In many development projects, XML is widely used as configuration files, data transmission, and even document media. In web development, the PHP language also plays an important role, and PHP reading xml and converting it into an array is a basic and necessary skill. This article will describe the process in detail.
1. Reading XML
Before reading XML, we need to obtain an XML file instance. There are many methods, such as getting it from the server, uploading files, writing directly in scripts, etc. Here is an example of getting an XML file from the server:
<?php $xmlFile = 'example.xml'; //设置XML文件路径 $xml = simplexml_load_file($xmlFile); //将文件载入到对象中 ?>
Here we use the simplexml_load_file() method, which can load XML content into an object. An object can represent an entire XML document or a single element. The returned object can be used as a simple array.
2. Convert into an array
Before converting the XML file into an array, we need to understand the basic structure of the XML file. There are three basic node types in XML: elements, attributes and text nodes.
The following is an example:
<person> <name>John Smith</name> <age>30</age> <gender>M</gender> <address> <city>New York</city> <street>123 Main St</street> <zipcode>10000</zipcode> </address> </person>
Next, let us take a look at how to convert XML into a PHP array:
<?php function xmlToArray($xml) { $result = []; foreach ($xml->children() as $element) { $elementName = $element->getName(); if(count($element->children()) > 0) { $result[$elementName] = xmlToArray($element); } else { $result[$elementName] = trim((string)$element); } foreach($element->attributes() as $attrName => $attrValue) { $result['attribute'][$attrName] = (string)$attrValue; } } return $result; } ?>
As shown in the above code, we use recursion here function. First, we traverse the XML node, processing its elements and attributes. If the current node has child nodes, the function is called recursively to convert it into a multidimensional array. If the node has no children, use that as the value of the item.
In addition, we can also add an attribute. For each element, we loop through its attributes and add them as array items to the "attribute" array.
3. Using arrays
Now we have converted the XML into a PHP array. Next, we can use the array we just converted to get the values in the document.
<?php $xmlFile = 'example.xml'; //设置XML文件路径 $xml = simplexml_load_file($xmlFile); //将文件载入到对象中 $result = xmlToArray($xml); //转换成数组 echo $result['person']['name']; //输出John Smith echo $result['person']['address']['city']; //输出New York ?>
Using this method, we can process and manage XML documents more conveniently.
4. Summary
This article introduces how to use PHP to read XML files and convert them into multi-dimensional arrays. Although converting XML into PHP arrays may be a bit tedious, it is still a very necessary skill in web development. At the same time, converting a simple XML file into a PHP array is only a basic process. There are many advanced uses of XML, involving DTD (Document Type Definition) and XSLT (XML Stylesheet Language Transformation). I hope that while learning PHP to obtain XML and convert it into an array, you can also understand the basic knowledge of XML and apply it to more complex projects.
The above is the detailed content of How to read xml data in php and convert it into an array. For more information, please follow other related articles on the PHP Chinese website!
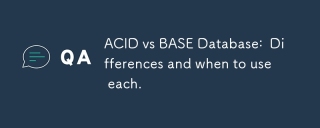
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
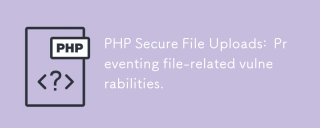
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
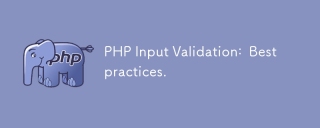
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
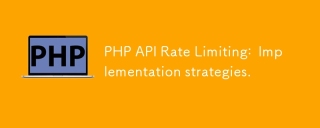
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
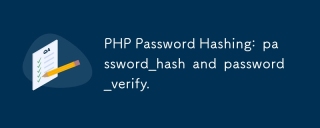
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
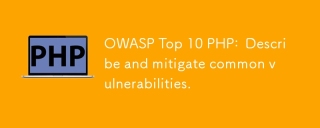
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
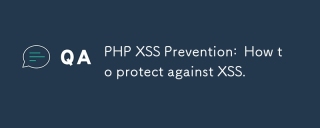
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
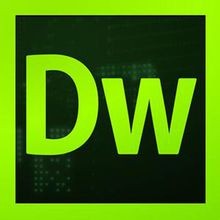
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
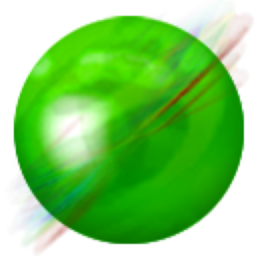
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment