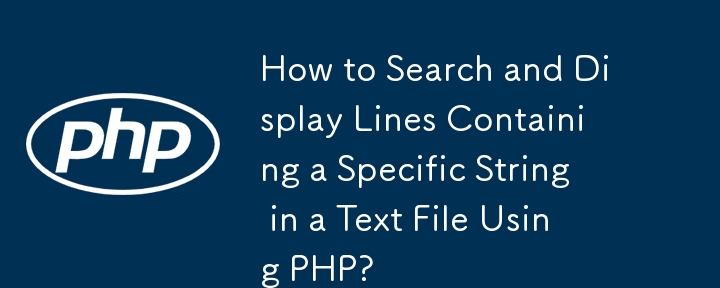
PHP Script to Search and Display Entire Line Containing Specified String from Text File
To create a PHP script that searches for and displays the entire line containing a specified string from a text file, follow these steps:
-
Open the Text File: Use file_get_contents() function to read the contents of the text file into a variable.
-
Escape Special Characters: Utilize preg_quote() function to escape any special characters in the search string to prevent unexpected results.
-
Create Regular Expression: Formulate a regular expression that matches the entire line containing the search string. Use the following pattern: "/^.*$pattern.*$/m". It ensures that the search string can appear anywhere on the line.
-
Perform Search: Utilize preg_match_all() function to search the text file contents for all occurrences of the regular expression. The matching lines will be stored in an array.
-
Display Results: Iterate over the matches and display each entire line.
-
Handle No Matches: If the search string is not found, echo an appropriate message indicating the lack of matches.
Here's a PHP script that implements the above steps:
<?php
$file = 'numorder.txt';
$searchfor = 'aullah1';
header('Content-Type: text/plain');
$contents = file_get_contents($file);
$pattern = preg_quote($searchfor, '/');
$pattern = "/^.*$pattern.*$/m";
if (preg_match_all($pattern, $contents, $matches)) {
echo "Found matches:\n";
echo implode("\n", $matches[0]);
} else {
echo "No matches found";
}
?>
The above is the detailed content of How to Search and Display Lines Containing a Specific String in a Text File Using PHP?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn