PHP is a widely used server-side scripting language commonly used to develop web applications. In PHP, array is a very common data type that can be used to store large amounts of data, such as text, numbers, and objects. However, sometimes, we need to remove specific elements from the array. This article will introduce how to delete specified elements in a PHP array.
Use the unset() function to delete specified array elements
PHP has a built-in function called unset(), which can be used to delete specified variables. In PHP, an array element is actually just a variable, so we can use the unset() function to delete a specific array element.
Here is a simple example showing how to delete elements from a PHP array:
<?php $fruits = array('apple', 'banana', 'cherry', 'dates', 'elderberry'); // 删除第2个元素 unset($fruits[1]); // 打印数组 print_r($fruits); ?>
In this example, we have created an array called $fruits that contains 5 elements . We used the unset() function to delete the 2nd element (i.e. "banana"). Finally, we printed the array using the print_r() function so we can view the results.
You can run this example in the console or browser to see its output. You will find that the second element in the array has been deleted, and the printed result only contains 4 elements (ie "apple", "cherry", "dates" and "elderberry").
Use the array_diff() function to delete multiple specified elements
If you want to delete multiple specified array elements, you can use the PHP built-in function array_diff(). This function finds the difference between two arrays, i.e. returns only the elements that are present in the first array.
Here is a simple example showing how to use the array_diff() function to delete multiple elements in a PHP array:
<?php $fruits = array('apple', 'banana', 'cherry', 'dates', 'elderberry'); // 要删除的元素 $to_remove = array('banana', 'dates'); // 使用array_diff()函数删除指定元素 $fruits = array_diff($fruits, $to_remove); // 打印数组 print_r($fruits); ?>
In this example, we first create an object called $fruits An array containing 5 elements. We then create another array, $to_remove, which contains the elements we want to remove (i.e. "banana" and "dates"). Finally, we use the array_diff() function to remove these elements and assign the result to the $fruits array. Finally, we print the result using the print_r() function again so we can see the elements in the array.
You can run this example in the console or browser to see its output. You will find that the "banana" and "dates" elements in the array have been deleted, and only 3 elements (i.e. "apple", "cherry" and "elderberry") are left in the printed result.
Use the array_splice() function to delete the specified element and keep the array key value
If you want to delete the specified element in the array and keep the key values of other elements unchanged, you need to use array_splice ()function.
Here is a simple example that shows how to use the array_splice() function to delete specified elements in a PHP array and keep the key value:
<?php $fruits = array('a' => 'apple', 'b' => 'banana', 'c' => 'cherry', 'd' => 'dates', 'e' => 'elderberry'); // 删除b,c两个元素 $removed = array_splice($fruits, 1, 2); // 打印原数组和删除的元素 print_r($fruits); print_r($removed); ?>
In this example, we create an array named $fruits array, which contains 5 elements, each element has a key name. We used the array_splice() function to delete the two elements with keys "b" and "c". This function returns a new array containing the deleted elements (i.e. "banana" and "cherry"). Finally, we use the print_r() function to print the array and the deleted elements.
You can run this example in the console or browser to see its output. You will find that the two specified elements in the array have been deleted, and only 3 elements (ie "a", "d" and "e") are left in the printed result. At the same time, we can see that the deleted elements are returned in a new array.
Conclusion
In PHP, various methods can be used to delete specified array elements. You can use the unset() function to delete one element, the array_diff() function to delete multiple elements, or the array_splice() function to delete a specified element and keep the keys of other elements unchanged. You can choose a method that suits you based on your specific needs to delete specified elements from the array.
The above is the detailed content of php delete specified array. For more information, please follow other related articles on the PHP Chinese website!
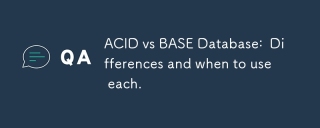
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
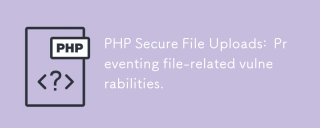
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
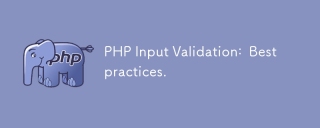
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
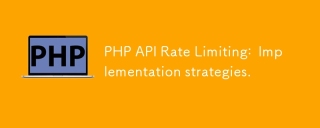
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
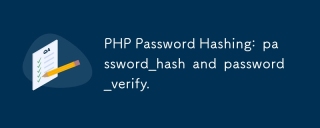
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
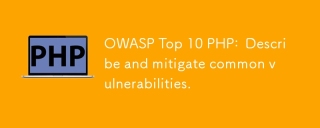
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
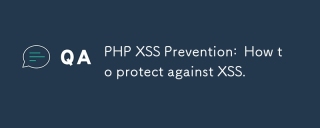
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
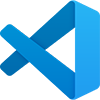
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
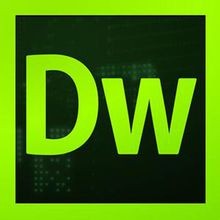
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.