


Use PHP and coreseek to implement accurate image-driven search functions
Use PHP and coreseek to achieve accurate image-driven search function
With the rapid development of the Internet, image search function plays an increasingly important role in user experience and information retrieval. The more important the role. This article will introduce how to use PHP and coreseek to implement accurate image-driven search functions to help users quickly find the images they need.
- Installing coreseek
First, we need to install coreseek - an open source search server software developed based on Sphinx. It can be installed through the following command:
$ wget http://sphinxsearch.com/files/sphinx-2.2.11-release.tar.gz $ tar -xzf sphinx-2.2.11-release.tar.gz $ cd sphinx-2.2.11-release $ ./configure --prefix=/usr/local/coreseek $ make && make install
After installing coreseek, we need to configure indexing and search for it.
- Configuration Index
In the coreseek installation directory, create a folder named/usr/local/coreseek/etc
to store the core configuration files. Create a file namedPictures.conf
under this folder to configure the index for picture search:
source src1 { type = mysql sql_host = localhost sql_user = root sql_pass = your_p@ssword sql_db = your_database_name sql_port = 3306 } index img_index { type = rt rt_mem_limit = 1024M path = /usr/local/coreseek/var/data/img_index morphology = stem_en min_word_len = 1 charset_dictpath = /usr/local/coreseek/var/data/dict charset_type = zh_cn.utf-8 charset_table = 0..9, A..Z->a..z, _, a..z, U+410..U+42F->U+430..U+44F, U+430..U+44F rt_field = description rt_attr_uint = categoryId rt_attr_uint = uploaderId rt_attr_timestamp = uploadTime } indexer { mem_limit = 256M } searchd { listen = 9312 log = /usr/local/coreseek/var/log/searchd.log query_log = /usr/local/coreseek/var/log/query.log read_timeout = 5 max_children = 30 pid_file = /usr/local/coreseek/var/log/searchd.pid max_matches = 1000 }
in the Pictures.conf
file , we set the data source to be the MySQL database, the user is root, the password is your_p@ssword, and the database is your_database_name. At the same time, we configured parameters such as index name, index file storage path, and word separator.
- Create PHP script
Next, we will write a PHP script to implement the image search function. Create a file calledimage_search.php
and add the following code:
<?php require_once('sphinxapi.php'); $sphinx = new SphinxClient(); $sphinx->SetServer('localhost', 9312); $sphinx->SetConnectTimeout(3); $sphinx->SetArrayResult(true); $keyword = $_GET['keyword']; // 获取用户输入的关键词 $sphinx->SetMatchMode(SPH_MATCH_EXTENDED); // 使用扩展模式 $sphinx->SetSortMode(SPH_SORT_ATTR_ASC, 'uploadTime'); // 根据上传时间排序 $sphinx->SetLimits(0, 10); // 每页显示10条结果 $queryResult = $sphinx->Query($keyword, 'img_index'); // 查询结果 $ids = array(); if ($queryResult && isset($queryResult['matches'])) { foreach ($queryResult['matches'] as $match) { $ids[] = $match['id']; } } // 根据搜索结果获取对应的图片信息,并进行展示 if (!empty($ids)) { $db = new PDO("mysql:host=localhost;dbname=your_database_name", "root", "your_p@ssword"); $stmt = $db->prepare("SELECT * FROM images WHERE id IN (".implode(',', $ids).")"); $stmt->execute(); $images = $stmt->fetchAll(PDO::FETCH_ASSOC); foreach ($images as $image) { echo '<img src="/static/imghwm/default1.png" data-src="'.$image['url'].'" class="lazy".$image['url'].'" alt="'.$image['description'].'">'; } } else { echo '没有找到相关图片'; }
In the above code, we have used Sphinx’s PHP client library sphinxapi.php
, and called relevant search and query methods in the script. The keywords entered by the user are obtained through GET, and then searched using Sphinx's Query method. Finally, the corresponding images are displayed on the web page based on the search results.
- Run and test
Save the above code to the web root directory of the server and access it in the browserhttp://localhost/image_search.php?keyword=xxx
, wherekeyword
is the keyword entered by the user. If everything is configured correctly, you can see the images that meet the conditions displayed on the page.
Through the above simple steps, we have successfully implemented the precise image-driven search function using PHP and coreseek. Users can easily enter keywords and quickly find the pictures they need. At the same time, through the core configuration files and code examples, you can further optimize and expand according to actual needs to meet business needs.
The above is the detailed content of Use PHP and coreseek to implement accurate image-driven search functions. For more information, please follow other related articles on the PHP Chinese website!
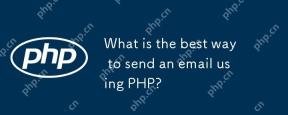
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
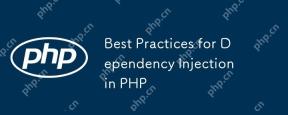
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
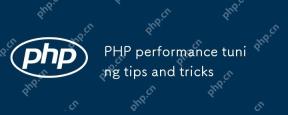
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
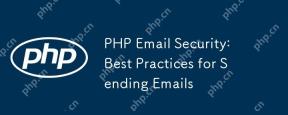
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
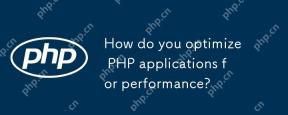
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
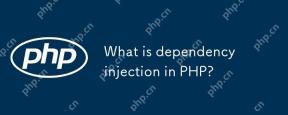
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
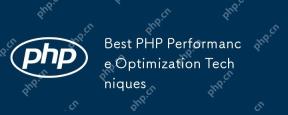
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
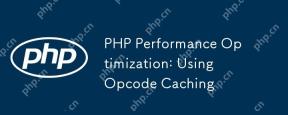
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
