php implements login database
In modern web applications, user authentication is extremely important. A common authentication method is for the user to enter a username and password. After receiving the request, the server will verify whether the information entered by the user is correct. Only after successful verification can the relevant information be successfully accessed. During this process, both parties need to cooperate to achieve normal user authentication. Among them, using a database to store user information on the server side is currently one of the most common implementation methods.
So how to use PHP to implement database user login? This article will explain this process for you.
First of all, we need to make it clear that the user authentication process usually includes the following steps:
- The user visits the login page and enters the username and password
- The server receives the user name and password entered by the user, and checks whether the user exists in the database
- If the user exists, verify whether the password is correct
- If the password is correct, return success information to the user , otherwise a failure message is returned.
Below we will introduce step by step how to implement the process of logging into the database.
Step 1: Create a database
Before we start to implement the login function, we need to create a database first. In this article, we use the MySQL database as an example. We first need to install MySQL, then log in to MySQL and create a database named "test".
CREATE DATABASE test;
After the creation is completed, we need to create a user table. In this user table, we need to store the user's username and password.
CREATE TABLE users (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(30) NOT NULL,
password VARCHAR(30) NOT NULL
);
Step 2: Connect to the database
Before connecting to the database, we need to configure the relevant connection information first. This information includes the user name, password, host name, port, etc. of the database. We can save this configuration information in a separate file and then include this file wherever we need to use it.
Configuration file, such as config.php:
$servername = "localhost";
$username = "root";
$password = "123456";
$dbname = "test";
?>
Next we need to connect to the database and select the database we need to use. This process usually requires the use of the mysqli object in PHP.
include('config.php');
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname) ;
//Detect connection
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
?>
Step3: Implement user login
Next we need to implement the user login function. In this example, we need to get the username and password from the form entered by the user. Then pass the username to the database and query whether the user exists. If the user exists, verify that the password is correct. If the password is correct, success information is returned to the user, otherwise failure information is returned.
First, we need to create a simple HTML form to obtain the username and password entered by the user.
The form above combines the username and The password is submitted to the login.php page.
Next, we need to use PHP code to get the username and password entered by the user from the form and pass the username to the database. If the user is found, verify whether the password is correct. If the password is correct, the user information is stored in the session and success information is returned, otherwise failure information is returned.
include('config.php');
// Check whether the form is submitted
if($_SERVER["REQUEST_METHOD"] == "POST") {
// 主动设置编码,防止中文出现乱码 header('Content-type:text/html;charset=utf-8'); $input_username = mysqli_real_escape_string($conn,$_POST['username']); $input_password = mysqli_real_escape_string($conn,$_POST['password']); // SQL语句 $sql = "SELECT * FROM users WHERE username = '$input_username'"; $result = mysqli_query($conn,$sql); $row = mysqli_fetch_array($result,MYSQLI_ASSOC); $count = mysqli_num_rows($result); // 如果查询到了该用户,检查密码是否正确 if($count == 1) { if(password_verify($input_password, $row['password'])) { // 密码正确,将用户信息存入session中 session_start(); $_SESSION["username"] = $row['username']; $_SESSION["login_time"] = time(); echo '登陆成功'; } else { // 密码错误,返回错误信息 echo '密码错误'; } } else { // 没有查询到该用户,返回错误信息 echo '用户名不存在'; }
}
?>
In the above code, the mysqli_real_escape_string function is used to escape strings to avoid SQL injection vulnerabilities. At the same time, we use the password_verify function to verify whether the password entered by the user is correct. This function will compare whether the entered password and the encrypted password are the same.
Step 4: Security considerations
After completing the above steps, we can implement user login. But in practical applications, we also need to consider some security issues, such as: how to ensure the security of user passwords in the database, how to prevent SQL injection, etc.
In order to ensure the security of the user's password in the database, we need to encrypt it. PHP provides many encryption methods, such as MD5, SHA1, Bcrypt, etc. In this article, we use the password_hash and password_verify functions that come with PHP, which allow us to encrypt and decrypt passwords more conveniently while ensuring their security.
At the same time, we also need to consider how to prevent SQL injection vulnerabilities. In the above code, we use the mysqli_real_escape_string function to avoid SQL injection vulnerabilities. At the same time, we can also use PHP PDO to implement database operations. PDO provides a more secure and reliable way to effectively prevent SQL injection attacks.
In this article, we introduce how to use PHP to implement database user login, which includes the process of connecting to the database, obtaining user input, and querying the database. Through the introduction of this article, I believe everyone can understand more clearly how to implement the user login function in PHP, and at the same time be able to notice the security issues involved.
The above is the detailed content of PHP implements login database. For more information, please follow other related articles on the PHP Chinese website!
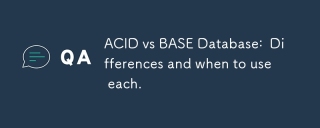
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
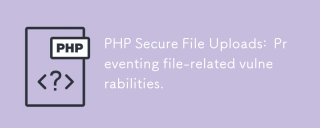
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
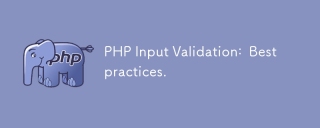
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
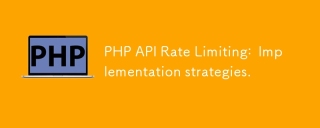
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
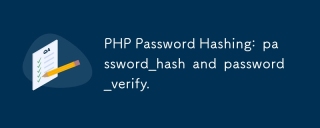
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
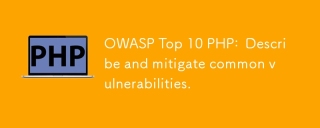
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
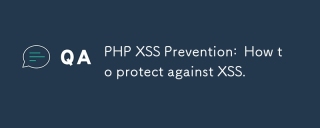
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
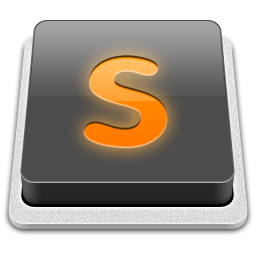
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor