PHP Email Security: Best Practices for Sending Emails
The best practices for sending emails securely in PHP include: 1) Using secure configurations with SMTP and STARTTLS encryption, 2) Validating and sanitizing inputs to prevent injection attacks, 3) Encrypting sensitive data within emails using OpenSSL, 4) Properly handling email headers to avoid manipulation, 5) Logging email sending attempts for tracking issues and security breaches, and 6) Implementing asynchronous email sending for improved performance.
In the ever-evolving landscape of web development, ensuring the security of email communications is not just a best practice—it's a necessity. When it comes to PHP, a language that powers a significant portion of the web, understanding and implementing secure email practices is crucial. So, what are the best practices for sending emails securely in PHP?
Sending emails securely in PHP involves a blend of technical know-how, understanding common vulnerabilities, and implementing robust security measures. From my experience, the journey to secure email sending isn't just about writing code; it's about understanding the ecosystem of email transmission and the potential pitfalls that await the unwary developer.
Let's dive into the world of PHP email security, where I'll share insights from my own projects and the lessons learned along the way. We'll explore how to set up secure email transmission, validate and sanitize inputs, use encryption, and much more. And yes, we'll look at some code that I've tweaked and tested over time to ensure it's not just functional but secure.
To start, consider the basics of email security in PHP. It's not just about using mail()
or some third-party library. It's about ensuring every aspect of your email sending process is locked down tight. I've seen projects where a simple oversight led to major security breaches, so let's ensure we're covering all bases.
For instance, when I was working on a project for an e-commerce platform, we had to send order confirmations securely. We used PHPMailer, which is a robust library for sending emails, but even then, we had to ensure our configuration was spot-on. Here's a snippet of how we set it up:
<?php use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\Exception; require 'vendor/autoload.php'; $mail = new PHPMailer(true); try { //Server settings $mail->isSMTP(); $mail->Host = 'smtp.example.com'; $mail->SMTPAuth = true; $mail->Username = 'user@example.com'; $mail->Password = 'your-password'; $mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS; $mail->Port = 587; //Recipients $mail->setFrom('from@example.com', 'Mailer'); $mail->addAddress('recipient@example.com', 'Recipient Name'); //Content $mail->isHTML(true); $mail->Subject = 'Order Confirmation'; $mail->Body = 'Your order has been confirmed!'; $mail->AltBody = 'Your order has been confirmed!'; $mail->send(); echo 'Message has been sent'; } catch (Exception $e) { echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}"; } ?>
This code snippet is more than just a setup; it's a testament to the importance of secure configurations. Using SMTP with STARTTLS encryption ensures that the email transmission is encrypted, which is vital for security. But this is just the tip of the iceberg.
In my experience, one of the critical areas to focus on is input validation and sanitization. Emails can be a vector for injection attacks, so ensuring that every piece of data you're sending through an email is sanitized is crucial. I've implemented a simple yet effective function for this:
<?php function sanitizeInput($input) { return htmlspecialchars(strip_tags($input), ENT_QUOTES, 'UTF-8'); } // Usage $email = sanitizeInput($_POST['email']); $name = sanitizeInput($_POST['name']); ?>
This function strips tags and converts special characters to HTML entities, which helps prevent XSS attacks. But remember, this is just one layer of security. You should also validate email addresses using PHP's built-in filter_var()
function:
<?php if (filter_var($email, FILTER_VALIDATE_EMAIL)) { // Email is valid } else { // Email is invalid } ?>
Another aspect I've found essential is the use of encryption for sensitive data. While the email transmission itself can be encrypted with STARTTLS, you might also need to encrypt sensitive data within the email. I've used OpenSSL for this purpose:
<?php function encryptData($data, $key) { $ivlen = openssl_cipher_iv_length($cipher="AES-128-CBC"); $iv = openssl_random_pseudo_bytes($ivlen); $ciphertext_raw = openssl_encrypt($data, $cipher, $key, $options=OPENSSL_RAW_DATA, $iv); $hmac = hash_hmac('sha256', $ciphertext_raw, $key, $as_binary=true); $ciphertext = base64_encode( $iv.$hmac.$ciphertext_raw ); return $ciphertext; } // Usage $encryptedData = encryptData('Sensitive Data', 'your-secret-key'); ?>
This function encrypts data using AES-128-CBC, which is robust enough for most applications. But be mindful of key management; storing keys securely is as important as the encryption itself.
Now, let's talk about some of the pitfalls I've encountered. One common mistake is not properly handling email headers. Headers can be manipulated to inject malicious content, so always ensure you're using the appropriate methods to set headers:
<?php $mail->addCustomHeader('X-Mailer', 'PHP/' . phpversion()); ?>
Another pitfall is not logging email sending attempts. Logging can help you track down issues and potential security breaches:
<?php function logEmail($emailDetails) { $logFile = 'email_log.txt'; $logEntry = date('Y-m-d H:i:s') . ' - ' . json_encode($emailDetails) . "\n"; file_put_contents($logFile, $logEntry, FILE_APPEND); } // Usage logEmail([ 'to' => $mail->getToAddresses(), 'subject' => $mail->Subject, 'status' => $mail->isSent() ? 'Sent' : 'Failed' ]); ?>
In terms of performance and best practices, one thing I've learned is to use asynchronous email sending when possible. This can significantly improve the user experience, especially on high-traffic sites. Here's a simple way to implement this using PHP's exec()
function:
<?php function sendEmailAsync($to, $subject, $body) { $command = "php -q send_email.php '{$to}' '{$subject}' '{$body}' > /dev/null &"; exec($command); } // Usage sendEmailAsync('recipient@example.com', 'Subject', 'Body'); ?>
And in send_email.php
, you would have your email sending logic. This approach offloads the email sending to a separate process, which can be beneficial for performance.
In conclusion, securing email communications in PHP is a multifaceted challenge. It's not just about the code you write but how you manage and think about security at every step. From my own journey, I've learned that vigilance, continuous learning, and a proactive approach to security are the keys to success. Whether you're sending order confirmations, newsletters, or any other type of email, these best practices will help you build a more secure and robust email system in PHP.
The above is the detailed content of PHP Email Security: Best Practices for Sending Emails. For more information, please follow other related articles on the PHP Chinese website!
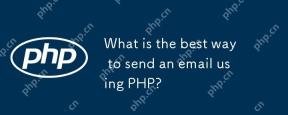
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
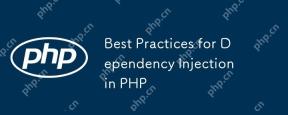
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
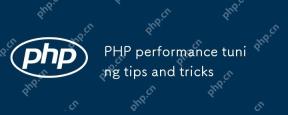
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
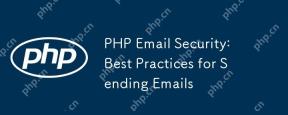
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
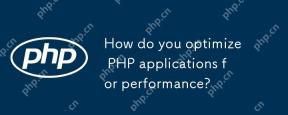
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
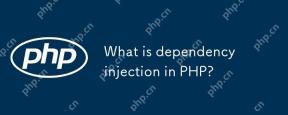
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
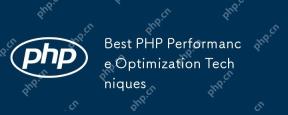
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
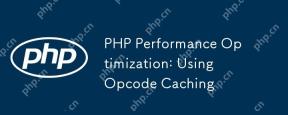
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
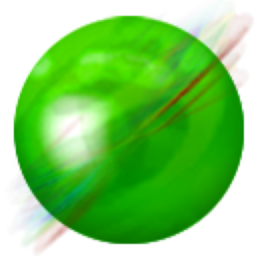
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
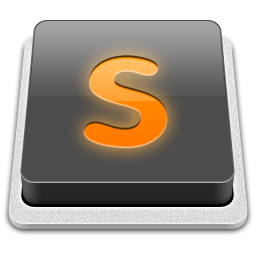
SublimeText3 Mac version
God-level code editing software (SublimeText3)
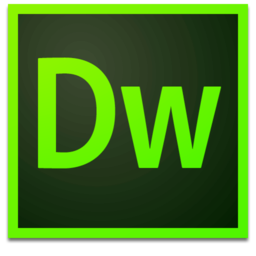
Dreamweaver Mac version
Visual web development tools
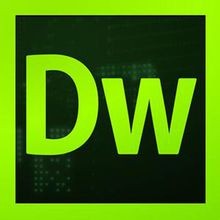
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
