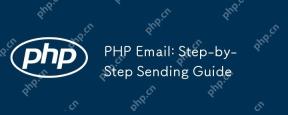
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
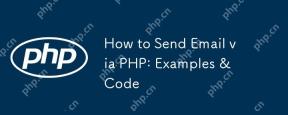
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
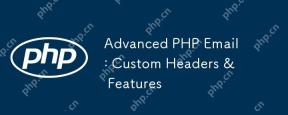
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
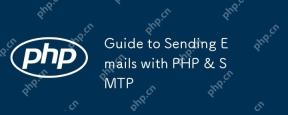
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
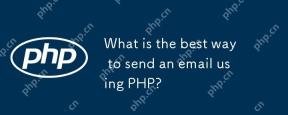
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
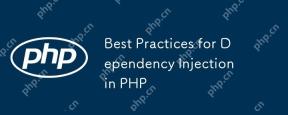
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
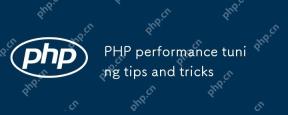
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
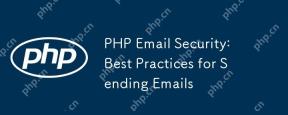
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
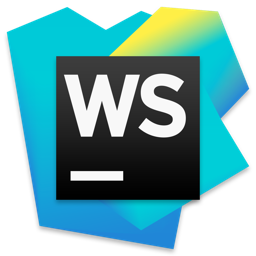
WebStorm Mac version
Useful JavaScript development tools
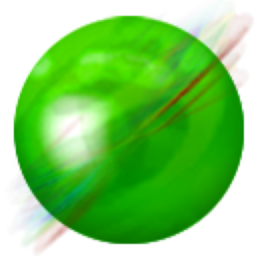
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
