


In PHP, we usually use SQL statements to query data in the database, and the result is often a two-dimensional array, that is, an array containing multiple records. This article will introduce how to use PHP language to query the database and store the results as a two-dimensional array.
1. Establish a database connection
Before querying the database, you first need to establish a database connection. This can be easily accomplished using PHP's built-in mysqli or PDO extensions. The following is a sample code for establishing a connection using mysqli extension:
// 连接数据库 $host = 'localhost'; $user = 'root'; $password = ''; $dbname = 'test'; $conn = new mysqli($host, $user, $password, $dbname); // 检查连接是否成功 if ($conn->connect_errno) { die('连接失败: ' . $conn->connect_error); }
2. Execute SQL query statement
After completing the database connection, we need to execute SQL query statement to obtain data. The query statement can be any legal SQL statement. For example, the SELECT statement can be used to obtain the value of a specific field from the database. The following is an example:
//执行查询并存储结果 $sql = "SELECT id, name, age FROM users"; $result = $conn->query($sql); //检查结果是否为空 if ($result->num_rows > 0) { // 存储结果为二维数组 $data = array(); while ($row = $result->fetch_assoc()) { $data[] = $row; } }
In the above code, we use the mysqli extended query method to execute the SQL query statement. After the query is successful, we use the num_rows method to check whether the result is empty. If the result is not empty, use the fetch_assoc method to store each row of data into an associative array, and add the array to the $data array. Finally, all data is stored in the $data array. So far, we have successfully stored the query results into a two-dimensional array.
3. Use the query results
Once the query results are stored as a two-dimensional array, we can use PHP language to operate on it. For example, you can output each record by looping through the array:
// 遍历数组并输出每条记录 foreach ($data as $row) { echo $row['id'] . "\t" . $row['name'] . "\t" . $row['age'] . "\n"; }
In the above code, we use a foreach loop to traverse the $data array and output the value of the id, name, and age fields of each record, where " \t" represents a tab character, and "\n" represents a newline character.
4. Close the database connection
After using the database, we need to close the database connection to release resources and ensure security. The following is an example:
// 关闭连接 $conn->close();
In the above code, we use the close method of the mysqli extension to close the database connection.
Summary
Querying the database and storing the results as a two-dimensional array in PHP is very simple. You only need to establish a database connection, execute the SQL query statement, store the results as a two-dimensional array, and use the query The result is enough. If you are using other database extensions, such as the PDO extension, it is also very simple to implement similar operations.
The above is the detailed content of How to query a database using PHP and store the results as a 2D array. For more information, please follow other related articles on the PHP Chinese website!
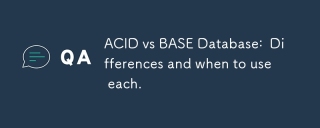
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
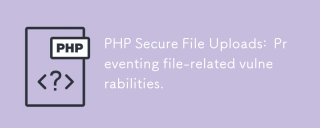
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
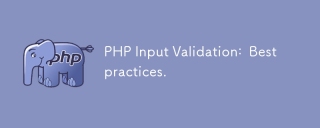
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
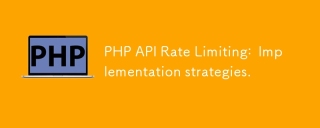
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
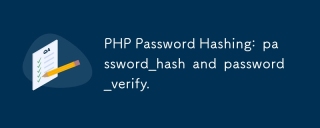
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
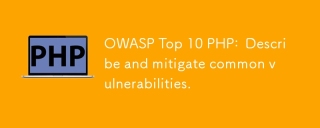
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
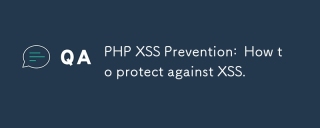
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
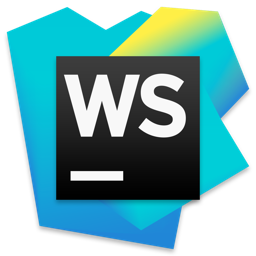
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
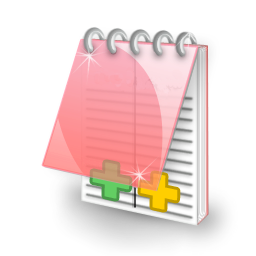
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software