PHP is a programming language widely used for web development. In web applications, arrays are a very important data type as it allows us to group multiple elements together and process them in a more organized way. Receiving array parameters in PHP is also a common need, especially when dealing with web form data. In this article, we'll explain how to handle this situation.
First, we need to know how to send array parameters. When we use a field name in an HTML form and add a number in brackets after it, we can send an array parameter. For example, the following HTML code will send an array named "fruits" to the server:
When we submit this form to a page named submit.php, we will get an array containing all the input field values array. We can receive this array by using PHP's $_POST global variable. For example, the following PHP code will output the form content above:
<?php print_r($_POST['fruits']); ?>
The output will look like this:
Array ( [0] => apple [1] => banana [2] => orange )
We can use this array to perform other operations, such as storing it in a database or performing Other data processing tasks.
In addition to using parentheses, there are other ways to send array parameters. For example, we can send multidimensional arrays using square brackets. Here is an example form through which you can send a multi-dimensional array called "contact":
The above form will send a multi-dimensional array called "contact" to the server which contains name, email and nested arrays of home phone and work phone. We can use the $_POST variable to receive this array in PHP and perform corresponding operations. For example, the following PHP code will output the contents of the form above:
<?php print_r($_POST['contact']); ?>
The output will look like this:
Array ( [name] => John Doe [email] => john.doe@example.com [phone] => Array ( [home] => 1234567890 [work] => 0987654321 ) )
It is a common task to receive array parameters like this and use them for data processing. Whether you wish to store simple values or nested multidimensional arrays in a web form, PHP can easily receive and handle these parameters. Armed with this knowledge, you can start writing web applications that are more powerful, more organized, and easier to maintain.
The above is the detailed content of How to receive array parameters in php. For more information, please follow other related articles on the PHP Chinese website!
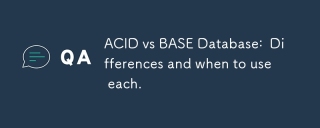
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
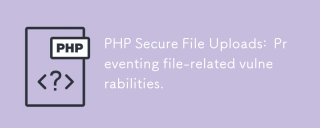
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
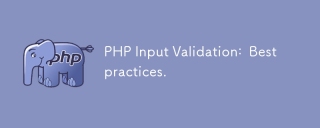
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
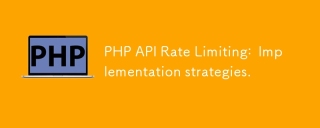
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
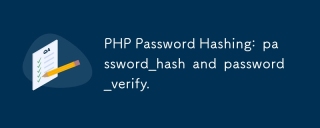
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
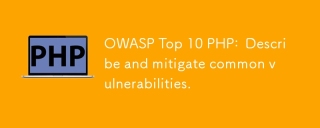
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
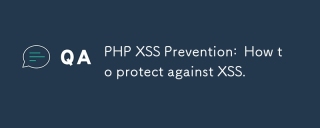
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
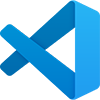
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
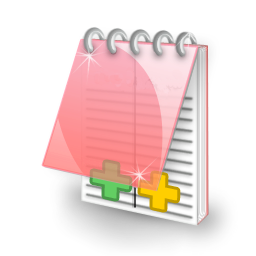
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
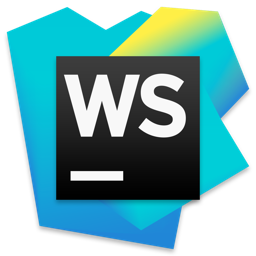
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.