


User authentication and third party login for PHP based live chat system
User authentication and third-party login of real-time chat system based on PHP
Foreword:
In modern social networks, real-time chat system has become a must An indispensable feature. To ensure the security of user data and the accuracy of personal identity, user authentication and third-party login capabilities have also become critical. This article will introduce how to implement user authentication and third-party login functions in a PHP-based real-time chat system, and provide specific code examples.
1. User Authentication
User authentication is a way to ensure user identity. In a live chat system, users must authenticate to access the system's chat functionality. Below is a simple sample code that shows how to implement basic user authentication functionality in PHP.
<?php // 假设已经有一个用户表,包含字段:id、username、password // 这里假设使用的是简单的用户名和密码验证 // 获取用户提交的表单数据 $username = $_POST['username']; $password = $_POST['password']; // 进行数据库查询,检查用户名和密码是否匹配 $query = "SELECT * FROM users WHERE username = '$username' AND password = '$password'"; $result = mysqli_query($connection, $query); // 如果查询结果不为空,则表示用户名和密码匹配成功 if (mysqli_num_rows($result) > 0) { // 用户身份验证成功,可以进一步处理逻辑,例如保存用户登录状态等 // 例如:$_SESSION['user'] = $username; echo "身份验证成功!"; } else { // 用户身份验证失败,可以返回错误信息或重新登录 echo "用户名或密码错误,请重新登录!"; } ?>
2. Third-party login
In order to provide a more convenient login method, many real-time chat systems also support third-party login, such as logging in with Google, Facebook or WeChat accounts. Below is a simple sample code that shows how to implement third-party login functionality in PHP.
<?php // 假设已经通过Google提供的API获取到用户的授权凭证 // 进行Google账户验证 $client = new Google_Client(); $client->setClientId('YOUR_CLIENT_ID'); $client->setClientSecret('YOUR_CLIENT_SECRET'); $client->setRedirectUri('YOUR_REDIRECT_URI'); $client->addScope('email'); // 获取授权码 $code = $_GET['code']; // 获取访问令牌 $token = $client->fetchAccessTokenWithAuthCode($code); // 验证访问令牌 if (!empty($token)) { $payload = $client->verifyIdToken(); // 如果验证成功,则表示用户身份验证通过 if ($payload) { // 用户身份验证成功,可以进一步处理逻辑 // 例如:保存用户登录状态等 echo "用户登录成功!"; } else { // 验证失败,返回错误信息或重新登录 echo "用户登录失败,请重新登录!"; } } else { // 无效的访问令牌,返回错误信息或重新登录 echo "无效的访问令牌,请重新登录!"; } ?>
Summary:
User authentication and third-party login are essential features in a real-time chat system. This article introduces how to implement user authentication and third-party login functions in a PHP-based real-time chat system by giving specific code examples. Readers can make corresponding modifications and extensions according to their actual needs to meet the needs of the system.
The above is the detailed content of User authentication and third party login for PHP based live chat system. For more information, please follow other related articles on the PHP Chinese website!
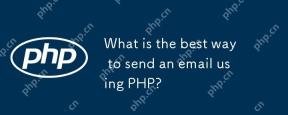
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
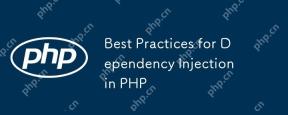
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
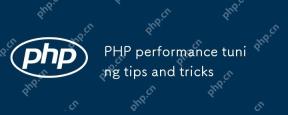
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
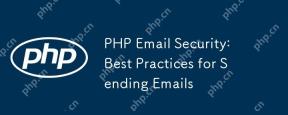
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
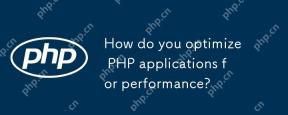
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
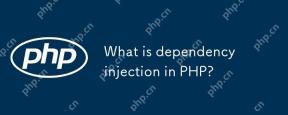
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
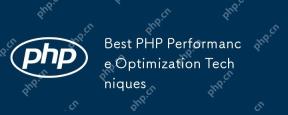
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
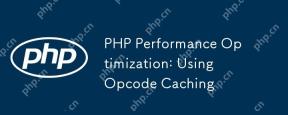
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
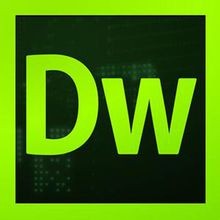
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
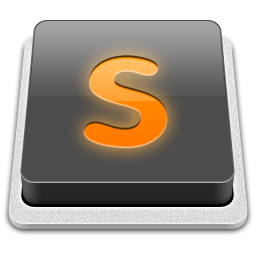
SublimeText3 Mac version
God-level code editing software (SublimeText3)
