php Method to merge two arrays: 1. Use the " " operator to directly merge two arrays into one array; 2. Use the "array_merge()" function to merge one or more arrays into an array; 3. Use the "array_merge_recursive()" function to merge one or more arrays into one array; 4. Use the "array_combine()" function to merge two arrays to create a new array.
The operating environment of this tutorial: Windows 10 system, php8.1.3 version, DELL G3 computer.
I believe that in your daily development of PHP, you will often encounter scenarios where you need to merge arrays. So, what methods can be used to merge arrays in php?
The first method is to use the " " operator
Using the plus " " operator, you can directly merge two arrays into one array.
Sample code:
<?php $a = [1,2,3,'a'=>'a']; $b = ['a'=>'aa','b'=>'bb',4,5,6,7,'c'=>'cc',8]; $c = $a + $b; var_dump($a); var_dump($b); var_dump($c);
Output result:
// $a array(4) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> string(2) "a" } // $b array(8) { ["a"]=> string(2) "aa" ["b"]=> string(2) "bb" [0]=> int(4) [1]=> int(5) [2]=> int(6) [3]=> int(7) ["c"]=> string(2) "cc" [4]=> int(8) } // $c array(8) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> string(2) "a" ["b"]=> string(2) "bb" [3]=> int(7) ["c"]=> string(2) "cc" [4]=> int(8) }
Note: Use the plus sign " " operator to merge arrays. When the keys of the two array elements are the same, the previous The elements will overwrite the following elements.
Second, use array_merge() function
Usage: array_merge(array1,array2,array3...)
array_merge() function Used to combine one or more arrays into one array.
If two or more array elements have the same key name, the last element will overwrite the other elements.
Sample code:
<?php $a = [1,2,3,'a'=>'a']; $b = ['a'=>'aa','b'=>'bb',4,5,6,7,'c'=>'cc',8]; $c = array_merge($a,$b); $d = []; $e = array_merge($a,$d); $f = array_merge($d,$b); $g = array_merge([],[]); var_dump($a); var_dump($b); var_dump($c); var_dump($e); var_dump($f); var_dump($g);
Output result:
// $a array(4) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> string(1) "a" } // $b array(8) { ["a"]=> string(2) "aa" ["b"]=> string(2) "bb" [0]=> int(4) [1]=> int(5) [2]=> int(6) [3]=> int(7) ["c"]=> string(2) "cc" [4]=> int(8) } // $c array(11) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> string(2) "aa" ["b"]=> string(2) "bb" [3]=> int(4) [4]=> int(5) [5]=> int(6) [6]=> int(7) ["c"]=> string(2) "cc" [7]=> int(8) } // $e array(4) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> string(1) "a" } // $f array(8) { ["a"]=> string(2) "aa" ["b"]=> string(2) "bb" [0]=> int(4) [1]=> int(5) [2]=> int(6) [3]=> int(7) ["c"]=> string(2) "cc" [4]=> int(8) } // $g array(0) { }
Note: When the element keys of the two arrays are the same, the later elements will overwrite the previous elements. But if the index of the array is a numeric index or a numeric string index, the numeric index of the merged array will be reset in order, and the first numeric index element of the first array will be filled in sequence starting from 0.
The third method is to use array_merge_recursive() function
Usage: array_merge_recursive(array1,array2,array3...)
array_merge_recursive() function Used to combine one or more arrays into one array.
Sample code:
<?php $a = [1,2,3,'a'=>'a']; $b = ['a'=>'aa','b'=>'bb',4,5,6,7,'c'=>'cc',8]; $c = array_merge_recursive($a,$b); var_dump($a); var_dump($b); var_dump($c);
Output result:
// $a array(4) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> string(1) "a" } // $b array(8) { ["a"]=> string(2) "aa" ["b"]=> string(2) "bb" [0]=> int(4) [1]=> int(5) [2]=> int(6) [3]=> int(7) ["c"]=> string(2) "cc" [4]=> int(8) } // $c array(11) { [0]=> int(1) [1]=> int(2) [2]=> int(3) ["a"]=> array(2) { [0]=> string(1) "a" [1]=> string(2) "aa" } ["b"]=> string(2) "bb" [3]=> int(4) [4]=> int(5) [5]=> int(6) [6]=> int(7) ["c"]=> string(2) "cc" [7]=> int(8) }
Note: If two or more array elements have the same key, array_merge_recursive() will not perform keying Name overwriting, instead, multiple identical key names are recursively formed into an array. If the index of the array is a numeric index or a numeric string index, the numeric index of the merged array will be reset in order, starting from the first numeric index element of the first array and filling it in sequence from 0.
The fourth method is to use the array_combine() function
Usage: array_combine(keys, values)
array_combine() function, by merging two array to create a new array in which one array element is the key name and the other array element is the key value.
Sample code:
<?php $a = [1,2,3]; $b = ['a','b','c']; $c = array_combine($a,$b); $d = array_combine($b,$a); var_dump($c); var_dump($d);
Output result:
// $c array(3) { [1]=> string(1) "a" [2]=> string(1) "b" [3]=> string(1) "c" } // $d array(3) { ["a"]=> int(1) ["b"]=> int(2) ["c"]=> int(3) }
Note: The number of elements in the key name array and the key value array must be the same!
The above is the detailed content of How to merge two arrays in php. For more information, please follow other related articles on the PHP Chinese website!
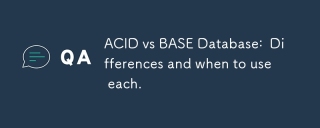
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
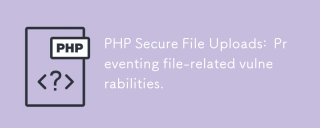
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
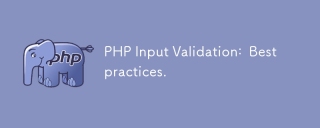
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
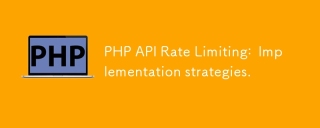
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
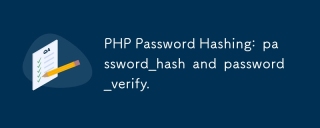
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
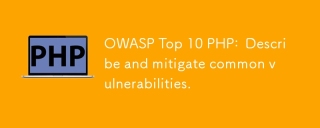
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
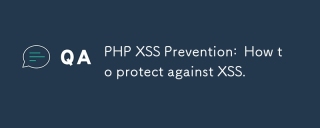
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
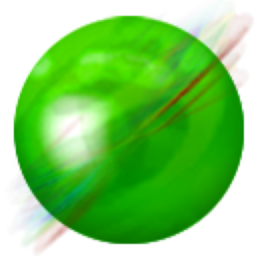
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
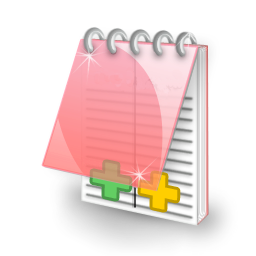
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor