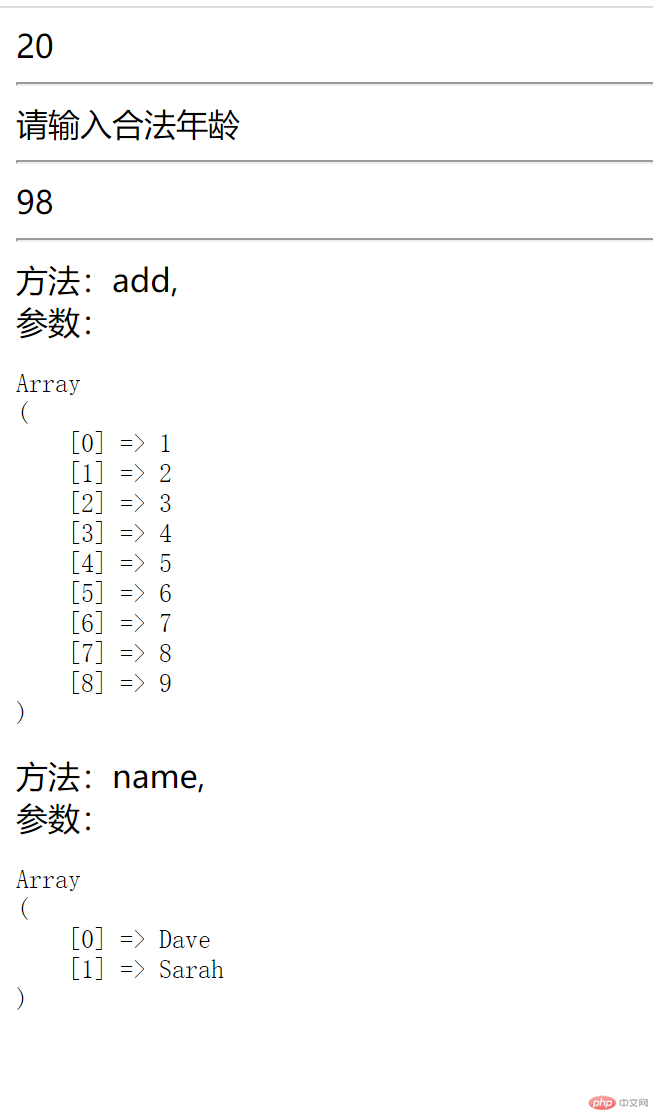
<?php
// 1. 实例演示属性与方法重载
// 1. 属性重载:__get(), __set()
class hw{
private $data=[
'age'=>20
];
// 查询拦截器
public function __get($name){
return $this->data[$name];
}
// 更新拦截器
public function __set($name,$value){
if($value<99){
return $this->data[$name] = $value;
}else{
echo "请输入合法年龄" . "<hr>";
}
}
// 2. 方法重载:__call(),__callStatic()
public function __call($name, $args){
printf("方法:%s,<br/>参数:<pre>%s</pre>",$name,print_r($args,true));
}
public static function __callStatic($name, $args){
printf("方法:%s,<br/>参数:<pre>%s</pre>",$name,print_r($args,true));
}
}
$HW = new hw;
echo $HW->age . "<hr>";
$HW->age = 101;
$HW->age = 98;
echo $HW->age . "<hr>";
$HW->add(1,2,3,4,5,6,7,8,9);
hw::name("Dave","Sarah");
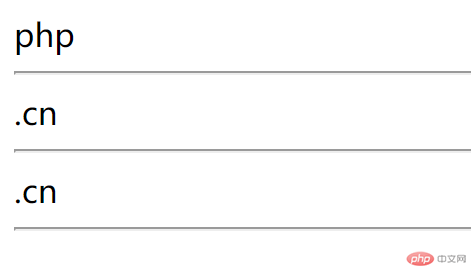
<?php
// 2. 实例演示命名空间与类自动加载器
namespace ns1{
const ns = ".cn";
}
namespace {
const ns = "php";
echo ns . "<hr>";
echo ns1\ns . "<hr>";
echo \ns1\ns . "<hr>";
}
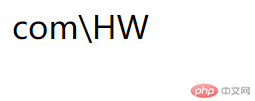
<?php
// 类自动加载器
spl_autoload_register(function($class){
$path = str_replace('\\', DIRECTORY_SEPARATOR, $class);
$file = __DIR__ . DIRECTORY_SEPARATOR . $path . '.php';
require $file;
});
new \com\HW;
<?php
namespace com;
class HW{
}
echo HW::class . "<br/>";