The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
When it comes to dependency injection (DI) in PHP, the question often arises: why should I both with it? Well, dependency injection is not just a fancy buzzword; it's a powerful design pattern that promotes loose coupling, testability, and maintainability of your code. By using DI, you can easily swap out dependencies, making your application more flexible and easier to test. But let's dive deeper into the world of DI in PHP and explore some best practices that can truly elevate your coding game.
Let's talk about the essence of dependency injection first. Imagine you're building a house, and instead of having all the tools and materials permanently fixed in place, you have a system where you can easily swap out tools or change materials based on what's needed at the moment. That's what DI does for your code. It allows you to inject dependencies into your classes rather than having them hard-coded, making your code more modular and adaptable.
Here's a simple example of how you might implement DI in PHP:
class Logger { public function log($message) { echo "Logging: " . $message . "\n"; } } class UserService { private $logger; public function __construct(Logger $logger) { $this->logger = $logger; } public function registerUser($username) { $this->logger->log("Registering user: " . $username); // User registration logic here } } $logger = new Logger(); $userService = new UserService($logger); $userService->registerUser("john_doe");
In this example, UserService
depends on Logger
, but instead of creating a Logger
instance inside UserService
, it receives it through its constructor. This approach makes UserService
more flexible because you can easily switch out the logger with a different implementation if needed.
Now, let's explore some best practices for implementing dependency injection in PHP that go beyond the basics:
Use Constructor Injection Whenever Possible
Construction injection is the most common and recommended way to inject dependencies. It makes it clear what dependencies a class needs right from the start. Here's how you might refine the previous example:
class UserService { private $logger; public function __construct(LoggerInterface $logger) { $this->logger = $logger; } // ... rest of the class } interface LoggerInterface { public function log($message); } class ConsoleLogger implements LoggerInterface { public function log($message) { echo "Console: " . $message . "\n"; } } class FileLogger implements LoggerInterface { public function log($message) { file_put_contents('log.txt', $message . "\n", FILE_APPEND); } } $consoleLogger = new ConsoleLogger(); $fileLogger = new FileLogger(); $userServiceWithConsole = new UserService($consoleLogger); $userServiceWithFile = new UserService($fileLogger);
By using an interface, LoggerInterface
, you decouple UserService
from specific implementations of the logger. This approach allows you to easily switch between different logging mechanisms without changing the UserService
class.
Avoid Service Locators
Service locators can seem like a convenient way to access dependencies, but they often lead to tightly coupled code and can hide dependencies, making your code harder to test and maintain. Instead of using a service locator, stick to explicit dependency injection. Here's an example of what to avoid:
class BadUserService { public function registerUser($username) { $logger = ServiceLocator::getLogger(); $logger->log("Registering user: " . $username); // User registration logic here } }
This approach makes it harder to see what dependencies BadUserService
has, and it can lead to issues when testing or when you want to switch out the logger.
Leverage Dependency Injection Containers
For larger applications, manually managing dependencies can become cumbersome. That's where dependency injection containers come in handy. They help you manage and wire up your dependencies automatically. Popular PHP DI containers include Symfony's DependencyInjection component and PHP-DI. Here's a quick look at how you might use PHP-DI:
use DI\Container; $container = new Container(); $container->set('logger', function () { return new ConsoleLogger(); }); $container->set('user_service', function (Container $c) { return new UserService($c->get('logger')); }); $userService = $container->get('user_service'); $userService->registerUser("john_doe");
Using a DI container can significantly simplify your dependency management, especially in large applications. However, be cautious not to overuse containers, as they can introduce complexity if not managed properly.
Testability and Mocking
One of the biggest advantages of DI is improved testability. By injecting dependencies, you can easily mock them in your tests. Here's how you might test UserService
using PHPUnit and mocking:
use PHPUnit\Framework\TestCase; use PHPUnit\Framework\MockObject\MockObject; class UserServiceTest extends TestCase { public function testRegisterUser() { /** @var LoggerInterface|MockObject $logger */ $logger = $this->createMock(LoggerInterface::class); $logger->expects($this->once()) -> method('log') ->with('Registering user: john_doe'); $userService = new UserService($logger); $userService->registerUser('john_doe'); } }
This test ensures that the log
method of the logger is called exactly once with the correct message, without actually logging anything.
Avoid Over-Injection
While DI is powerful, over-injecting dependencies can lead to classes that are too complex and hard to understand. If a class has too many dependencies, it might be a sign that the class is doing too much and should be broken down into smaller, more focused classes. Here's an example of what to avoid:
class OverloadedService { private $logger; private $database; private $mailer; private $cache; // ... many more dependencies public function __construct(LoggerInterface $logger, Database $database, Mailer $mailer, Cache $cache, /* ... */) { $this->logger = $logger; $this->database = $database; $this->mailer = $mailer; $this->cache = $cache; // ... many more assignments } // ... methods using all these dependencies }
Instead, try to keep your classes focused and inject only what is necessary.
Consider the Performance Impact
While DI generally improves code quality, it can have a slight performance impact due to the additional indirection. In most cases, this impact is negligible, but in performance-critical applications, you might want to measure the impact of DI and consider optimizing where necessary. For example, you might cache frequently used objects or use lazy loading for dependencies that are not always needed.
Conclusion
Dependency injection in PHP is more than just a technique; it's a mindset that promotes better software design. By following these best practices, you can make your code more modular, testable, and maintainable. Remember, the key is to keep your classes focused, use interfaces to define dependencies, and leverage DI containers for larger applications. And always keep an eye on performance, ensuring that your use of DI doesn't introduce unnecessary overhead. With these principles in mind, you'll be well on your way to writing cleaner, more robust PHP code.
The above is the detailed content of Best Practices for Dependency Injection in PHP. For more information, please follow other related articles on the PHP Chinese website!
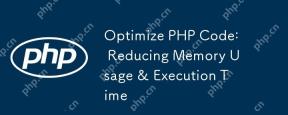
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
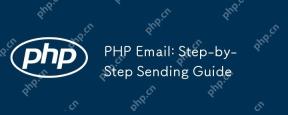
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
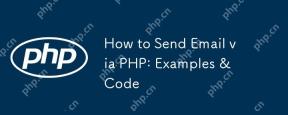
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
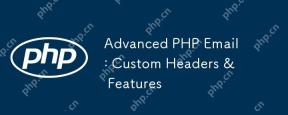
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
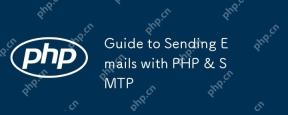
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
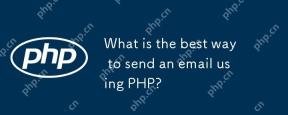
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
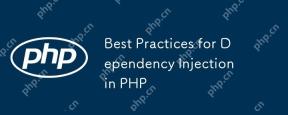
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
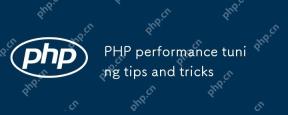
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
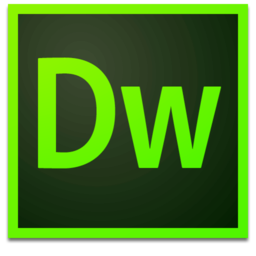
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
