PHP is a well-known and popular server-side programming language. It is very powerful and allows developers to quickly develop Web applications. Therefore, PHP is crucial for web developers. Among them, querying and sorting are one of the most important functions in PHP. They can make data more orderly and easier to manage. In this article, we will explore how to query and sort a database using PHP.
1. Establish a database connection
Before querying and sorting data, you need to establish a connection with the database and provide necessary information, such as user name, password, etc. In PHP, to establish a connection, we need to use the mysqli_connect() function.
For example:
`
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = “mydb”;
//Establish a connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
//Check whether the connection is successful
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
`
2. Query the database
After the connection is established, we can start querying the database. For simple queries, we can use the mysqli_query() function. For example, if we take the "users" table as an example, we can use the following statement to query the information of all users:
`
$query = "SELECT * FROM users";
$result = mysqli_query( $conn, $query);
`
This will return the following results:
` | |||
---|---|---|---|
name | phone | ||
John Doe | john@example.com | 555-1234 | |
Jane Doe | jane@example .com | 555-5678 | |
Bob Smith | bob@example.com | 555 -9012 |
$query = "SELECT * FROM users ORDER BY name ASC" ;
$result = mysqli_query($conn, $query);
`
name | phone | ||
Jane Doe | jane@example.com | 555-5678 | |
John Doe | john@example.com | 555-1234 | |
Bob Smith | bob@example .com | 555-9012 |
$query = “SELECT * FROM users ORDER BY id DESC”;
$result = mysqli_query($conn, $query);
`
name | phone | ||
Bob Smith | bob@ example.com | 555-9012 | |
Jane Doe | jane@example.com | 555-5678 | |
John Doe | john@example.com | 555-1234 |
$servername = "localhost ”;
$username = “username”;
$password = “password”;
$dbname = “mydb”;
$conn = mysqli_connect( $servername, $username, $password, $dbname);
if (!$conn) {
die("连接失败: " . mysqli_connect_error());}/ / Query and sort data
$query = “SELECT * FROM users ORDER BY name ASC”;
$result = mysqli_query($conn, $query);
if (mysqli_num_rows($result) > 0) {
while($row = mysqli_fetch_assoc($result)) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. " - Email: " . $row["email"]. " - Phone: " . $row["phone"]. "<br>"; }} else {
echo "0 结果";}mysqli_close($conn);
`
id: 2 - Name: Jane Doe - Email: jane@example.com - Phone: 555-5678
id: 1 - Name: John Doe - Email: john@example.com - Phone: 555-1234
id: 3 - Name: Bob Smith - Email: bob@example.com - Phone: 555-9012
`
The above is the detailed content of php query database sorting. For more information, please follow other related articles on the PHP Chinese website!
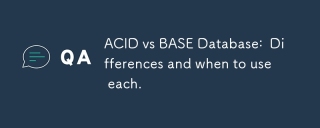
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
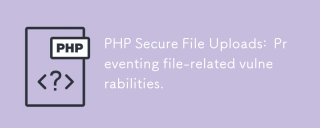
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
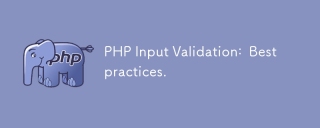
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
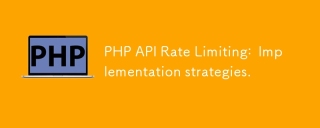
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
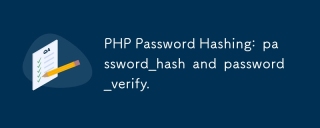
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
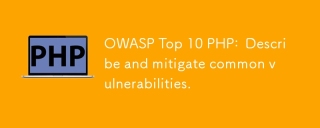
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
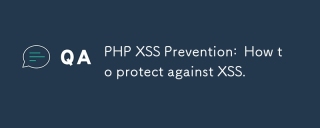
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
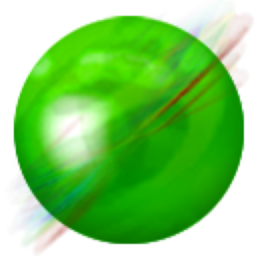
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
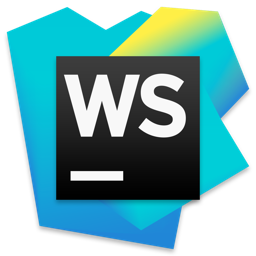
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor