How to use PHP to optimize database performance
How to optimize database performance with PHP
The database is the core component of most web applications, and database performance is crucial to the overall performance and response time of the application important. As a popular server-side scripting language, PHP can optimize database performance through some technical means. This article will introduce some commonly used PHP methods to optimize database performance through specific code examples.
1. Reasonable use of indexes
Indexes are the key to improving database query performance. In database tables, using appropriate indexes can speed up queries and reduce the load on the database. In PHP, indexes can be created by using the "CREATE INDEX" command of the SQL statement. The following is an example:
$sql = "CREATE INDEX index_name ON table_name(column_name)"; $result = mysqli_query($conn, $sql);
When creating an index, you need to select appropriate columns as index columns, and appropriate index types, such as B-tree, Hash, full-text index, etc. Also avoid using too many indexes, as each index adds overhead to the database.
2. Reduce the number of database connections
Every time a connection is established with the database, a certain amount of overhead will be incurred. Therefore, in PHP, it is necessary to reduce the number of database connections as much as possible. A simple and effective method is to use a persistent connection (Persistent Connection). Long connections can maintain the status of the database connection after the script is executed. The connection can be directly reused the next time the script is executed, avoiding the overhead of re-establishing the connection each time. In PHP, you can use the mysqli_pconnect function in the mysqli function to implement long connections. The sample code is as follows:
$conn = mysqli_pconnect('localhost', 'username', 'password', 'database');
3. Use prepared statements
Preprocessed statements can effectively improve the performance of database queries. Through prepared statements, SQL statements and parameters can be separated. Only parameters need to be passed every time it is executed, without re-parsing the SQL statement. This can reduce database load and network transmission overhead.
In PHP, you can use mysqli or PDO extensions to implement prepared statements. The following is an example of using mysqli extension:
$stmt = $conn->prepare("SELECT * FROM table_name WHERE column_name = ?"); $stmt->bind_param("s", $value); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { // 处理查询结果 }
4. Using cache
Using cache can reduce the number of database queries and improve query efficiency. A common caching technology in PHP is to use memory cache, such as using Memcached or Redis. The database query results can be saved in the cache, and the next query can be obtained directly from the cache, avoiding querying and reading operations on the database. The sample code is as follows:
$memcached = new Memcached(); $memcached->addServer('127.0.0.1', 11211); $key = 'cache_key'; $result = $memcached->get($key); if (!$result) { $result = // 从数据库中查询结果 $memcached->set($key, $result, 3600); // 设置缓存有效期为1小时 } // 使用查询结果
5. Reasonable use of transactions
Transactions can ensure the atomicity of a set of database operations and ensure the consistency and integrity of the data. In PHP, this can be achieved by using the transaction functionality of mysqli or PDO extensions. Transactions can effectively reduce database load and lock conflicts.
$conn->begin_transaction(); try { // 执行一组数据库操作 $conn->commit(); } catch (Exception $e) { $conn->rollback(); }
To sum up, through technical means such as reasonable use of indexes, reducing the number of database connections, using prepared statements, using caching and reasonable use of transactions, the database performance of PHP applications can be effectively optimized. Of course, in practical applications, it is necessary to comprehensively consider the specific situation and select an appropriate optimization strategy.
The above is the detailed content of How to use PHP to optimize database performance. For more information, please follow other related articles on the PHP Chinese website!
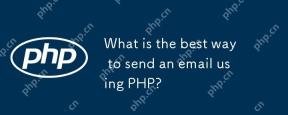
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
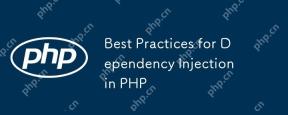
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
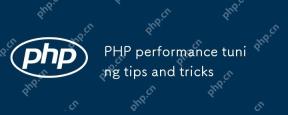
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
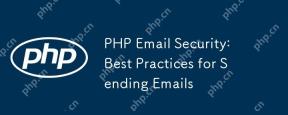
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
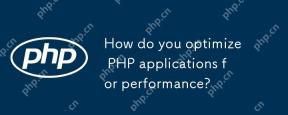
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
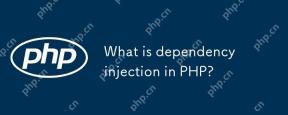
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
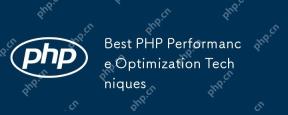
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
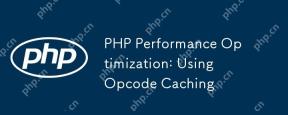
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor
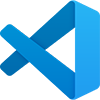
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
