In the process of Web development, PHP language has become one of the most popular programming languages. Among them, the array in PHP is a very common data structure. It is very flexible and adaptable and can be used to store and process various types of data.
However, in development, we often encounter the need to remove unique elements from a very large array. For this problem, PHP arrays provide many different solutions. Let’s introduce them one by one below.
Method 1: Use the array_unique function
The array_unique function is a function that comes with PHP and is used to remove duplicate elements from an array and return an array of non-duplicate elements. Its usage is very simple, as shown below:
$my_array = array(1,2,2,3,3,3,4,4,4,4); $unique_array = array_unique($my_array); print_r($unique_array);
The above code will output the following results:
Array ( [0] => 1 [1] => 2 [3] => 3 [6] => 4 )
As you can see, through the array_unique function, we can easily remove unique items from the original array element.
Method 2: Use array_flip and array_keys functions
If we need to return an array of key names with non-repeating elements, we can use a combination of array_flip and array_keys functions, as shown below:
$my_array = array(1,2,2,3,3,3,4,4,4,4); $unique_keys = array_keys(array_flip($my_array)); print_r($unique_keys);
The above code will output the following results:
Array ( [0] => 0 [1] => 1 [2] => 3 [3] => 6 )
As you can see, through the array_flip and array_keys functions, we get an array of unique element key names.
Method 3: Use loops and in_array function
This is a relatively common approach. We can use a loop to traverse the entire array, and then use the in_array function to check whether the current element already exists in the array. Determine the non-repeating element array. If it does not exist, add it to the non-repeating element array, as shown below:
$my_array = array(1,2,2,3,3,3,4,4,4,4); $unique_array = array(); foreach($my_array as $value){ if(!in_array($value,$unique_array)){ $unique_array[] = $value; } } print_r($unique_array);
The above code will output the following result:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 )
Here we Through the combination of loop and in_array function, unique elements are successfully removed from the original array.
Method 4: Use the array_reduce function
The array_reduce function can be used to iterate over an array and reduce each value in the array to a single value. Therefore, if we want to get an array with non-repeating elements, we can use the array_reduce function to operate. The code is as follows:
$my_array = array(1,2,2,3,3,3,4,4,4,4); $unique_array = array_reduce($my_array,function($result,$value){ if(!in_array($value,$result)){ $result[] = $value; } return $result; }, array()); print_r($unique_array);
The above code will output the following results:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 )
Okay We see that we have achieved the purpose of removing non-repeating elements from the original array by using the combination of array_reduce and in_array functions.
Summary
The above are several methods for removing non-repeating elements from PHP arrays. Although each method has its characteristics and uses, it should be noted that when processing large arrays, the efficiency of each method may be different, and you need to choose and use it according to the situation.
I hope this article will help everyone understand and use PHP arrays.
The above is the detailed content of How to remove unique elements from a php array. For more information, please follow other related articles on the PHP Chinese website!
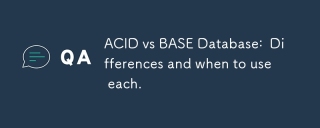
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
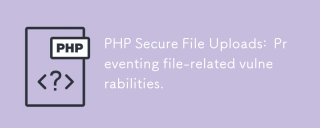
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
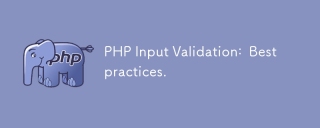
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
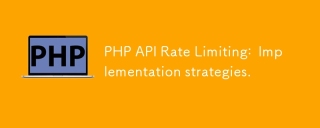
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
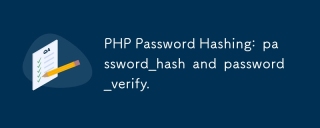
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
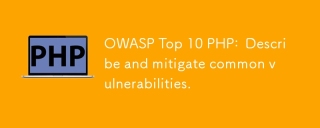
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
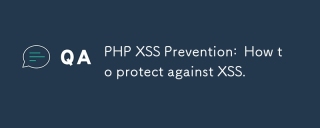
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
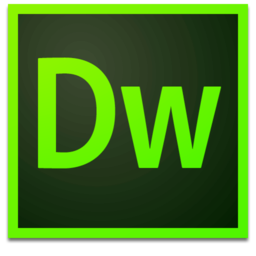
Dreamweaver Mac version
Visual web development tools
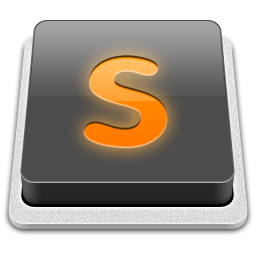
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
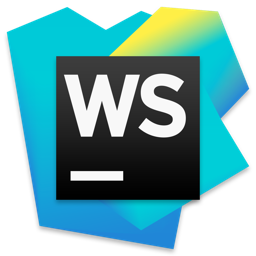
WebStorm Mac version
Useful JavaScript development tools