PHP array is a commonly used data type used to store large amounts of data and perform operations. There are some basic rules to follow when working with PHP arrays. One of them is that array values cannot be repeated. This means that you cannot use the same value as a key or element in the same array.
Why array values cannot be repeated?
An array is a special variable type that can store many different types of values. In PHP, arrays can be used to store numbers, strings, objects, etc. In an array, each element has a unique key and value. Keys can be repeated, but values cannot be repeated, otherwise conflicts will occur.
For example, if you have an array that stores a list of students, each student name is the value of an element. If two students have the same name, their position in the array will not be determined, which will lead to confusion and errors. Therefore, in order to avoid this situation, the values of the array must be unique.
How to avoid duplication of array values?
PHP provides some methods to ensure the uniqueness of array values. Here are some of the available methods:
- Using the array_unique() function
This function can be used to remove duplicate values from an array. It returns a new array in which each value appears only once. For example, the following code demonstrates how to use the array_unique() function:
$names = array("John", "Mary", "David", "Mary", "John"); $names = array_unique($names); print_r($names);
Output results:
Array ( [0] => John [1] => Mary [2] => David )
- Using the in_array() function
This function Can be used to check if a value already exists in the array. If the values are duplicated, appropriate action can be taken. For example, the following code demonstrates how to use the in_array() function:
$names = array("John", "Mary", "David"); if(in_array("Mary", $names)) { echo "Mary is already in the array"; } else { array_push($names, "Mary"); } print_r($names);
Output results:
Mary is already in the array Array ( [0] => John [1] => Mary [2] => David )
- Using the array_search() function
This function Can be used to find a value in an array and return the key of that value. If the values are duplicated, appropriate action can be taken. For example, the following code demonstrates how to use the array_search() function:
$names = array("John", "Mary", "David"); $key = array_search("Mary", $names); if($key !== false) { echo "Mary is already in the array at index $key"; } else { array_push($names, "Mary"); } print_r($names);
Output results:
Mary is already in the array at index 1 Array ( [0] => John [1] => Mary [2] => David )
Summary
When using PHP arrays, It is very important to avoid duplicate values. You can use functions such as array_unique(), in_array(), and array_search() to ensure the uniqueness of array values. When writing code, please pay attention to whether the values of the array are unique, this will help avoid unnecessary errors.
The above is the detailed content of Why can't array values be repeated in php? How to avoid it?. For more information, please follow other related articles on the PHP Chinese website!
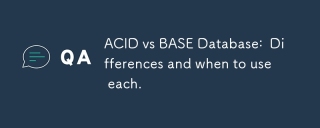
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
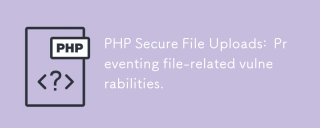
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
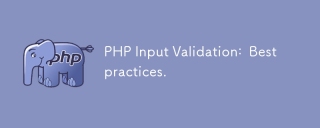
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
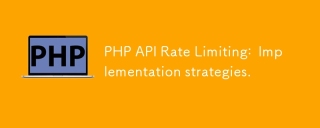
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
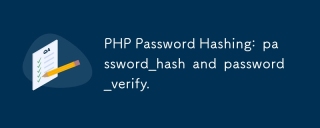
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
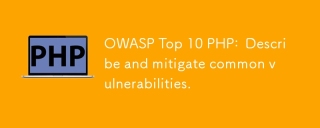
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
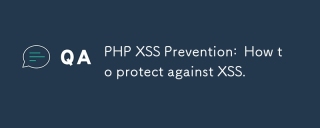
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
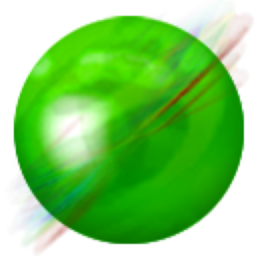
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
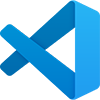
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment