Effective data validation is crucial for secure and robust web forms. Invalid data can create security vulnerabilities and website malfunctions. This tutorial demonstrates how PHP's filter_var
function efficiently sanitizes and validates user inputs, preventing these issues.
Why Data Sanitization is Often Overlooked
Many developers find data validation tedious, often involving:
- Exhaustive comparisons against every conceivable input variation.
- Crafting complex regular expressions to handle all possibilities.
- Or a combination of both, leading to time-consuming work and a high error rate.
Fortunately, PHP offers a streamlined solution.
Leveraging PHP's filter_var
Function
PHP's filter_var
function simplifies the process. Its syntax is:
filter_var( mixed $value, int $filter = FILTER_DEFAULT, array|int $options = 0 ): mixed
-
$value
: The data to be filtered. -
$filter
: The filter ID (e.g.,FILTER_SANITIZE_EMAIL
,FILTER_VALIDATE_INT
). -
$options
: Optional parameters for filter customization. ReturnsFALSE
on filter failure.
Sanitizing Data with filter_var
Email Sanitization:
The FILTER_SANITIZE_EMAIL
filter removes illegal characters from email addresses. For example:
$email = "test\"';DROP TABLE users;--@example.com"; $sanitizedEmail = filter_var($email, FILTER_SANITIZE_EMAIL); echo $sanitizedEmail; // Outputs: test@example.com (malicious script removed)
URL Sanitization:
Similarly, FILTER_SANITIZE_URL
cleans URLs of harmful characters:
$url = "http://example.com/?param=<🎜>"; $sanitizedUrl = filter_var($url, FILTER_SANITIZE_URL); echo $sanitizedUrl; // Outputs: http://example.com/?param= (script removed)
Validating Data with filter_var
IP Address Validation:
$ip = "127.0.0.1"; if (filter_var($ip, FILTER_VALIDATE_IP)) { // Valid IP address } else { // Invalid IP address }
Integer Validation:
$foo = "123"; if (filter_var($foo, FILTER_VALIDATE_INT)) { // Valid integer } else { // Invalid integer }
Practical Application: An Email Submission Form
Let's build a simple email submission form to illustrate data sanitization and validation. The form collects: name, email, homepage, and message. Only valid data triggers email submission.
Step 1: Creating the Form (form.html):
<form method="post" action="form-email.php"> Name: <input type="text" name="name"><br><br> Email Address: <input type="email" name="email"><br><br> Home Page: <input type="url" name="homepage"><br><br> Message: <textarea name="message"></textarea><br><br> <input type="submit" name="Submit" value="Send"> </form>
Step 2: Handling Form Submission (form-email.php):
<?php $errors = ""; if (isset($_POST['Submit'])) { // ... (Validation and sanitization logic as shown in original example) ... if (empty($errors)) { // Send email using mail() function with sanitized data echo "Thank you for your message!"; } else { echo "Errors: <br>" . $errors; } } ?>
(Note: The complete validation and sanitization logic from the original example should be inserted into the if (isset($_POST['Submit']))
block in form-email.php
.)
Conclusion
This tutorial provides a foundation for using PHP's data filtering capabilities. While not exhaustive, it showcases the efficiency of filter_var
for secure and reliable data handling. Refer to the PHP manual's Data Filtering section for more advanced techniques. The image was generated using OpenAI's DALL-E 2.
The above is the detailed content of Sanitize and Validate Data With PHP Filters. For more information, please follow other related articles on the PHP Chinese website!
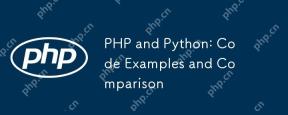
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
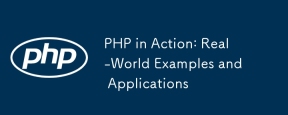
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
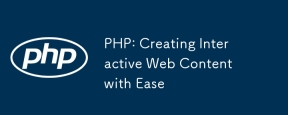
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
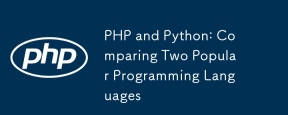
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
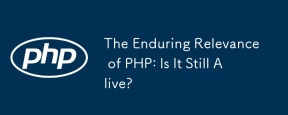
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
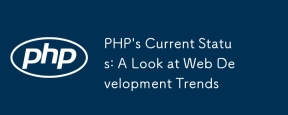
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
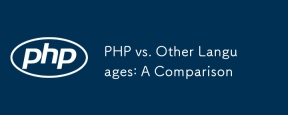
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
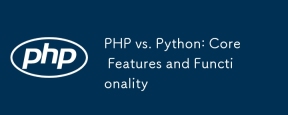
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
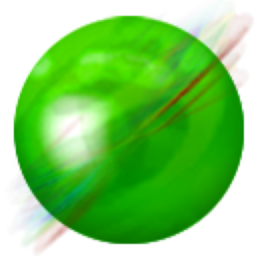
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
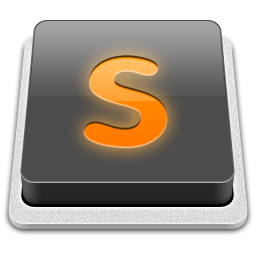
SublimeText3 Mac version
God-level code editing software (SublimeText3)