PHP is a powerful open source scripting language used for web development. Among them, array is one of the most commonly used data structures in PHP. In actual development, it is often necessary to merge two or more arrays together. At this time, we often need to keep the key name unchanged to facilitate subsequent operations. This article will explain how to keep key names unchanged when merging arrays in PHP.
The functions for merging arrays in PHP include array_merge() and array_merge_recursive(). array_merge() merges arrays together and returns the merged array. array_merge_recursive() merges two elements with the same key name into an array and returns the merged array. Both functions can merge arrays, but they differ in how they handle elements with the same key.
For example, we have two arrays $a and $b:
$a = array('apple'=>'red', 'banana'=>'yellow', 'orange'=>'orange'); $b = array('apple'=>'green', 'pear'=>'yellow', 'grape'=>'purple');
If we use the array_merge() function to merge these two arrays, the code is as follows:
$c = array_merge($a, $b);
Then, the final result of the merged array $c will be:
array('apple'=>'green', 'banana'=>'yellow', 'orange'=>'orange', 'pear'=>'yellow', 'grape'=>'purple');
As you can see, since only the key-value pairs in the subsequent array are retained after the merge, the key name in $a The element for apple is overwritten by the element with the same key name in $b.
If we use the array_merge_recursive() function to merge the two arrays, the code is as follows:
$c = array_merge_recursive($a, $b);
Then, the final result of the merged array $c will be:
array('apple'=>array('red', 'green'), 'banana'=>'yellow', 'orange'=>'orange', 'pear'=>'yellow', 'grape'=>'purple');
You can see that since elements with the same key name are merged into an array after merging, the element with the key name apple is retained.
However, although the array_merge_recursive() function can keep the key names unchanged, in some cases, we do not want to merge elements with the same key names into an array. For example, if we need to merge two arrays into the same array, and the two arrays contain the same key name, but their values are different, then we need to keep the key name unchanged to facilitate subsequent operations.
So, how to keep the key names unchanged when merging arrays in PHP? A simple and practical way is to use the " " operator. For example, we still use the two arrays $a and $b above, and the code is as follows:
$c = $a + $b;
Then, the final result of the merged array $c will be:
array('apple'=>'red', 'banana'=>'yellow', 'orange'=>'orange', 'pear'=>'yellow', 'grape'=>'purple');
It can be seen that because the " " operator is used, the element with the key name apple in $a is not replaced by the element with the same name in $b, but is retained. In this way, we successfully merged the two arrays and kept their key names unchanged.
Of course, the above method only applies to purely associative arrays. For numeric index arrays, even if the " " operator is used, the key names will change. Therefore, when dealing with numerically indexed arrays, other methods are needed to keep the key names unchanged.
In short, when merging arrays in PHP, you should choose the appropriate function or operator according to different needs to ensure the correctness and efficiency of the operation. When dealing with associative arrays, you can use the " " operator to merge the arrays and keep the key names unchanged.
The above is the detailed content of How to keep key names the same when merging arrays in PHP. For more information, please follow other related articles on the PHP Chinese website!
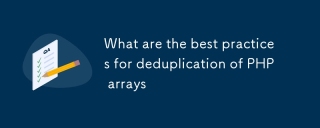
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
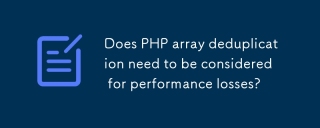
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
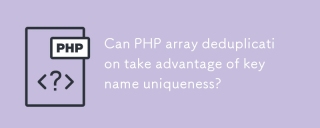
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
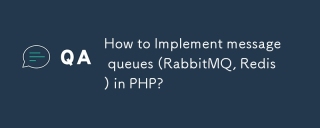
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
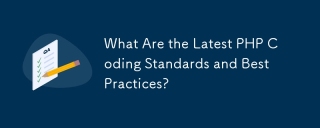
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
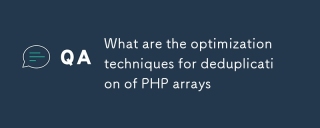
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
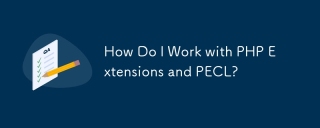
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
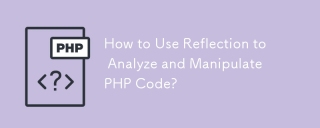
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
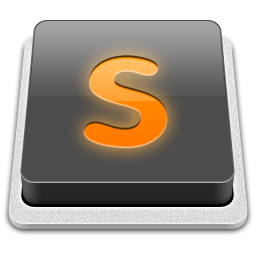
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
