In PHP, there are many methods to determine whether a number is in an array. This article will introduce these methods in detail, and also introduce some other array operation functions.
- Use the in_array() function
The in_array() function can determine whether a value is in an array. The syntax is as follows:
in_array($value, $array, $strict)
Among them, $ The value parameter indicates the value to be found, the $array parameter indicates the array to be searched, and the $strict parameter indicates whether the data type strictly matches, which can be omitted and defaults to false. Returns true if the value is found, false otherwise.
The sample code is as follows:
$fruits = array("apple", "banana", "orange"); if (in_array("apple", $fruits)) { echo "苹果在数组中存在!"; } else { echo "苹果不在数组中存在!"; }
The output result is:
苹果在数组中存在!
- Use the array_search() function
array_search() function Searches for the specified value in the array and returns the corresponding key name, or false if not found. The syntax is as follows:
array_search($value, $array, $strict)
Among them, the $value parameter indicates the value to be found, the $array parameter indicates the array to be searched, and the $strict parameter indicates whether the data type strictly matches, which can be omitted and defaults to false.
The sample code is as follows:
$fruits = array("apple", "banana", "orange"); $key = array_search("banana", $fruits); if ($key !== false) { echo "香蕉在数组中的键名为:" . $key; } else { echo "香蕉不在数组中存在!"; }
The output result is:
香蕉在数组中的键名为:1
If you want to find whether a certain value exists, you can use it like the following:
$fruits = array("apple", "banana", "orange"); if (array_search("orange", $fruits) !== false) { echo "橘子在数组中存在!"; } else { echo "橘子不在数组中存在!"; }
The output result is:
橘子在数组中存在!
- Use in_array() and array_search() to determine simultaneously
In some cases, you need to know whether a value is in the array and Knowing its key name, you can combine the two functions in_array() and array_search() to make a judgment at the same time.
The sample code is as follows:
$fruits = array("apple", "banana", "orange"); if (in_array("banana", $fruits) && ($key = array_search("banana", $fruits)) !== false) { echo "香蕉在数组中的键名为:" . $key; } else { echo "香蕉不在数组中存在!"; }
The output result is:
香蕉在数组中的键名为:1
- Use array_key_exists() function
array_key_exists() function can To determine whether the specified key name exists in the array, the syntax is as follows:
array_key_exists($key, $array)
Among them, the $key parameter represents the key name to be found, and the $array parameter represents the array to be searched. Returns true if the key name is found, false otherwise.
The sample code is as follows:
$fruits = array("apple" => 0, "banana" => 1, "orange" => 2); if (array_key_exists("banana", $fruits)) { echo "香蕉在数组中存在!"; } else { echo "香蕉不在数组中存在!"; }
The output result is:
香蕉在数组中存在!
- Use isset() function
isset() function can To determine whether the specified key name exists in the array, the syntax is as follows:
isset($array[$key])
Among them, the $key parameter represents the key name to be found, and the $array parameter represents the array to be searched. Returns true if the key name is found, false otherwise.
The sample code is as follows:
$fruits = array("apple" => 0, "banana" => 1, "orange" => 2); if (isset($fruits["orange"])) { echo "橘子在数组中存在!"; } else { echo "橘子不在数组中存在!"; }
The output result is:
橘子在数组中存在!
Note: When using the isset() function, please note that if the value corresponding to the key name is null, it will also Returns false, so it cannot accurately determine whether a key exists in the array.
- Use array_diff() function
array_diff() function can find the difference between two arrays, that is, return the difference that is in the first array but not in the following array all values. Therefore, if the value to be found is used as the first array and the original array is used as the second array, and the search result is that the difference set is empty, it means that it exists in the original array.
The sample code is as follows:
$fruits = array("apple", "banana", "orange"); if (count(array_diff(array("banana"), $fruits)) == 0) { echo "香蕉在数组中存在!"; } else { echo "香蕉不在数组中存在!"; }
The output result is:
香蕉在数组中存在!
- Use preg_grep() function
preg_grep() function can Use a regular expression to match array elements and return all matching elements. Therefore, if the value to be found is used as a regular expression and the original array is used as a parameter, and the search result is a non-empty array, it means that it exists in the original array.
The sample code is as follows:
$fruits = array("apple", "banana", "orange"); if (count(preg_grep("/banana/", $fruits)) > 0) { echo "香蕉在数组中存在!"; } else { echo "香蕉不在数组中存在!"; }
The output result is:
香蕉在数组中存在!
The above are various methods for judging whether a certain number is in an array in PHP. We can use it according to the actual situation. You need to choose a method that suits you. In addition, there are many other array operation functions, such as array_merge(), array_slice(), array_reverse(), etc. We can choose different functions to use according to different needs.
The above is the detailed content of How to determine whether a certain number is in an array in php. For more information, please follow other related articles on the PHP Chinese website!
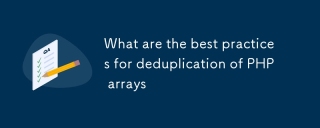
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
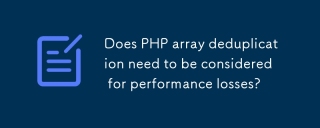
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
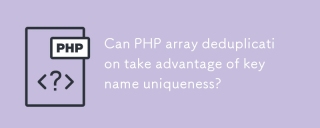
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
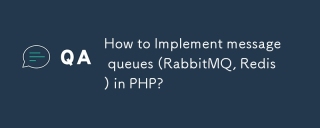
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
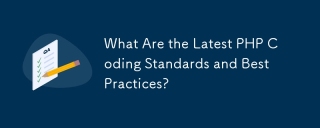
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
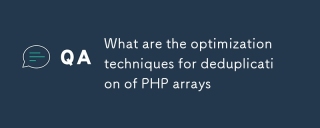
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
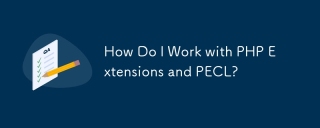
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
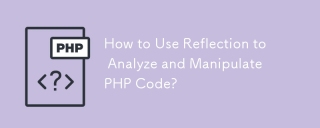
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
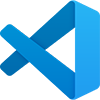
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
