The split string is one of the basic operations for any programming language. There are various built-in functions in PHP to deal with split relation operations. When we come to know the word split, we will experience the breaking of a single string into the multiple strings. There are various ways we can split a string as per our business requirements. In other words, if you want to split a string to get the string character by character (1 character each), by space, by special character etc. We can also go with the size combination while dealing with the string split.
ADVERTISEMENT Popular Course in this category PHP DEVELOPER - Specialization | 8 Course Series | 3 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax:
explode(Separator, String, Limit)
- Separator: Separator is a delimiter, that is the required parameter.
- String: The string that needs to be broken into multiple. Again, this is a required parameter.
The limit is an optional parameter.
str_split
str_split(String, Length)
- String: This is a required parameter.
- Length: This is an optional parameter.
preg_split(RegularExpressionPattern, String, Limit, Flags)
RegularExpressionPattern- required parameter.
- String: Required parameter.
- Limit: Optional one.
Working of PHP Split String
Before moving ahead with the string split, we must have a string first to perform the operation on that string. After string, we can come to the required delimiter upon which the string split operation needs to perform.
1. User of explode() Function
This function can be used to break a string into an array of string.
Let’s understand the same with a basic example:
$string = 'Welcome to the India.'; // a string $arrayString= explode(" ", $string ); // split string with space (white space) as a delimiter. Print_r($arrayString); // printing the output array…
2. The User of preg_split()
This function can be used to break a string into multiple ones based on regular expression. This can be useful when we have a string, let’s say date string separated by -. We can break that string with – key.
$string = "2020-06-21 10:10:20"; // a string $outputArr= preg_split("/[-\s:]/", $string);
The above of the code will break the string either by – or by the white space.
3. The user of str_split
This can also do the same job, converting a string to an array of smaller strings.
$string = "Welcome to the India"; // a string $outputArr = str_split("Welcome to the India");
Examples to Implement PHP Split String
Below are the Example of PHP Split String:
Example #1
Split a string where we find the white space.
Code:
<?php $string = 'Welcome to the India.'; // a string echo "Actual String:\n"; echo $string; echo "\n\n"; echo "Array of string after string split:\n"; $arrayString= explode(" ", $string ); // split string with space (white space) as a delimiter. Print_r($arrayString); // printing the output array… ?>
Output:
Example #2
In this example, we will see how a string can be split into multiple strings using a regular expression.
Code:
<?php $string = "2020-06-21 10:10:20"; // a string echo "Actual String:\n"; echo $string; echo "\n\n"; echo "Array of string after string preg_split:\n"; $components = preg_split("/[-\s:]/", $string); print_r($components); ?>
Output:
Example #3
Let’s see another very simple example of string split using the str_split() function.
Code:
<?php $string = "Welcome to the India"; // a string echo "Actual String:\n"; echo $string; echo "\n\n>"; echo "Array of string after string str_split:\n"; $arrays = str_split($string); print_r($arrays); ?>
Output:
Example #4
String split using str_split() function with a specified length.
Code:
<?php $string = "Welcome to the India"; // a string echo "Actual String:\n"; echo $string; echo "\n\n"; echo "Array of string after string str_split:\n"; $arrays = str_split($string,4); print_r($arrays); ?>
Output:
Explanation: Looking at the output of the above program, we can say that some string of length 2 or 3 but we have mentioned the length as a 4. This is just because the string element with 2 carries 2 spaces and the string with 3 characters carry one space with it.
Example #5
Now, using the string split method lets try to get the first string from of that string.
Code:
<?php $string = "What do you want to learn?"; // a string echo "Actual String:\n"; echo $string; echo "\n\n"; echo "Array of string after string str_split:\n"; $arrays = explode(" ",$string); print_r($arrays); echo "\n\nNow getting the first element from this array:\n"; echo $arrays[0]; ?>
Output:
Explanation: In the same way, we can access the other elements of the string. For example, $arrays[1] to access the 2nd element.
