Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
When it comes to sending emails using PHP with SMTP, it's not just about getting the code to work. It's about understanding the nuances of email protocols, the security implications, and how to ensure your emails land in the inbox rather than the spam folder. Let's dive deep into this topic, sharing not just the how-to but also the why and the gotchas.
Sending emails programmatically is a common task for web developers, whether it's for user registration confirmations, password resets, or marketing campaigns. PHP, being a popular server-side language, offers several ways to send emails, but using SMTP (Simple Mail Transfer Protocol) is often preferred for its reliability and flexibility.
Let's start with the basics. SMTP is the standard protocol for sending emails across the internet. When you use PHP's mail()
function, it typically relies on the server's local mail transfer agent (MTA), which can be problematic due to configuration issues or security restrictions. By using an SMTP server directly, you have more control over the sending process.
Here's a simple example of how you can send an email using PHP with SMTP:
<?php require 'PHPMailer/PHPMailerAutoload.php'; $mail = new PHPMailer; $mail->isSMTP(); $mail->Host = 'smtp.example.com'; $mail->SMTPAuth = true; $mail->Username = 'your_username'; $mail->Password = 'your_password'; $mail->SMTPSecure = 'tls'; $mail->Port = 587; $mail->setFrom('from@example.com', 'Your Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); $mail->isHTML(true); $mail->Subject = 'Subject'; $mail->Body = 'This is the HTML message body <b>in bold!</b>'; $mail->AltBody = 'This is the body in plain text for non-HTML mail clients'; if(!$mail->send()) { echo 'Message could not be sent.'; echo 'Mailer Error: ' . $mail->ErrorInfo; } else { echo 'Message has been sent'; } ?>
This example uses the PHPMailer library, which is widely recommended due to its ease of use and robust feature set. However, let's unpack this further.
The choice of SMTP server is cruel. You can use your own server if you have one set up, or you can use third-party services like Gmail, SendGrid, or Mailgun. Each has its own setup requirements and limitations. For instance, using Gmail requires enabling "Less secure app access" or using OAuth2, which adds another layer of complexity.
Security is another critical aspect. Always use encryption (TLS or SSL) when connecting to the SMTP server. The example above uses TLS on port 587, which is common for modern SMTP servers. However, some servers might use SSL on port 465. It's important to check your SMTP provider's documentation.
Now, let's talk about some advanced scenarios and potential pitfalls.
When sending bulk emails, you need to be aware of rate limiting. Most SMTP servers have limits on how many emails you can send per hour or per day. Exceeding these limits can result in your emails being blocked or your account being suspended. To mitigate this, consider using a service like SendGrid, which is designed for high-volume email sending and provides better delivery ability metrics.
Another common issue is emails landing in the spam folder. This can happen for various reasons, including:
- Your domain or IP has a poor reputation.
- The email content triggers spam filters.
- You're not using proper authentication methods like SPF, DKIM, and DMARC.
To improve delivery ability, ensure your domain is set up correctly with the necessary DNS records. Also, avoid using words or phrases commonly associated with spam, and always include a clear unsubscribe option in your emails.
Let's look at a more advanced example that includes authentication and better error handling:
<?php require 'PHPMailer/PHPMailerAutoload.php'; $mail = new PHPMailer(true); try { $mail->isSMTP(); $mail->Host = 'smtp.sendgrid.net'; $mail->SMTPAuth = true; $mail->Username = 'apikey'; $mail->Password = 'your_sendgrid_api_key'; $mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS; $mail->Port = 587; $mail->setFrom('from@example.com', 'Your Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); $mail->isHTML(true); $mail->Subject = 'Subject'; $mail->Body = 'This is the HTML message body <b>in bold!</b>'; $mail->AltBody = 'This is the body in plain text for non-HTML mail clients'; $mail->send(); echo 'Message has been sent'; } catch (Exception $e) { echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}"; } ?>
This example uses SendGrid as the SMTP provider, which is a good choice for production environments. It also uses a try-catch block for better error handling, which is essential for robust applications.
In terms of performance, consider using asynchronous sending if you're dealing with a large number of emails. PHP's mail()
function is synchronous, but with PHPMailer, you can use the send()
method in a loop to send multiple emails, which can be time-consuming. For high-volume scenarios, look into using a queue system like RabbitMQ or a service like Amazon SES.
Lastly, let's touch on some best practices:
- Always validate and sanitize user input to prevent email header injection attacks.
- Use environment variables or a configuration file to store sensitive information like SMTP credentials.
- Test your email setup thoroughly in different environments (development, staging, production).
- Monitor your email sending metrics and adjust your strategy based on delivery ability and engagement data.
Sending emails with PHP and SMTP is a powerful tool, but it comes with its challenges. By understanding the underlying protocols, choosing the right tools, and following best practices, you can ensure your emails are delivered effectively and securely.
The above is the detailed content of Guide to Sending Emails with PHP & SMTP. For more information, please follow other related articles on the PHP Chinese website!
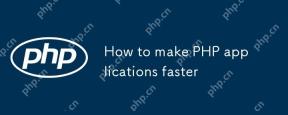
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
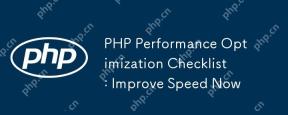
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
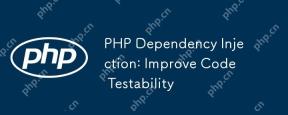
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
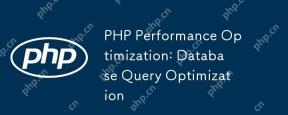
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
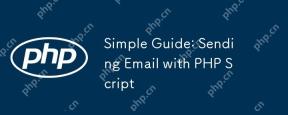
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
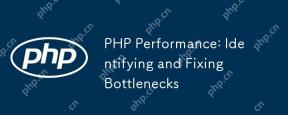
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
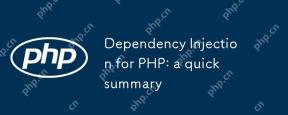
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
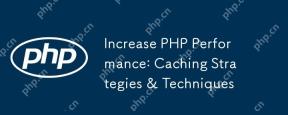
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
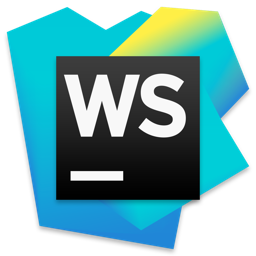
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
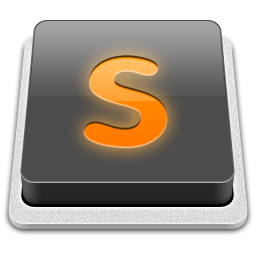
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
