Increase PHP Performance: Caching Strategies & Techniques
Caching improves PHP performance by storing results of computations or queries for quick retrieval, reducing server load and enhancing response times. Effective strategies include: 1) Opcode caching, which stores compiled PHP scripts in memory to skip compilation; 2) Data caching using Memcached or Redis to store query results; 3) Page caching for static content; 4) Full-page caching with reverse proxies like Varnish; 5) Database query caching to reduce database load.
When it comes to enhancing PHP performance, one of the most effective approaches is through caching strategies and techniques. Caching can dramatically reduce the load on your server, improve response times, and enhance the overall user experience. But how exactly does caching improve PHP performance, and what are the best practices to implement it effectively?
Caching works by storing the results of expensive computations or database queries so that subsequent requests can retrieve this data quickly without having to perform the operation again. This is particularly beneficial for PHP applications because PHP, being an interpreted language, can be resource-intensive. By caching data, you can bypass the need for repetitive processing, which is where PHP's performance often bottlenecks.
Let's dive into some of the most effective caching strategies and techniques that I've used and seen in action:
Opcode Caching
Opcode caching is a game-changer for PHP performance. PHP scripts are compiled into opcodes before execution, and with opcode caching, these opcodes are stored in memory. This means that subsequent requests can skip the compilation step entirely, leading to significant performance gains. I've implemented OPcache in several projects, and the difference is night and day.
Here's a snippet of how you might configure OPcache in your php.ini
:
opcache.enable=1 opcache.memory_consumption=128 opcache.interned_strings_buffer=8 opcache.max_accelerated_files=4000 opcache.revalidate_freq=60 opcache.fast_shutdown=1 opcache.enable_cli=1
One thing to watch out for with OPcache is that it can lead to stale code if not configured properly. The revalidate_freq
setting, for instance, controls how often OPcache checks for updated scripts. Set it too high, and you might miss updates; too low, and you might lose some performance benefits.
Data Caching
Data caching involves storing the results of database queries or API calls. This is where libraries like Memcached or Redis come into play. I've found Memcached to be particularly useful for its simplicity and speed, but Redis offers more advanced features like persistence and pub/sub messaging, which can be invaluable in certain scenarios.
Here's an example of using Memcached to cache a database query result:
$memcache = new Memcache; $memcache->connect('localhost', 11211) or die ("Could not connect"); $key = 'user_data_123'; if (($data = $memcache->get($key)) === false) { // Data not found in cache, fetch from database $data = fetchUserDataFromDatabase(123); $memcache->set($key, $data, 0, 3600); // Cache for 1 hour } // Use $data
The key to effective data caching is to strike a balance between cache freshness and performance. You don't want to hit the database every time, but you also don't want to serve outdated data. Implementing a proper cache invalidation strategy is crucial here. I've seen projects go awry because they didn't account for cache invalidation, leading to inconsistent data across the application.
Page Caching
Page caching is about storing the entire output of a page, which can be particularly useful for static or semi-static content. I've used this technique on e-commerce sites where product pages don't change frequently. Here's a simple example of how you might implement page caching:
$cacheFile = 'cache/homepage.html'; if (file_exists($cacheFile) && (filemtime($cacheFile) > (time() - 3600 ))) { // Serve from cache echo file_get_contents($cacheFile); exit; } else { // Generate the page ob_start(); // Your PHP code to generate the page goes here $pageContent = ob_get_clean(); // Save to cache file_put_contents($cacheFile, $pageContent); // Serve the page echo $pageContent; }
The challenge with page caching is managing cache invalidation, especially when dealing with user-specific content or dynamic elements. You might need to implement a more sophisticated system that can selectively invalidate parts of the cache.
Full-Page Caching with Reverse Proxies
For even more performance, consider using a reverse proxy like Varnish. Varnish can cache entire HTTP responses, which means it can serve content directly without even hitting your PHP application. I've used Varnish on high-traffic sites, and it's incredibly effective, but it does require careful configuration to ensure it's caching what you want it to.
Here's a basic VCL (Varnish Configuration Language) snippet:
vcl 4.0; backend default { .host = "127.0.0.1"; .port = "8080"; } sub vcl_recv { if (req.url ~ "\.(css|js|jpg|jpeg|png|gif|ico)$") { return (hash); } } sub vcl_backend_response { if (bereq.url ~ "\.(css|js|jpg|jpeg|png|gif|ico)$") { set beresp.ttl = 1h; } }
Varnish can be a bit complex to set up, but the performance gains are worth it. Just be aware that you'll need to configure it to handle user sessions and dynamic content correctly.
Database Query Caching
Lastly, don't overlook the power of database query caching. Many databases, like MySQL, have built-in query caching mechanisms. Here's how you might enable query caching in MySQL:
SET GLOBAL query_cache_type = ON; SET GLOBAL query_cache_size = 64M;
This can significantly reduce the load on your database, but be cautious. Query caching can lead to issues if not managed properly, especially with frequent data updates.
In conclusion, caching is a powerful tool for boosting PHP performance, but it's not without its challenges. From opcode caching to full-page caching with reverse proxies, each technique has its place and its pitfalls. The key is to understand your application's specific needs and implement a caching strategy that balances performance with data freshness and consistency. Remember, the devil is in the details—proper configuration and cache invalidation strategies are crucial to reaping the full benefits of caching.
The above is the detailed content of Increase PHP Performance: Caching Strategies & Techniques. For more information, please follow other related articles on the PHP Chinese website!
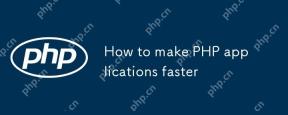
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
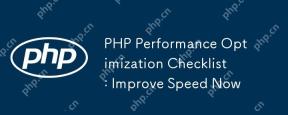
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
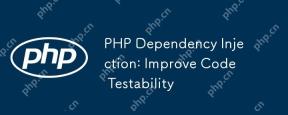
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
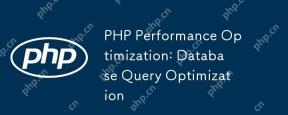
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
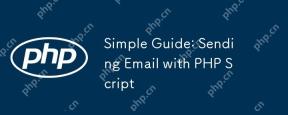
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
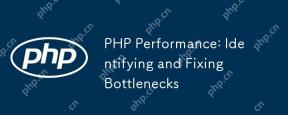
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
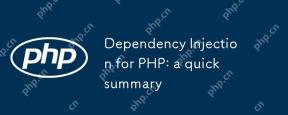
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
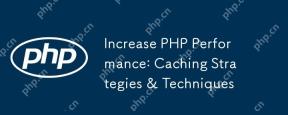
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
