PHP is a popular programming language used for web programming because it excels in aspects such as flexibility and security during the development of web applications. PHP can be used to solve many computational problems, such as matrix transformations. Matrix transformation converts a matrix from one form to another. In this article, we will explore how to transform matrices using PHP.
Matrix transformation is a common mathematical operation, especially in data science. In this process, we transform one matrix into another form. Code can be written using PHP to perform this conversion. In this example, we will transpose and row/column swap a matrix using PHP.
Transpose Matrix
Transposing a matrix is an operation in which the rows and columns of the matrix are swapped. For example, if there is a 3 x 2 matrix A:
1 2
3 4
5 6
its transposed matrix AT will be :
1 3 5
2 4 6
We can use PHP code to achieve this operation.
For example, the following code demonstrates how to transpose a matrix named $matrix. We will first create a $matrix and print the original matrix on the screen. We will then use a for loop to create a new array $transposed to store the transposed matrix. For each column, we will loop through each row and copy the corresponding value from the original matrix into the new matrix. Finally, we will print the transposed matrix.
$matrix = array( array(1, 2), array(3, 4), array(5, 6) ); echo "Original Matrix:<br>"; printMatrix($matrix); $transposed = array(); for ($i=0; $i<count echo></count>"; printMatrix($transposed); function printMatrix($matrix) { echo "
This code will output the following on the screen:
原始矩阵: 1 2 3 4 5 6 转置矩阵: 1 3 5 2 4 6
Row/column swapping
Row/column swapping is another form of matrix transformation. In this case, we swap the rows and columns of the matrix. For example, suppose we have the following matrix A:
1 2 3
4 5 6
7 8 9
If we want to convert the By exchanging column 1 and column 3, we can get a new matrix:
3 2 1
6 5 4
9 8 7
Below is an example of PHP code to do this. We will create an array and print it to the screen. We then swap the first and third columns of the matrix and print the matrix again.
$matrix = array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ); echo "Original Matrix:<br>"; printMatrix($matrix); for ($i=0; $i<count echo></count>"; printMatrix($matrix);
This code will output the following on the screen:
Original Matrix: 1 2 3 4 5 6 7 8 9 Matrix after swapping columns: 3 2 1 6 5 4 9 8 7
Summary
Matrix transformation is a common mathematical operation that can be used in a variety of fields, including Data Science. PHP is a popular programming language used for web programming and can be used to transform matrices. In this article, we have covered how to transpose and row/column swap a matrix using PHP code. Such matrix transformations can be easily done in PHP, and this kind of task is extremely common in data science.
The above is the detailed content of Explore how to use PHP transformation matrices. For more information, please follow other related articles on the PHP Chinese website!
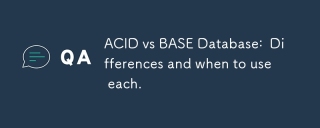
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
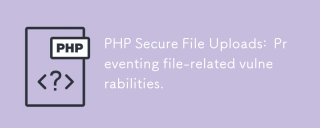
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
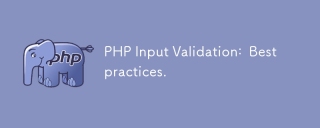
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
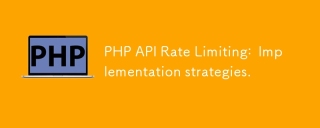
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
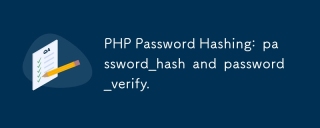
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
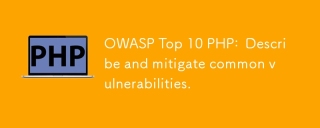
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
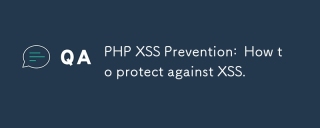
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
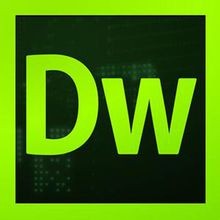
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor