In PHP, if we need to convert an array into an integer, we can use the array_sum() function. This function can return the sum of all elements of the array. If the array contains non-numeric elements, they will be automatically converted to 0.
For example, if we have an array $numbers=array(1,2,'3',4,'five'), we can use the following code to convert it to an integer:
$numbers = array(1,2,'3',4,'five'); $sum = array_sum($numbers); echo $sum;
The output result is: 10. This is because in this array, 1, 2, 3 and 4 are counted as 4 numbers, while the non-numeric element "five" is automatically converted to 0. Therefore, the sum is 10.
If we need to perform the same operation on a multi-dimensional array, we can use a recursive function to achieve it.
For example, if we have the following multidimensional array $arrays:
$arrays = array( array(1,2,3), array('four',5,6), array(7,8,'nine') );
We can use the following code to convert it to an integer:
function recursiveArraySum($array) { $sum = 0; foreach($array as $value) { if(is_array($value)) { $sum += recursiveArraySum($value); } elseif(is_numeric($value)) { $sum += $value; } else { $sum += 0; } } return $sum; } $total = recursiveArraySum($arrays); echo $total;
The output result is: 36. In this multidimensional array, 1, 2, 3, 5, 6, 7, and 8 are all evaluated as numbers, while the non-numeric elements "four" and "nine" are automatically converted to 0.
In short, using the array_sum() function can easily convert an array into an integer. For multi-dimensional arrays, we can use recursive functions to achieve the same operation.
The above is the detailed content of How to convert array to int in php. For more information, please follow other related articles on the PHP Chinese website!
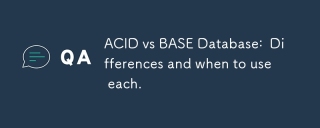
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
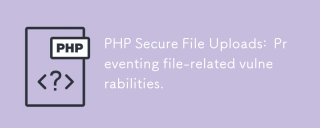
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
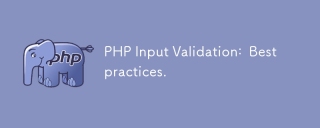
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
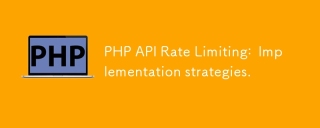
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
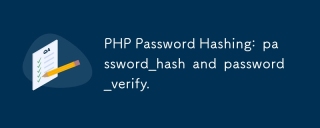
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
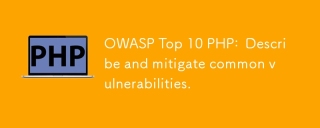
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
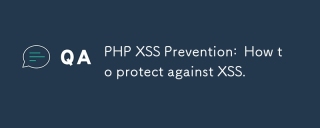
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
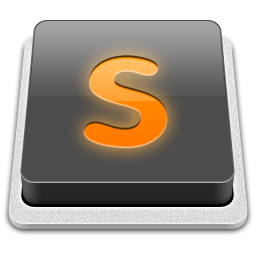
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor