Optimize PHP Code: Reducing Memory Usage & Execution Time
To optimize PHP code for reduced memory usage and execution time, follow these steps: 1) Use references instead of copying large data structures to reduce memory consumption. 2) Leverage PHP's built-in functions like array_map for faster execution. 3) Implement caching mechanisms, such as APCu, to decrease server load and execution time for repeated operations. These techniques, when applied thoughtfully, can significantly enhance your PHP code's performance.
When it comes to optimizing PHP code, reducing memory usage and execution time is often at the forefront of developers' minds. So, how can we achieve this? Let's dive into the world of PHP optimization, where I'll share some personal insights and practical examples to help you streamline your code.
The quest for efficiency in PHP begins with understanding the language's quirks and capabilities. I remember working on a large-scale e-commerce platform where every millisecond counted. We had to optimize our PHP scripts to handle thousands of concurrent users without breaking a sweat. This experience taught me that optimization is not just about writing less code; it's about writing smarter code.
Let's start with memory usage. One of the most effective ways to reduce memory consumption is by using references instead of copying large data structures. Here's a quick example to illustrate:
// Before: Copying the entire array $largeArray = range(1, 1000000); $newArray = $largeArray; // After: Using a reference $largeArray = range(1, 1000000); $newArray =& $largeArray;
By using the reference operator &
, we ensure that $newArray
points to the same memory location as $largeArray
, significantly reducing memory overhead. This technique is particularly useful when dealing with large datasets or when passing arrays to functions.
Now, let's talk about execution time. One of my favorite techniques is to leverage PHP's built-in functions, which are often more optimized than custom code. For instance, consider the difference between using array_map
and a traditional foreach
loop:
// Before: Using a foreach loop $numbers = range(1, 1000000); $squaredNumbers = []; foreach ($numbers as $number) { $squaredNumbers[] = $number * $number; } // After: Using array_map $numbers = range(1, 1000000); $squaredNumbers = array_map(function($number) { return $number * $number; }, $numbers);
The array_map
function is not only more concise but also generally faster, especially for larger arrays. However, it's worth noting that for very small datasets, the difference might be negligible, so always benchmark your code to ensure the optimization is worthwhile.
Another powerful optimization technique is caching. I've seen projects where implementing a simple caching mechanism reduced server load by over 50%. Here's a basic example using PHP's APCu (Alternative PHP Cache User):
// Before: No caching function expensiveCalculation($input) { // Simulating an expensive calculation sleep(2); return $input * 2; } // After: Using APCu for caching function expensiveCalculation($input) { $cacheKey = 'expensive_calculation_' . $input; $cachedResult = apcu_fetch($cacheKey); if ($cachedResult !== false) { return $cachedResult; } // Simulating an expensive calculation sleep(2); $result = $input * 2; apcu_store($cacheKey, $result, 3600); // Cache for 1 hour return $result; }
Caching can dramatically reduce execution time for operations that are repeated frequently, but it's crucial to consider cache invalidation strategies to ensure data consistency.
When optimizing PHP code, it's also important to be aware of potential pitfalls. For instance, while using references can save memory, it can also lead to unexpected behavior if not managed carefully. Similarly, aggressive caching might lead to stale data if not properly maintained. Always weigh the benefits against the potential drawbacks and test thoroughly in a staging environment before deploying to production.
In terms of best practices, I've found that keeping functions small and focused, using meaningful variable names, and writing clear, concise comments can make a big difference in code maintainability, which indirectly impacts performance. Additionally, regularly profiling your code with tools like Xdebug or Blackfire can help identify bottlenecks that might not be immediately apparent.
In conclusion, optimizing PHP code for reduced memory usage and execution time is a multifaceted challenge that requires a deep understanding of the language and its ecosystem. By applying techniques like using references, leveraging built-in functions, and implementing caching, you can significantly improve your code's performance. Just remember to always measure the impact of your optimizations and consider the trade-offs involved. Happy coding!
The above is the detailed content of Optimize PHP Code: Reducing Memory Usage & Execution Time. For more information, please follow other related articles on the PHP Chinese website!
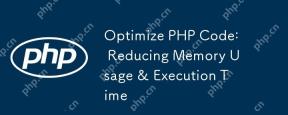
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
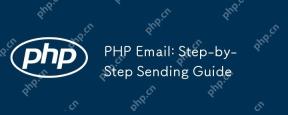
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
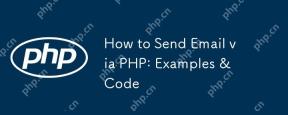
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
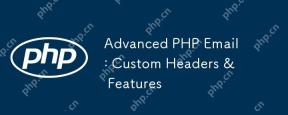
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
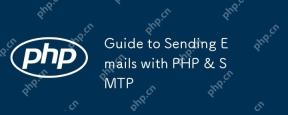
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
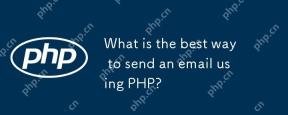
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
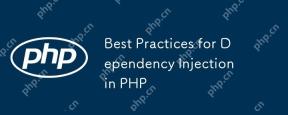
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
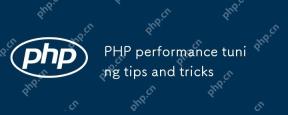
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
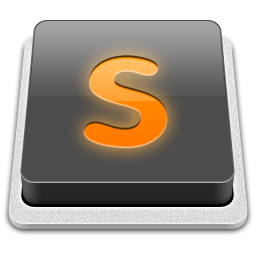
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
