PHP CSRF Protection: How to prevent CSRF attacks
Cross-Site Request Forgery (CSRF) attacks can be particularly dangerous because they trick users into performing unintended actions on a web application that trusts them. To prevent CSRF attacks in PHP, you can follow these strategies:
- Use CSRF Tokens: Generate a unique token for each user session and include this token in every form that triggers a state-changing operation. The token should be verified on the server before processing the request.
-
Same-Site Cookies: Use the
SameSite
attribute for cookies. SettingSameSite
toStrict
orLax
can help prevent CSRF by ensuring cookies are not sent with cross-origin requests. - Double-Submit Cookie: This method involves sending the CSRF token in both a cookie and as a request parameter. The server then verifies that the token values match.
- Check Referer Header: While not foolproof, checking the referer header can provide an additional layer of protection. Ensure the request comes from your own domain.
- Avoid Using GET for State-Changing Operations: Use POST for operations that change server state, as GET requests can be easily triggered from other sites.
-
Implement Proper Session Management: Ensure sessions are properly managed and cookies are set with appropriate security flags like
HttpOnly
andSecure
.
By implementing these measures, you can significantly reduce the risk of CSRF attacks on your PHP application.
What are the best practices for implementing CSRF tokens in PHP?
Implementing CSRF tokens effectively in PHP involves several best practices:
-
Generate Unique Tokens: Use a cryptographically secure method to generate tokens. PHP's
random_bytes
andbin2hex
functions can be used to create a secure token.$token = bin2hex(random_bytes(32));
-
Store Tokens Securely: Store the token in the user's session or as a cookie. If using a session, ensure session fixation attacks are prevented.
session_start(); $_SESSION['csrf_token'] = $token;
-
Include Token in Forms: Embed the token in forms as a hidden input field.
<input type="hidden" name="csrf_token" value="<?php echo htmlspecialchars($token); ?>">
- Validate Tokens on Submission: Verify the token on form submission against the stored value.
- Regenerate Tokens: Consider regenerating tokens after successful form submissions or after a certain period to reduce the attack window.
- Use Token in All State-Changing Requests: Include CSRF tokens in all requests that modify server state, not just traditional form submissions but also AJAX calls.
- Avoid Predictable Tokens: Ensure tokens are not predictable or guessable by an attacker.
Following these practices will help you maintain the integrity of your CSRF protection mechanism.
Can you recommend any PHP libraries for CSRF protection?
Several PHP libraries can simplify the implementation of CSRF protection:
- OWASP CSRFGuard PHP: A library from the Open Web Application Security Project (OWASP) designed specifically for CSRF protection. It offers robust mechanisms for token generation, validation, and integration with various frameworks.
-
Symfony Security: If you are using the Symfony framework, it comes with built-in CSRF protection. The
CsrfExtension
andCsrfTokenManager
classes provide comprehensive support for generating and validating CSRF tokens. -
Laravel: Laravel's CSRF protection is straightforward to implement. The framework automatically generates a CSRF token for each active user session, and it's included in forms via the
@csrf
Blade directive. -
Zend Framework: Zend Framework offers CSRF protection through its
Zend\Validator\Csrf
component, which can be easily integrated into forms. - Aura.Web: A lightweight library offering CSRF token generation and validation, suitable for use with any PHP project.
Using one of these libraries can save development time and ensure robust CSRF protection in your application.
How do I validate CSRF tokens on form submissions in PHP?
Validating CSRF tokens on form submissions in PHP involves comparing the token sent with the form to the one stored in the session or cookie. Here’s a step-by-step guide:
-
Retrieve the Stored Token: Access the token stored in the session or cookie.
session_start(); $storedToken = $_SESSION['csrf_token'];
-
Retrieve the Submitted Token: Get the token sent with the form submission.
$submittedToken = $_POST['csrf_token'];
-
Validate the Token: Compare the stored token with the submitted token.
if (!hash_equals($storedToken, $submittedToken)) { // Token mismatch, handle the error http_response_code(403); die("CSRF token validation failed"); }
-
Proceed with the Request: If the tokens match, proceed with processing the form data.
// Tokens match, proceed with the form submission // Process the form data here
-
Regenerate the Token: Optionally, regenerate the token after a successful submission to enhance security.
$newToken = bin2hex(random_bytes(32)); $_SESSION['csrf_token'] = $newToken;
By following these steps, you can ensure that CSRF tokens are properly validated, thereby protecting your application against CSRF attacks.
The above is the detailed content of PHP CSRF Protection: How to prevent CSRF attacks.. For more information, please follow other related articles on the PHP Chinese website!
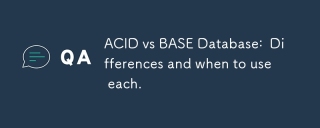
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
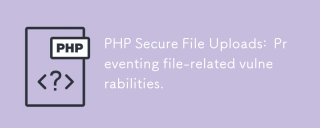
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
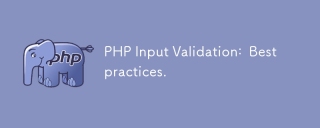
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
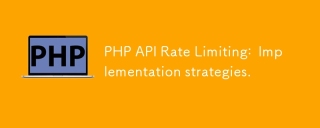
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
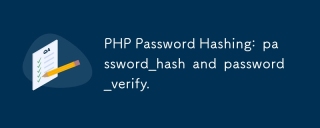
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
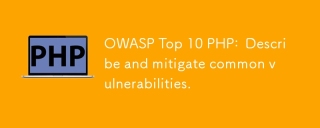
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
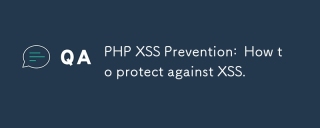
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
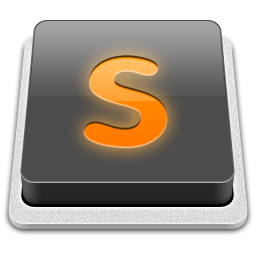
SublimeText3 Mac version
God-level code editing software (SublimeText3)
