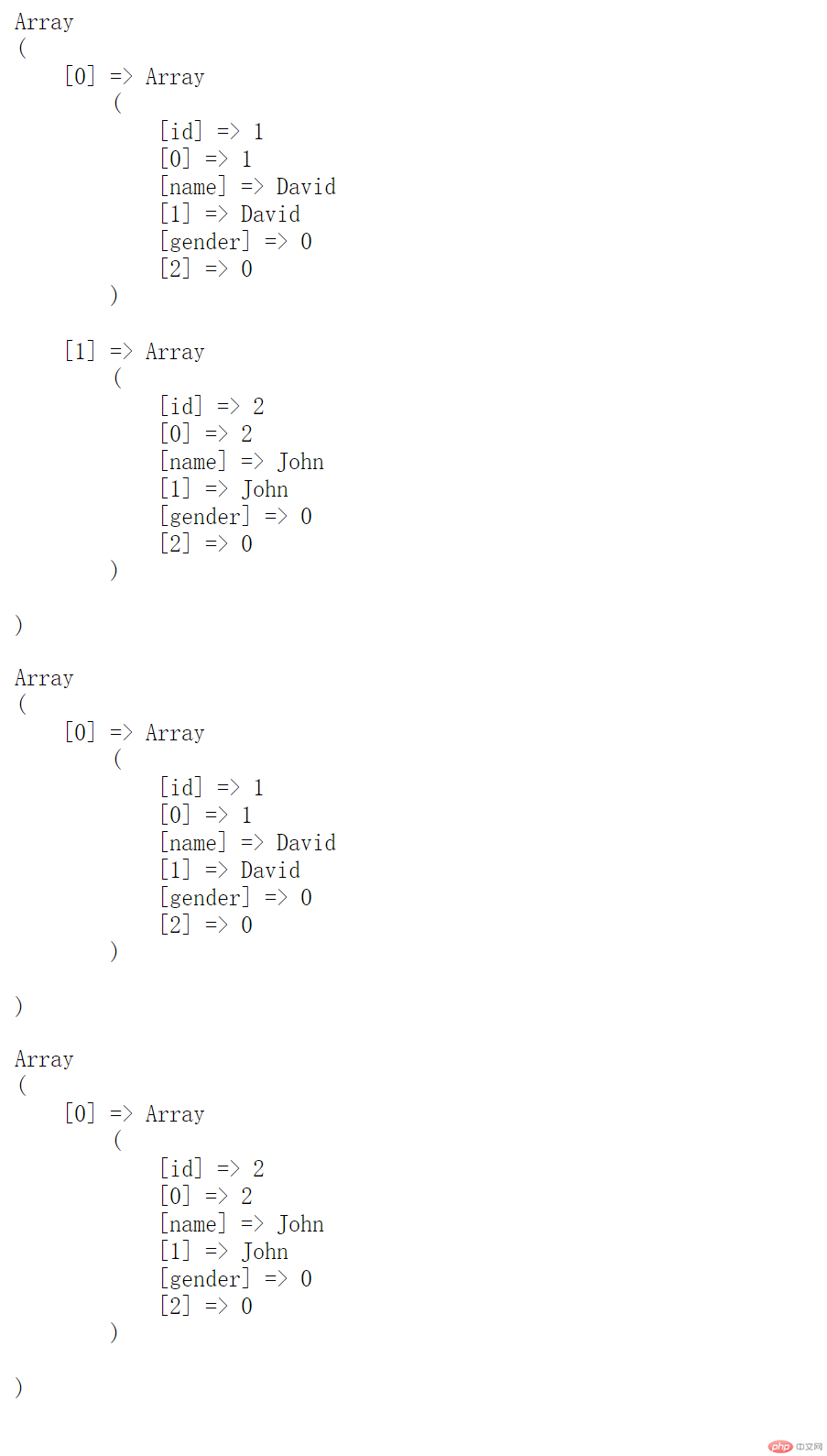
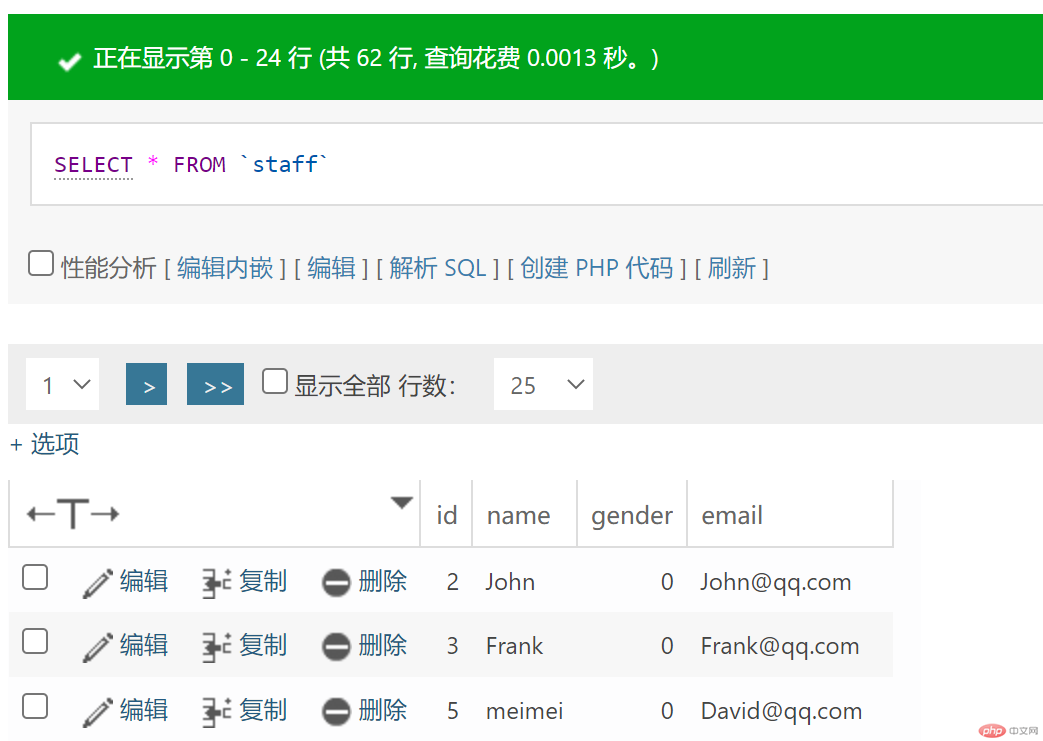
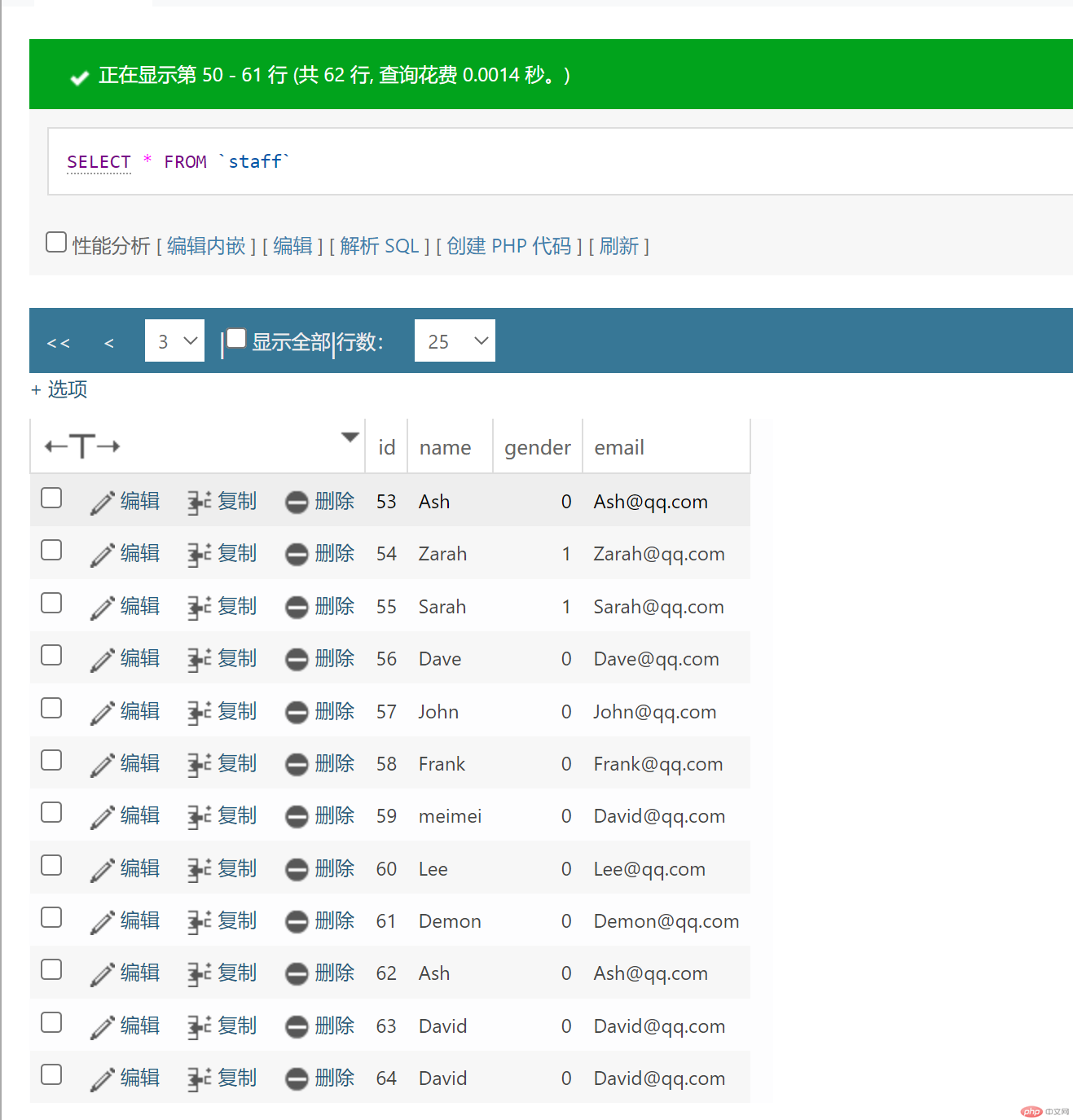
<?php
namespace phpedu;
use PDO;
class DB{
protected $db;
protected $table;
protected $field;
protected $limit;
protected $opt = [];
public function __construct($dsn,$username,$password){
$this->db = new PDO($dsn,$username,$password);
}
// 获取数据表
public function table($table){
$this->table = $table;
return $this;
}
// 获取查询字段
public function field($field){
$this->field = $field;
return $this;
}
// 获取位置限制条件
public function where($where=''){
$this->opt["where"] = " where $where";
return $this;
}
// 获取查询限制
public function limit($limit=10){
$this->limit = $limit;
$this->opt["limit"] = " LIMIT ".$this->limit;
return $this;
}
// 获取偏移量
public function page($page=1){
$this->opt["offset"] = " OFFSET ".($page-1)*$this->limit;
return $this;
}
// 清理查询条件助手
private function cleaner(){
foreach($this->opt as $key=>$item){
$this->opt[$key]=null;
}
}
private function execute($sql){
$stmt = $this->db->prepare($sql);
$stmt->execute();
$this->cleaner();
return $stmt;
}
// 查
public function select(){
$sql = 'SELECT ' . $this->field . ' FROM ' . $this->table;
$sql .= $this->opt["where"] ?? null;
$sql .= $this->opt["limit"] ?? null;
$sql .= $this->opt["offset"] ?? null;
return $this->execute($sql)->fetchAll();
}
// 增
public function insert($data){
$str = '';
foreach ($data as $key=>$value){
$str .= $key.'="'. $value .'", ';
}
$sql = 'INSERT '.$this->table . ' SET ' . rtrim($str, ', ');
return $this->execute($sql)->rowCount();
}
// 改
public function update($data){
$str = '';
foreach ($data as $key=>$value){
$str .= $key.'="'. $value .'", ';
}
$sql = 'UPDATE '.$this->table . ' SET ' . rtrim($str, ', ');
$sql .= $this->opt['where'] ?? die('禁止无条件更新');
return $this->execute($sql)->rowCount();
}
// 删
public function delete(){
$sql = 'DELETE FROM ' . $this->table;
$sql .= $this->opt['where'] ?? die('禁止无条件删除');
return $this->execute($sql)->rowCount();
}
}
$db = new Db('mysql:dbname=phpedu','root','root');
$db_select = $db->table('staff')->field('id,name,gender')->limit(2)->select();
printf("<pre>%s</pre>",print_r($db_select,true));
$db->insert(['name'=>'David', 'gender'=>0, 'email'=>'David@qq.com']);
$db->where('id = 1')->update(['name'=>'David', 'gender'=>0]);
$db_select = $db->table('staff')->field('id,name,gender')->limit(1)->select();
printf("<pre>%s</pre>",print_r($db_select,true));
$db->where('id = 1')->delete();
$db_select = $db->table('staff')->field('id,name,gender')->limit(1)->select();
printf("<pre>%s</pre>",print_r($db_select,true));