PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
introduction
Have you ever thought about how to easily create interactive web content in PHP? This article will take you into the deep understanding of how to utilize the powerful features of PHP to quickly achieve a dynamic and interactive web experience. Whether you are a beginner or an experienced developer, mastering these techniques will greatly improve your web development efficiency. Ready to explore the interactive world of PHP?
Review of basic knowledge
Before we get started, let's quickly review the basics of PHP. PHP is a server-side scripting language that is widely used in Web development. One of its main advantages is that it can be embedded in HTML, making the generation of dynamic content very simple. In addition, PHP also provides a rich set of built-in functions and libraries, supporting database operations, file processing and other functions.
If you are not familiar with the basic syntax and variable operations of PHP, it is recommended to learn these basic knowledge first. Understanding concepts such as variables, loops, conditional statements, etc. will lay a solid foundation for your subsequent learning.
Core concept or function analysis
Definition and function of PHP dynamic content generation
One of the core advantages of PHP is the ability to dynamically generate web content. This means that you can generate web content in real time based on user input, data in the database, or other conditions. This dynamicity makes the web page no longer static, but can display different information according to different situations.
For example, suppose you want to display different welcome messages based on the user's login status, you can do this:
<?php if (isset($_SESSION['username'])) { echo "Welcome, " . $_SESSION['username']; } else { echo "Welcome, Guest"; } ?>
This simple example shows how PHP generates content dynamically based on session state.
How it works
PHP works by parsing PHP code and converting it to HTML output. When the browser requests a PHP file, the web server passes the request to the PHP interpreter. The PHP interpreter executes PHP code, generates HTML and other outputs, and then sends those outputs back to the browser.
When processing dynamic content, PHP calculates and operates based on the logic in the code. For example, in the example above, PHP checks if the session variable exists and then decides what to output based on the result. This dynamic generation process makes PHP very suitable for creating interactive web pages.
Example of usage
Basic usage
Let's look at a more complex example showing how to create a simple form handler using PHP. This example will show how to receive user input and generate dynamic content based on the input.
<!DOCTYPE html> <html> <body> <form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>"> Name: <input type="text" name="fname"> <input type="submit"> </form> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { // Collect form data $name = htmlspecialchars($_REQUEST['fname']); if (empty($name)) { echo "Name is empty"; } else { echo "Hello " . $name . "!"; } } ?> </body> </html>
This example shows how to process form submissions using PHP and generate dynamic content based on user input. Note that we used the htmlspecialchars
function to prevent XSS attacks, which is an important security practice.
Advanced Usage
Now, let's look at a more advanced example showing how to create a simple user registration system using PHP and MySQL. This example will show how to process user input, verify data, and store data into a database.
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // Create a connection $conn = new mysqli($servername, $username, $password, $dbname); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST['username']; $password = $_POST['password']; $email = $_POST['email']; // Verify input if (empty($username) || empty($password) || empty($email)) { echo "All fields are required"; } else { // Prepare the SQL statement $stmt = $conn->prepare("INSERT INTO users (username, password, email) VALUES (?, ?, ?)"); $stmt->bind_param("sss", $username, password_hash($password, PASSWORD_DEFAULT), $email); // Execute SQL statement if ($stmt->execute()) { echo "New record created successfully"; } else { echo "Error: " . $stmt->error; } $stmt->close(); } } $conn->close(); ?> <!DOCTYPE html> <html> <body> <form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>"> Username: <input type="text" name="username"><br> Password: <input type="password" name="password"><br> Email: <input type="email" name="email"><br> <input type="submit"> </form> </body> </html>
This example shows how to create a simple user registration system using PHP and MySQL. We used password_hash
function to store passwords securely, which is an important security practice. In addition, we use preprocessing statements to prevent SQL injection attacks.
Common Errors and Debugging Tips
There are some common problems you may encounter when creating interactive web content using PHP. Here are some common errors and their debugging tips:
Form data not received : Make sure that the form's
method
property is set correctly and thataction
property points to the correct processing script. Usevar_dump($_POST)
to check the received data.Database connection failed : Check whether the database connection parameters are correct, and use
mysqli_connect_error()
function to obtain detailed error information.SQL query error : Use
mysqli_error()
function to obtain detailed error information to ensure that the SQL statement syntax is correct.Security issues : Always use the
htmlspecialchars
function to prevent XSS attacks, and preprocessing statements to prevent SQL injection attacks.
Performance optimization and best practices
Here are some recommendations for performance optimization and best practices when creating interactive web content using PHP:
Using Cache : For frequently accessed pages, a caching mechanism can be used to reduce the overhead of database queries and PHP processing. PHP provides caching solutions such as
APC
andMemcached
.Optimize database query : minimize the number of database queries and use indexes to improve query efficiency. Avoid using
SELECT *
and select only the fields you want.Code readability : Write clear, readable code, use meaningful variable and function names, and add appropriate comments. This not only helps debugging, but also improves the maintenance of the code.
Error handling : Use
try-catch
block to handle exceptions, provide friendly error information to the user, and record detailed error logs for debugging.Security : Always verify and filter user input to prevent XSS and SQL injection attacks. Use
password_hash
andpassword_verify
functions to handle passwords.
By mastering these tips and best practices, you will be able to create interactive web content more efficiently and safely with PHP. I hope this article can provide you with valuable insights and guidance, and I wish you all the best on the road to PHP development!
The above is the detailed content of PHP: Creating Interactive Web Content with Ease. For more information, please follow other related articles on the PHP Chinese website!
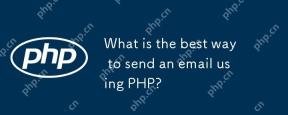
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
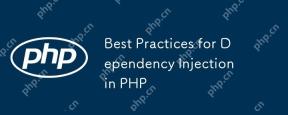
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
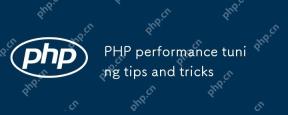
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
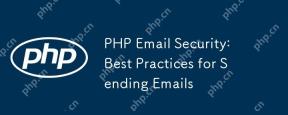
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
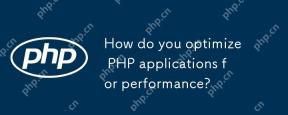
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
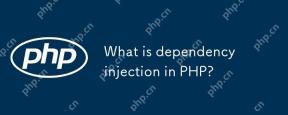
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
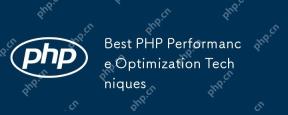
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
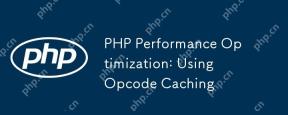
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
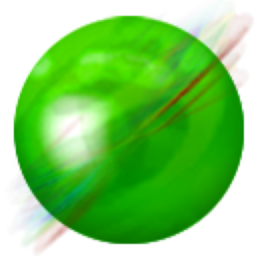
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
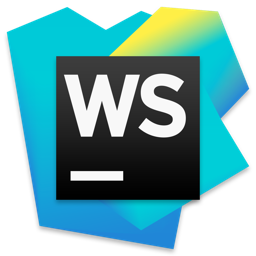
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
