PHP is a powerful programming language with a wide range of applications. When we use PHP to query the database, we usually need to put the query results into an array for subsequent operations. This article will introduce how to use PHP to put query results into an array.
1. Use the mysql_fetch_array() function to obtain query results
In PHP, we use the mysql_query() function to perform query operations. At the same time, we can use the mysql_fetch_array() function to get the data rows from the query results and return them as an array. This function accepts one parameter - the result set returned after executing the query, so we can place it in a while loop to get multiple rows. The following is the sample code:
$query = mysql_query("SELECT * FROM mytable"); $result = array(); while($row = mysql_fetch_array($query)){ $result[] = $row; }
In the above example, we first performed a query operation using the mysql_query() function and saved the result set in the variable $query. Next, we define an array called $result and use a while loop to iterate over the rows in $query. During the loop, we use the mysql_fetch_array() function to get the row data and save it to the $row variable. Next, we add $row to the $result array for subsequent use.
2. Use the mysqli_fetch_array() function to obtain query results
If you are using PHP 5 or higher, you should use the mysqli function instead of the mysql function. The mysqli function provides an improved set of functions and options for MySQL databases. When using mysqli, you can use the mysqli_query() function and mysqli_fetch_array() function to query and obtain results. The following is a sample code for using the mysqli_fetch_array() function to obtain query results:
$query = mysqli_query($connection,"SELECT * FROM mytable"); $result = array(); while($row = mysqli_fetch_array($query)){ $result[] = $row; }
In the above example, we use the mysqli_query() function to perform the query operation and save the result set in the variable $query. Next, we define an array called $result and use a while loop to iterate over the rows in $query. During the loop, we use the mysqli_fetch_array() function to get the row data and save it to the $row variable. Finally, we add $row to the $result array for subsequent use.
Summary
Putting query results into a PHP array is a very simple task. We just need to use the mysql_fetch_array() function or mysqli_fetch_array() function to access the result set and place it in a loop. Whether you're using an older or newer version of PHP, you can use these features to easily get MySQL query results and place them into an array for subsequent processing.
The above is the detailed content of How to put query results into an array using PHP. For more information, please follow other related articles on the PHP Chinese website!
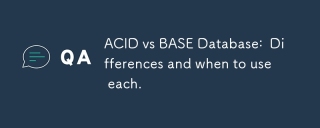
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
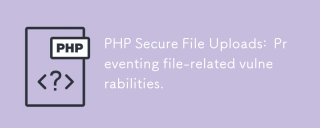
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
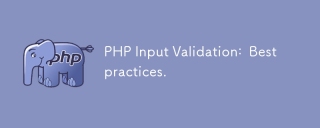
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
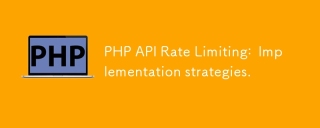
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
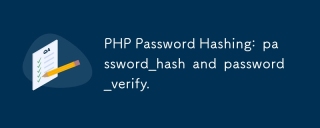
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
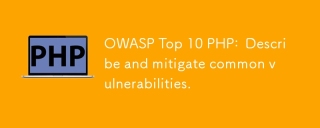
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
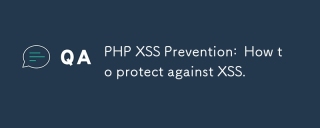
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
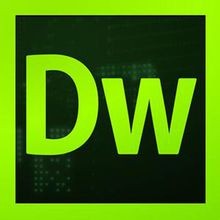
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool