PHP array deduplication: How to verify the result?
Verifying the result of PHP array deduplication involves confirming that all duplicate elements have been removed while preserving the order of the unique elements. There's no single definitive "correct" answer, as the best approach depends on the complexity of your array and your deduplication method. However, several techniques can be employed to achieve a high degree of confidence.
The simplest approach is a visual inspection, especially for small arrays. However, this becomes impractical for larger datasets. More robust methods involve programmatic checks. One such method is to compare the array before and after deduplication. If the post-deduplication array contains fewer elements than the original, and none of the elements in the post-deduplication array appear more than once, then the deduplication was likely successful. We can use PHP's built-in functions to help with this:
<?php $originalArray = [1, 2, 2, 3, 4, 4, 5, 5, 5]; $deduplicatedArray = array_unique($originalArray); // Verify using count() if (count($originalArray) > count($deduplicatedArray)) { echo "Deduplication likely successful (fewer elements).\n"; } else { echo "Deduplication failed (same number of elements).\n"; } //Verify using array_count_values() $counts = array_count_values($deduplicatedArray); $hasDuplicates = false; foreach($counts as $count){ if($count > 1){ $hasDuplicates = true; break; } } if($hasDuplicates){ echo "Deduplication failed (duplicates found).\n"; } else { echo "Deduplication likely successful (no duplicates).\n"; } //Preserve keys while deduplicating $originalArray = ['a' => 1, 'b' => 2, 'c' => 2, 'd' => 3]; $deduplicatedArray = array_unique($originalArray, SORT_REGULAR); //SORT_REGULAR preserves keys ?>
This code snippet first checks if the number of elements has decreased. Then it uses array_count_values()
to count the occurrences of each element in the deduplicated array. If any element appears more than once, it indicates a failure in the deduplication process. Note the use of SORT_REGULAR
with array_unique
to maintain key association if that's important. Remember that array_unique
preserves the first occurrence of each element.
How can I ensure all duplicates are removed from my PHP array after deduplication?
Ensuring complete duplicate removal requires a combination of a robust deduplication algorithm and thorough verification. While array_unique()
is convenient, it might not be sufficient for all scenarios, particularly if you need to handle complex data structures within the array or require specific key preservation.
For more complex scenarios, consider a custom deduplication function. This allows for greater control and the possibility of handling custom comparison logic if your array elements aren't simple scalar values. For example, you might need to compare objects based on specific properties rather than strict equality.
<?php function customDeduplicate(array $array, callable $comparator): array { $unique = []; foreach ($array as $element) { $isDuplicate = false; foreach ($unique as $uniqueElement) { if ($comparator($element, $uniqueElement)) { $isDuplicate = true; break; } } if (!$isDuplicate) { $unique[] = $element; } } return $unique; } //Example usage with objects class User { public $id; public $name; public function __construct($id, $name){ $this->id = $id; $this->name = $name; } } $users = [ new User(1, 'John'), new User(2, 'Jane'), new User(1, 'John'), new User(3, 'Peter') ]; $uniqueUsers = customDeduplicate($users, function($a, $b){ return $a->id === $b->id; //Compare based on ID }); //Verify foreach($uniqueUsers as $user){ echo $user->id . " " . $user->name . "\n"; } ?>
This custom function uses a comparator function to define how duplicates are identified. This allows flexibility in handling different data types and comparison criteria. Always follow the deduplication with the verification steps outlined in the previous section.
What are the efficient ways to check the uniqueness of elements in a PHP array after removing duplicates?
The most efficient way to check uniqueness after removing duplicates is to leverage PHP's built-in functions, specifically array_count_values()
. As shown in the first answer, this function creates an associative array where keys are the values from the input array, and values are their counts. If any count is greater than 1, duplicates remain. This method has a time complexity of O(n), which is quite efficient.
Another approach, though less efficient for large arrays, is to use a combination of array_unique()
and count()
. If the count of the array after array_unique()
is equal to the count of the array before, then no duplicates were removed, indicating a problem with the deduplication process. This is a quicker initial check, but it doesn't definitively prove the absence of duplicates. It only highlights potential problems.
What techniques can I use to confirm the accuracy of my PHP array deduplication function?
Confirming the accuracy of a PHP array deduplication function involves a multi-pronged approach:
- Unit Testing: Write unit tests that cover various scenarios, including empty arrays, arrays with duplicates, arrays with no duplicates, and arrays containing complex data types. Use assertion libraries like PHPUnit to verify the correctness of your function's output.
- Code Review: Have another developer review your code to identify potential flaws or edge cases you might have missed.
- Systematic Testing: Create a suite of test cases with diverse inputs, including edge cases like arrays with mixed data types, null values, and large datasets.
- Comparison with Known Good Results: For a subset of your test data, manually deduplicate the array and compare the result with the output of your function. This serves as a ground truth for verification.
- Profiling and Optimization: If performance is critical, profile your deduplication function to identify bottlenecks and optimize its efficiency.
By combining these techniques, you can significantly increase your confidence in the accuracy and reliability of your PHP array deduplication function. Remember that thorough testing is crucial, especially when dealing with data integrity.
The above is the detailed content of How to verify the results after deduplication of PHP array. For more information, please follow other related articles on the PHP Chinese website!
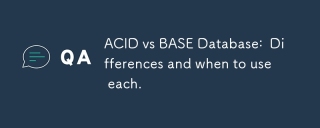
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
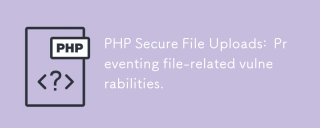
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
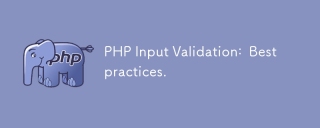
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
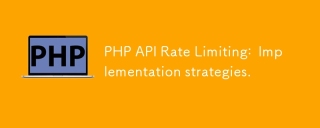
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
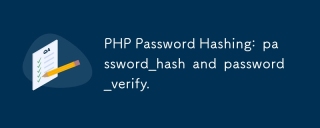
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
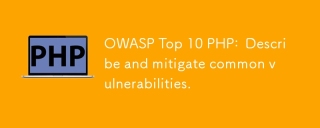
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
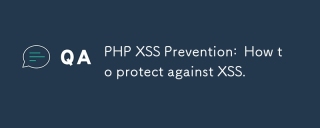
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
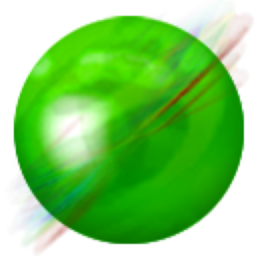
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
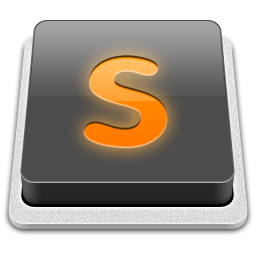
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
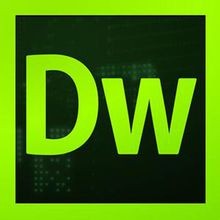
Dreamweaver CS6
Visual web development tools