Conclusion
We can simply say, in PHP there are various ways to handle the string split. Built-in PHP functions can be used as per the business requirements to process the split string. But code or developer should be aware of using these and the pros and cons of it. Most of the functions of the string split changed the string into an array then we can get the specified string from that array.
Recommended Article
This is a guide to the PHP Split String. Here we discuss the Introduction, syntax, and working of Split String in PHP along with different examples and code implementation. You can also go through our other suggested articles to learn more –
- Overview of Abstract Class in Python
- What is Abstract Class in PHP?
- Socket Programming in PHP with Methods
- PHP chop() | How to Work?
The above is the detailed content of PHP Split String. For more information, please follow other related articles on the PHP Chinese website!
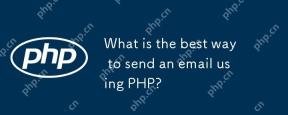
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
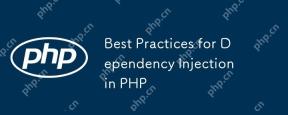
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
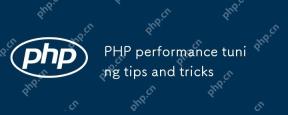
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
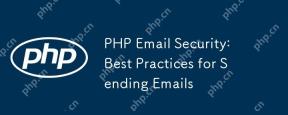
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa
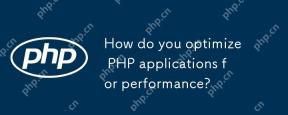
TooptimizePHPapplicationsforperformance,usecaching,databaseoptimization,opcodecaching,andserverconfiguration.1)ImplementcachingwithAPCutoreducedatafetchtimes.2)Optimizedatabasesbyindexing,balancingreadandwriteoperations.3)EnableOPcachetoavoidrecompil
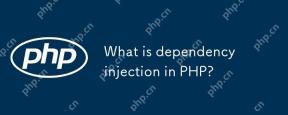
DependencyinjectioninPHPisadesignpatternthatenhancesflexibility,testability,andmaintainabilitybyprovidingexternaldependenciestoclasses.Itallowsforloosecoupling,easiertestingthroughmocking,andmodulardesign,butrequirescarefulstructuringtoavoidover-inje
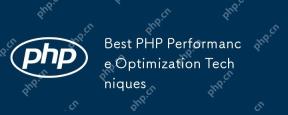
PHP performance optimization can be achieved through the following steps: 1) use require_once or include_once on the top of the script to reduce the number of file loads; 2) use preprocessing statements and batch processing to reduce the number of database queries; 3) configure OPcache for opcode cache; 4) enable and configure PHP-FPM optimization process management; 5) use CDN to distribute static resources; 6) use Xdebug or Blackfire for code performance analysis; 7) select efficient data structures such as arrays; 8) write modular code for optimization execution.
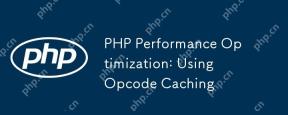
OpcodecachingsignificantlyimprovesPHPperformancebycachingcompiledcode,reducingserverloadandresponsetimes.1)ItstorescompiledPHPcodeinmemory,bypassingparsingandcompiling.2)UseOPcachebysettingparametersinphp.ini,likememoryconsumptionandscriptlimits.3)Ad


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
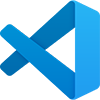
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
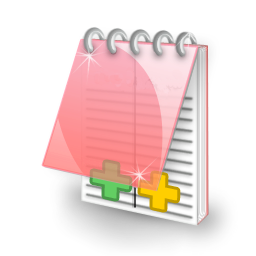
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
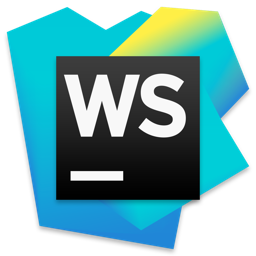
WebStorm Mac version
Useful JavaScript development tools
