In the process of web development, it is often necessary to convert data in PHP into JSON format for transmission. In PHP, there are two common data types that need to be converted to JSON format, namely arrays and objects. This article will introduce how to convert arrays and objects in PHP to JSON format, and convert JSON format to arrays or objects.
1. Convert PHP array to JSON format
1. Use the json_encode() function
In PHP, you can use the json_encode() function to convert the array into JSON format . This function receives one parameter, which is an array that needs to be converted into JSON format. The following is an example:
$array = array('name' => '张三', 'age' => 20); $json = json_encode($array); echo $json;
After execution, the output result is:
{"name":"张三","age":20}
The above code converts an array containing name and age into JSON format.
By default, the json_encode() function will convert the key names in the array into attribute names in JSON. If you need to preserve key names in JSON format, you can pass a special parameter JSON_FORCE_OBJECT to this function when using it. For example:
$array = array('name' => '张三', 'age' => 20); $json = json_encode($array, JSON_FORCE_OBJECT); echo $json;
After execution, the output result is:
{"name":"张三","age":20}
As you can see, the result here is the same as the previous example.
2. Use foreach to loop through the array
Another way to convert a PHP array into JSON format is to use foreach to loop through the array. For example:
$array = array('name' => '张三', 'age' => 20); $json = '{'; foreach ($array as $key => $value) { $json .= '"' . $key . '":"' . $value . '",'; } $json = substr($json, 0, -1); $json .= '}'; echo $json;
The output result is:
{"name":"张三","age":"20"}
As you can see, this code also converts the array into JSON format. However, this method requires manual splicing of strings, which is more cumbersome.
2. Convert PHP objects to JSON format
1. Use the json_encode() function
Similar to arrays, in PHP, you can also use the json_encode() function to convert The object is converted to JSON format. The following is an example:
class Student { public $name; public $age; } $student = new Student(); $student -> name = "张三"; $student -> age = 20; $json = json_encode($student); echo $json;
After code conversion, the output result is:
{"name":"张三","age":20}
As you can see, this code converts a Student type object into JSON format.
If you need to preserve object type information in JSON format, you can pass it a special parameter JSON_UNESCAPED_UNICODE when using the json_encode() function. For example:
class Student { public $name; public $age; } $student = new Student(); $student -> name = "张三"; $student -> age = 20; $json = json_encode($student, JSON_UNESCAPED_UNICODE); echo $json;
After execution, the output result is:
{"name":"张三","age":20,"__className":"Student"}
2. Use the json_encode() function and array traversal
is similar to the array, and can also be used in PHP Use foreach to loop through object properties. For example:
class Student { public $name; public $age; } $student = new Student(); $student -> name = "张三"; $student -> age = 20; $json = '{'; foreach ($student as $key => $value) { $json .= '"' . $key . '":"' . $value . '",'; } $json = substr($json, 0, -1); $json .= '}'; echo $json;
After execution, the output result is:
{"name":"张三","age":"20"}
As you can see, this code also converts the object into JSON format. However, this method also requires manual splicing of strings, which is more cumbersome.
3. Convert JSON to PHP array or object
When doing web development, it is often necessary to obtain data in JSON format from the front-end page and convert it into an array or object in PHP Carry out subsequent processing. Here's how to convert JSON to an array or object in PHP.
1. Use the json_decode() function to convert JSON into an array
You can use the json_decode() function to convert JSON format data into a PHP array. This function has two parameters, the first is the JSON string that needs to be converted, and the second is a Boolean value used to specify whether to convert the array into a PHP object. The default is false, that is, converted into an array. For example:
$json = '{"name":"张三","age":20}'; $array = json_decode($json, true); var_dump($array);
After execution, the output result is as follows:
array(2) { ["name"]=> string(6) "张三" ["age"]=> int(20) }
As you can see, this function converts the JSON format data into a PHP array and outputs the result.
2. Use the json_decode() function to convert JSON into an object
In addition to converting to an array, you can use the json_decode() function to convert JSON format data into a PHP object. For example:
$json = '{"name":"张三","age":20}'; $obj = json_decode($json); var_dump($obj);
After execution, the output result is as follows:
class stdClass#1 (2) { public $name => string(6) "张三" public $age => int(20) }
As you can see, this function converts the JSON format data into a PHP object and outputs the result.
To sum up, there are many ways to convert arrays and objects in PHP into JSON format, and you can choose to use them according to the actual situation. At the same time, there are also corresponding functions that can be used when obtaining JSON format data from the front-end page and converting it into an array or object in PHP. Mastering the correct use of these functions is of great significance for developing web applications.
The above is the detailed content of How to convert arrays and objects in PHP to JSON format. For more information, please follow other related articles on the PHP Chinese website!
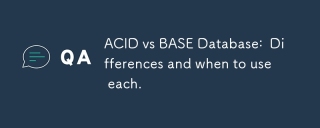
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
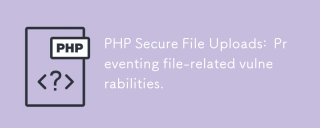
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
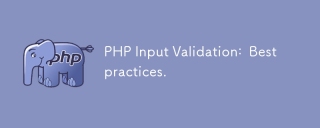
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
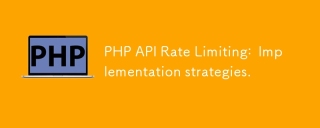
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
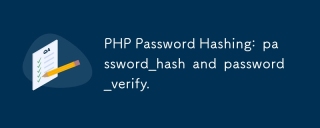
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
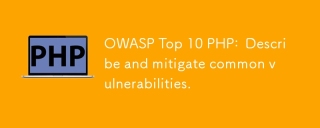
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
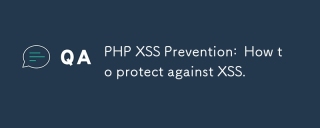
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
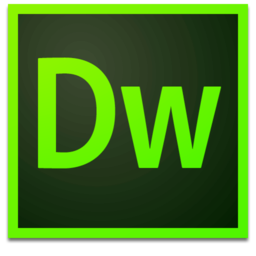
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
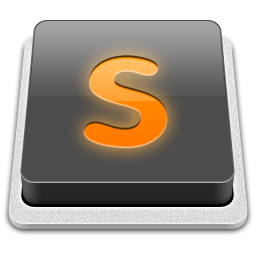
SublimeText3 Mac version
God-level code editing software (SublimeText3)
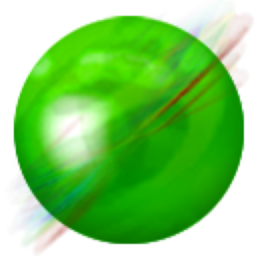
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment