In PHP, array is a very common data type, which can conveniently store a series of data. When using arrays, we often need to obtain an element in the array for subsequent operations. This article will introduce how to obtain array elements in PHP.
1. Basic array access methods
In PHP, you can access elements in an array through the array name and subscript. Array indexes start counting from 0, that is, the first element has an index of 0, the second element has an index of 1, and so on. The syntax for accessing array elements through subscripts is as follows:
$array = array('apple', 'orange', 'banana'); echo $array[0]; // 输出 apple echo $array[1]; // 输出 orange echo $array[2]; // 输出 banana
In the above example, we declare an array $array containing three elements, and then access the elements in the array through subscripts. Of course, you can also use variables or expressions as subscripts:
$i = 1; echo $array[$i]; // 输出 orange echo $array[1 + 1]; // 输出 banana
It should be noted that if you access a non-existent subscript, an error will occur:
echo $array[3]; // Notice: Undefined offset: 3
2. Use Associative array
In addition to using numeric subscripts, you can also use strings as array subscripts. This type of array is called an associative array. Associative arrays make it easy to access elements in an array based on their key values. The syntax of an associative array is as follows:
$array = array( 'name' => 'Tom', 'age' => 18, 'gender' => 'male' ); echo $array['name']; // 输出 Tom echo $array['age']; // 输出 18 echo $array['gender']; // 输出 male
In the above example, we declare an associative array $array containing three elements, and then access the elements in the array through key values. It should be noted that the key values of associative arrays are case-sensitive, so $array['Name'] and $array['name'] are two different elements.
3. Use loops to traverse arrays
In practical applications, we usually need to traverse the entire array and process the elements in it. PHP provides a variety of methods for traversing arrays, the most commonly used of which is the foreach loop. Use a foreach loop to iterate through each element in an array and process it. The syntax of the foreach loop is as follows:
$array = array('apple', 'orange', 'banana'); foreach ($array as $value) { echo $value . '<br>'; }
In the above example, we use the foreach loop to traverse each element in the array $array and output it to the page. It should be noted that the variable $value used in the foreach loop is a temporary variable used to store the current element in the array, and its scope is limited to the inside of the foreach loop.
If you need to get the subscript and value of the array element at the same time in the loop, you can use the following syntax:
$array = array('apple', 'orange', 'banana'); foreach ($array as $key => $value) { echo $key . ' => ' . $value . '<br>'; }
In the above example, we use the foreach loop to traverse the array $array for each element, and output its subscript and value at the same time.
4. Use built-in functions to process arrays
In PHP, many built-in functions are also provided for processing arrays, such as getting the length of the array, adding or removing elements to the array, etc. The following are some commonly used array functions:
- count function: Get the length of the array
The count function is used to get the number of elements in the array. When the parameter passed in is not an array, the function returns 1. The syntax for using the count function is as follows:
$array = array('apple', 'orange', 'banana'); echo count($array); // 输出 3
- array_push and array_pop functions: add or remove elements from the array
array_push function is used to add one or more elements to the end of the array elements, and the array_pop function is used to delete elements at the end of the array. The syntax for using these two functions is as follows:
$array = array('apple', 'orange'); array_push($array, 'banana'); echo count($array); // 输出 3 $fruit = array_pop($array); echo $fruit; // 输出 banana
In the above example, we use the array_push function to add an element to the array $array, and then use the count function to get the new array length; then use array_pop The function deletes the last element of the array $array and assigns it to the $fruit variable.
- array_slice function: intercept a part of the array
array_slice function is used to intercept a part of the array and return a new array. The syntax of this function is as follows:
$array = array('apple', 'orange', 'banana', 'pear', 'watermelon'); $newArray = array_slice($array, 1, 3); print_r($newArray); // 输出: // Array // ( // [0] => orange // [1] => banana // [2] => pear // )
In the above example, we use the array_slice function to intercept the 2nd to 4th elements of the array $array (that is, the elements with subscripts 1 to 3), and The result is saved in the variable $newArray.
Summary
The above is the method of obtaining array elements in PHP. Whether using numeric subscripts or associative array keys, PHP provides a simple and easy-to-use syntax for accessing elements in an array. In addition, PHP also provides a wealth of built-in functions to process arrays, making it easier for us to operate arrays. Proficiency in using arrays is crucial for PHP development.
The above is the detailed content of How to get array elements in php. For more information, please follow other related articles on the PHP Chinese website!
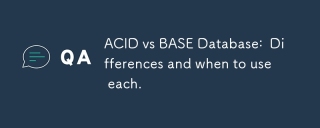
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
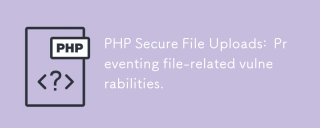
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
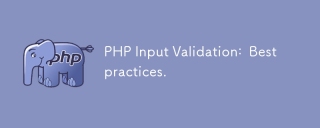
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
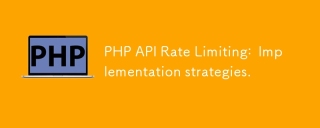
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
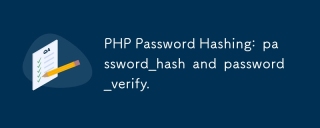
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
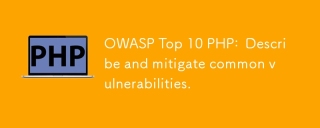
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
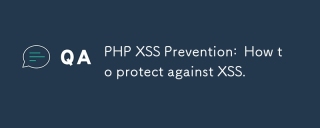
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
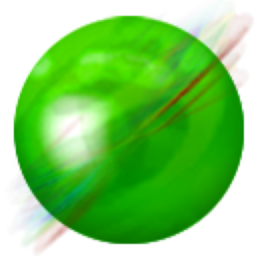
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
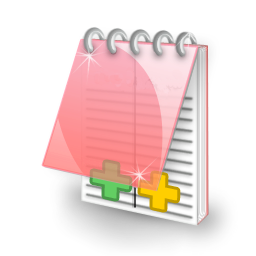
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor