How Can I Use the Observer Pattern for Event Handling in PHP?
The Observer pattern, also known as the Publish-Subscribe pattern, is a behavioral design pattern that defines a one-to-many dependency between objects. It allows one object (the Subject) to notify its dependents (the Observers) about state changes without the Subject having to know specifics about the Observers. This decoupling makes the system more flexible and maintainable. In PHP, you can implement this using interfaces or abstract classes.
A basic implementation involves a Subject
class that maintains a list of Observer
objects. The Subject
provides methods to attach and detach observers. When the Subject
's state changes, it iterates through its list of observers and calls a update()
method on each, passing relevant data. The Observer
interface defines the update()
method that each observer must implement.
Here's a simplified example:
interface Observer { public function update(Subject $subject); } interface Subject { public function attach(Observer $observer); public function detach(Observer $observer); public function notify(); } class ConcreteSubject implements Subject { private $observers = []; private $state; public function attach(Observer $observer) { $this->observers[] = $observer; } public function detach(Observer $observer) { $key = array_search($observer, $this->observers, true); if ($key !== false) { unset($this->observers[$key]); } } public function notify() { foreach ($this->observers as $observer) { $observer->update($this); } } public function setState($state) { $this->state = $state; $this->notify(); } public function getState() { return $this->state; } } class ConcreteObserver implements Observer { public function update(Subject $subject) { echo "Observer received notification: " . $subject->getState() . PHP_EOL; } } $subject = new ConcreteSubject(); $observer1 = new ConcreteObserver(); $observer2 = new ConcreteObserver(); $subject->attach($observer1); $subject->attach($observer2); $subject->setState("State changed!"); $subject->detach($observer1); $subject->setState("State changed again!");
This code demonstrates the basic interaction between the Subject and Observers. The setState()
method triggers the notification process.
What are the benefits of using the Observer pattern over other event handling methods in PHP?
The Observer pattern offers several advantages over other event handling mechanisms like simple callbacks or event listeners provided by frameworks:
- Loose Coupling: The Subject doesn't need to know the specifics of its Observers. This reduces dependencies and makes the system more modular and easier to maintain. Adding or removing Observers doesn't require modifying the Subject.
- Flexibility and Extensibility: New Observers can be added easily without affecting existing ones or the Subject. This promotes a highly extensible design.
- Improved Readability and Maintainability: The pattern clearly separates concerns, making the code more organized and easier to understand.
- Broadcast Capabilities: A single event from the Subject can be handled by multiple Observers simultaneously.
Compared to simple callbacks, the Observer pattern provides a more structured and manageable approach, especially in complex systems with numerous event handlers. Frameworks often offer event listener mechanisms that are conceptually similar to the Observer pattern, but the Observer pattern offers a more general-purpose solution that can be implemented independently of any specific framework.
How do I implement a concrete Observer and Subject in PHP using the Observer pattern?
Implementing concrete Observer
and Subject
classes involves extending the interfaces (or abstract classes) defined in the previous section. The Subject
class needs to manage the list of observers, provide methods for attaching and detaching observers, and trigger the notification mechanism. The Observer
class needs to implement the update()
method, which defines how the observer reacts to the notification.
The example in the first section already demonstrates this. ConcreteSubject
is a concrete implementation of the Subject
interface, and ConcreteObserver
is a concrete implementation of the Observer
interface. These classes demonstrate how to manage the observer list, trigger notifications, and handle updates. You would adapt these classes to your specific needs, defining the state changes and the actions that the observers should take upon receiving notifications. For example, you might have an Order
class as a Subject and EmailNotification
, SMSNotification
, and DatabaseLogger
as Observers.
Are there any common pitfalls to avoid when using the Observer pattern for event handling in PHP applications?
While the Observer pattern offers significant benefits, several pitfalls should be avoided:
-
Infinite Loops: If an Observer modifies the Subject's state within its
update()
method, it could trigger another notification, leading to an infinite loop. Careful design is necessary to prevent this. - Observer Overload: Having too many Observers attached to a single Subject can impact performance. Consider using more selective notification mechanisms or grouping Observers to mitigate this.
- Tight Coupling through Data Transfer: While the pattern aims for loose coupling, improper data transfer between Subject and Observer can introduce unintended dependencies. Use standardized data structures or events to maintain loose coupling.
-
Unhandled Exceptions: Exceptions thrown within an Observer's
update()
method could disrupt the entire notification process. Implement proper exception handling within the Observer'supdate()
method. - Memory Leaks: If Observers aren't properly detached when they are no longer needed, this could lead to memory leaks, especially if the Subject holds references to a large number of Observers. Ensure proper detaching of observers when they are no longer required.
By understanding and avoiding these common pitfalls, you can effectively leverage the Observer pattern to create robust and maintainable event handling systems in your PHP applications.
The above is the detailed content of How Can I Use the Observer Pattern for Event Handling in PHP?. For more information, please follow other related articles on the PHP Chinese website!
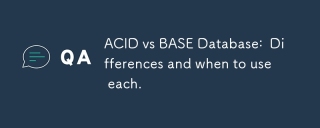
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
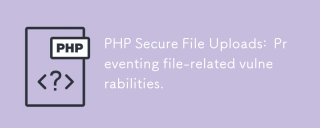
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
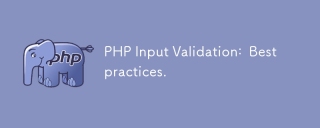
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
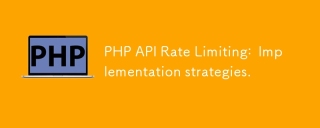
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
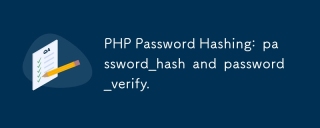
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
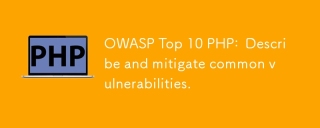
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
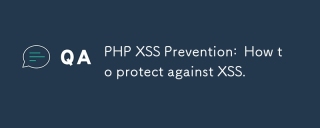
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment