PHP is a widely used open source scripting language. It is mainly used for web development and can quickly create dynamic web pages. PHP has many built-in functions, including functions for summing numbers in an array. In this article, we will explain how to sum numbers in an array using PHP.
First, we need to define an array containing numbers. For example, the following is a PHP array containing several numbers:
$numbers = array(2, 4, 6, 8, 10);
To request the sum of all numbers in this array, we can use the built-in function array_sum(). The function of array_sum() function is to calculate the sum of all values in the array. The following is a sample code for summing using the array_sum() function:
$sum = array_sum($numbers); echo "数组元素的总和为:" . $sum;
Add the above code to your PHP file and run it to get the output:
数组元素的总和为:30
In this example, We pass the $numbers array to the array_sum() function. The function returns the sum of all numbers in the array and assigns it to the variable $sum. Finally, use the echo statement to display the value of the variable $sum.
Of course, this is just a simple example. In actual development, you may need to perform calculation operations on multiple numeric arrays, or use conditional calculations to determine which elements in the array need to participate in the calculation. Here are some additional examples to help you better understand how to sum numbers in an array using PHP.
- For a randomly generated array of numbers, we can calculate the average of all elements in it and echo the result.
$numbers = array_rand(range(1, 100), 10); $average = array_sum($numbers) / count($numbers); echo "随机生成的数字数组为:"; print_r($numbers); echo "平均值为:" . $average;
In this example, we use the array_rand() function to generate 10 random numbers from the range of 1 to 100 and assign them to the $numbers array. We then calculate the sum of all the numbers in the array and divide it by the total number of array elements to get the average. Finally, use the print_r() function to display the randomly generated array of numbers, and use the echo statement to output the average.
- For a given array of numbers, we can ignore the negative numbers in it and calculate the sum of the remaining numbers.
$numbers = array(2, -4, 6, 8, -10, 12); $positive_numbers = array_filter($numbers, function($x) { return $x >= 0; }); $sum = array_sum($positive_numbers); echo "给定数组为:"; print_r($numbers); echo "正数元素的总和为:" . $sum;
In this example, we have defined an array of numbers containing positive and negative numbers and removed all negative numbers from it using the array_filter() function. The array_filter() function tests each element in the array using the specified callback function and returns an array containing only elements that satisfy the test. In this example, we use an anonymous function to test whether an array element is greater than or equal to zero. Finally, we use the array_sum() function to calculate the sum of all positive numbers and use the print_r() function to display the original array of numbers.
In PHP, summing numbers in an array is a common need and can be easily done using the array_sum() function. No matter how many numbers you need to sum, the array_sum() function is a fast, flexible and reusable solution. Don't forget to use this to optimize your code in your next PHP project!
The above is the detailed content of php sum array numbers. For more information, please follow other related articles on the PHP Chinese website!
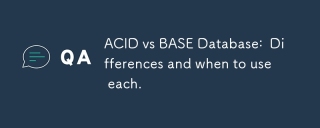
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
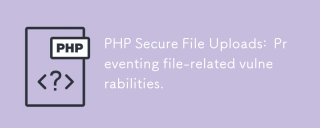
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
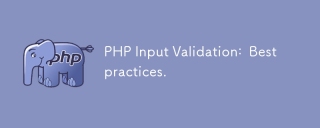
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
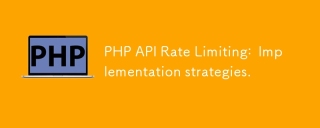
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
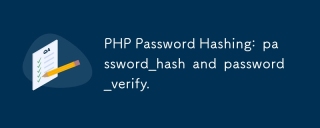
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
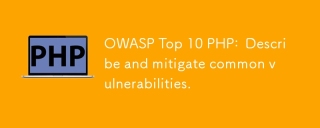
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
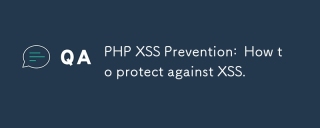
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
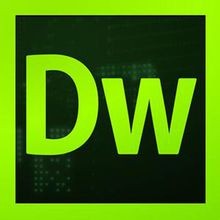
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool