In PHP, a two-dimensional array is a common data structure. It consists of multiple one-dimensional arrays, and each one-dimensional array can contain multiple elements. In some cases, we need to split a two-dimensional array into multiple one-dimensional arrays for more flexible operations and processing. This article will explain how to divide a two-dimensional array into a one-dimensional array.
1. Use foreach loop
In PHP, we can traverse a two-dimensional array by using a foreach loop, and then obtain the corresponding one-dimensional array through the array index. The following is an example of using a foreach loop to split a two-dimensional array into one-dimensional array:
$twoDimensionalArray = array( array('A', 'B', 'C'), array('D', 'E', 'F'), array('G', 'H', 'I') ); $oneDimensionalArray1 = array(); $oneDimensionalArray2 = array(); $oneDimensionalArray3 = array(); foreach ($twoDimensionalArray as $key => $value) { $oneDimensionalArray1[] = $value[0]; $oneDimensionalArray2[] = $value[1]; $oneDimensionalArray3[] = $value[2]; } print_r($oneDimensionalArray1); print_r($oneDimensionalArray2); print_r($oneDimensionalArray3);
In this example, we use a foreach loop to iterate over the two-dimensional array $twoDimensionalArray and get each one-dimensional array by array index Elements. Then, we divide the two-dimensional array into three one-dimensional arrays $oneDimensionalArray1, $oneDimensionalArray2, and $oneDimensionalArray3 by adding the element values to the corresponding one-dimensional arrays.
2. Use the array_column function
After PHP5.5, we can separate the columns of the two-dimensional array into a one-dimensional array by using the array_column function. The following is an example of using the array_column function to separate a two-dimensional array into a one-dimensional array:
$twoDimensionalArray = array( array('id' => 1, 'name' => 'apple', 'price' => 3.5), array('id' => 2, 'name' => 'banana', 'price' => 2.5), array('id' => 3, 'name' => 'orange', 'price' => 4.0) ); $idArray = array_column($twoDimensionalArray, 'id'); $nameArray = array_column($twoDimensionalArray, 'name'); $priceArray = array_column($twoDimensionalArray, 'price'); print_r($idArray); print_r($nameArray); print_r($priceArray);
In this example, we use the array_column function to separate each column of the two-dimensional array $twoDimensionalArray into a one-dimensional array $idArray, $nameArray and $priceArray. Using this function avoids using a foreach loop to traverse the array and is more concise and easier to maintain.
3. Use the array_map function
In PHP, we can also use the array_map function to divide a two-dimensional array into multiple one-dimensional arrays. The following is an example of using the array_map function to divide a two-dimensional array into a one-dimensional array:
$twoDimensionalArray = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); $firstArray = array_map(function($arr) { return $arr[0]; }, $twoDimensionalArray); $secondArray = array_map(function($arr) { return $arr[1]; }, $twoDimensionalArray); $thirdArray = array_map(function($arr) { return $arr[2]; }, $twoDimensionalArray); print_r($firstArray); print_r($secondArray); print_r($thirdArray);
In this example, we use the array_map function to divide the two-dimensional array $twoDimensionalArray into three one-dimensional arrays $firstArray, $secondArray and $thirdArray. We separate the elements of each one-dimensional array in the array and return it as a new one-dimensional array by using an anonymous function.
Through the above three methods, we can divide the two-dimensional array into multiple one-dimensional arrays to facilitate better manipulation and processing of data. Using these methods can reduce the amount of code, improve the readability and maintainability of the code, and also make the program performance more efficient.
The above is the detailed content of How to divide 2D array into 1D array php. For more information, please follow other related articles on the PHP Chinese website!
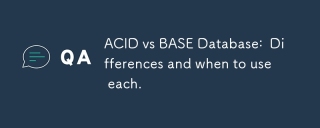
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
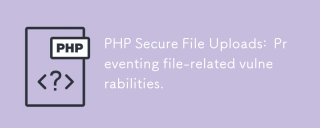
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
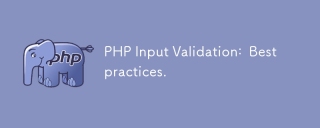
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
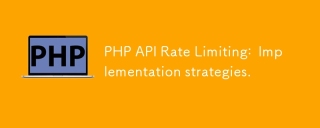
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
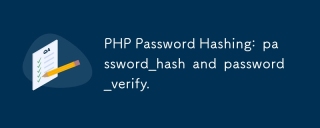
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
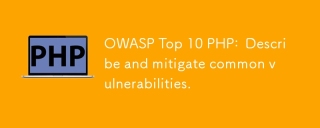
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
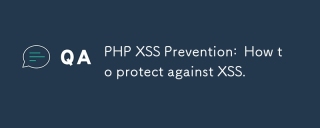
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.